Curious About Technology
Welcome to Coding Explorations, your go-to blog for all things software engineering, DevOps, CI/CD, and technology! Whether you're an experienced developer, a curious beginner, or simply someone with a passion for the ever-evolving world of technology, this blog is your gateway to valuable insights, practical tips, and thought-provoking discussions.
Recent Posts

How to Use Buffer in Go: A Comprehensive Guide
A buffer is a temporary storage area typically used to hold data while it is being moved from one place to another. In the context of Go, a buffer is often used to handle data read from or written to I/O operations, such as reading from a file or writing to a network connection.

Streamlining Your Go Projects with Taskfile: An alternative to Makefile
Managing the myriad tasks involved in software development can be a daunting challenge, particularly when working with Go. From running tests to building executables, repetitive tasks can consume valuable time and introduce errors. Enter Taskfile, a powerful task runner and build tool designed to simplify and automate your workflows.
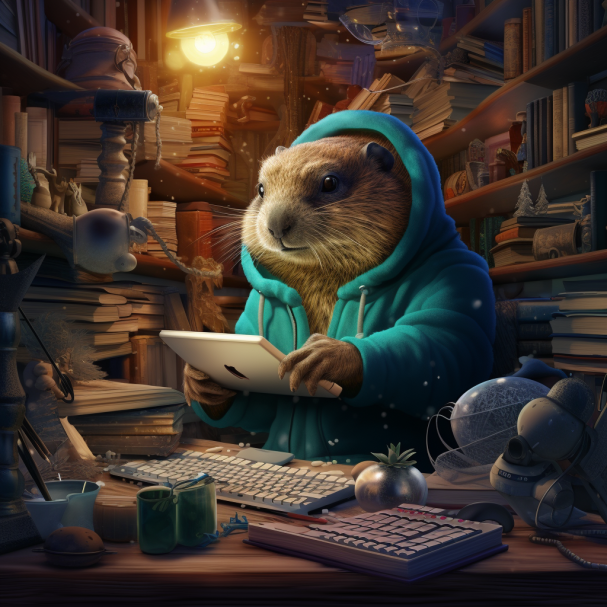
Mastering the Singleton Pattern with Goroutines in Go
In software development, design patterns provide proven solutions to common problems. One such pattern is the Singleton, which ensures a class has only one instance and provides a global point of access to it. When it comes to Go, implementing the Singleton pattern can be a bit tricky, especially when dealing with goroutines and concurrent programming.
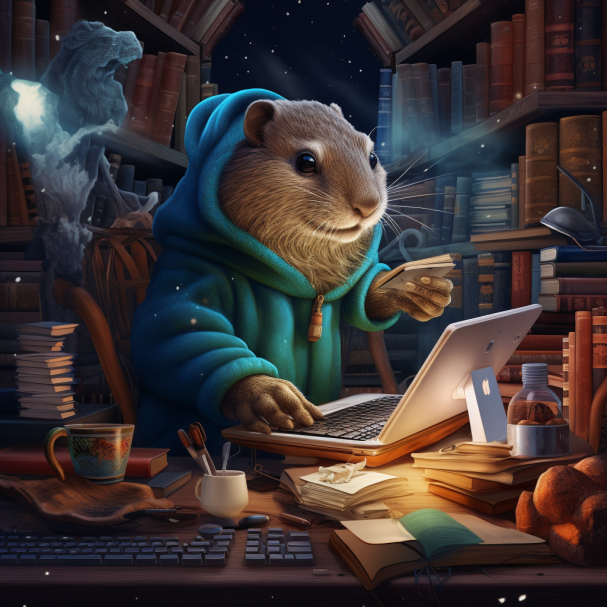
Understanding Null Pointers and Interfaces in Go
In the Go programming language, the concept of null pointers and their interaction with interfaces is an important topic for developers to grasp.
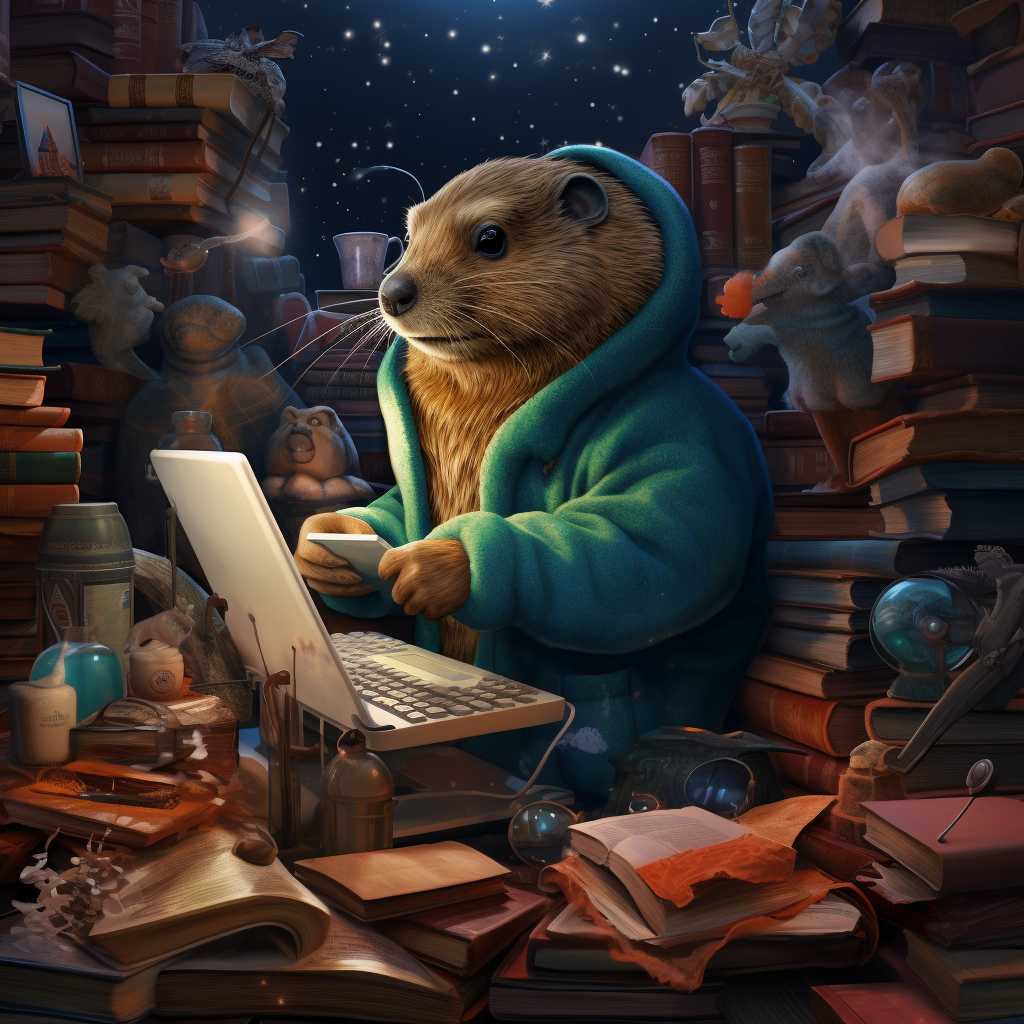
Exploring Function Options in Go
Go, with its simplicity and efficiency, has gained widespread popularity among developers. However, one area where Go initially seemed rigid was in configuring functions with numerous optional parameters. Traditional parameter passing could become cumbersome and error-prone when dealing with functions that required several optional settings. Thankfully, the functional options pattern in Go offers an elegant solution to this problem.

Function Parameters Simplified: Option Structs vs. Variadic Parameters
In programming, functions are the building blocks that allow us to encapsulate behavior and reuse code. However, designing function parameters can be challenging, especially when a function needs to handle a variety of input configurations. Two common techniques to address this challenge are option structs and variadic parameters. Each approach has its own benefits and trade-offs.
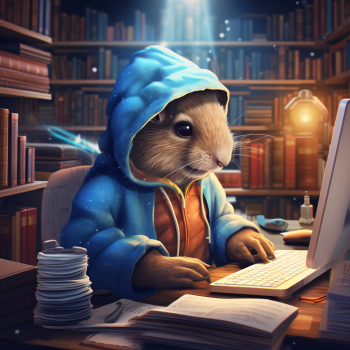
Using Jaeger with OpenTelemetry in Go: A Step-by-Step Guide
In the world of microservices, monitoring and tracing are essential to understand the interactions between services and diagnose issues effectively. OpenTelemetry and Jaeger are popular tools that help in achieving this.
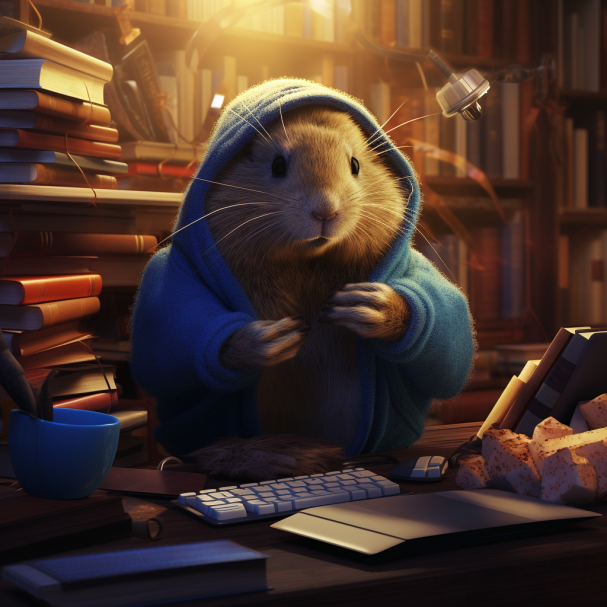
Understanding the Difference Between Update and Save in GORM
When working with databases in Go applications, the GORM library is a popular choice due to its powerful and developer-friendly ORM capabilities. Two of the most frequently used methods for modifying database entries in GORM are Update and Save. While they may seem similar at a glance, they serve distinct purposes and come with different behaviors and implications.

Harnessing the Power of In-Memory Caching in Go
In the realm of software development, performance optimization is a constant priority, especially for applications that demand high throughput and low latency. One effective way to achieve this is through the use of in-memory caching.
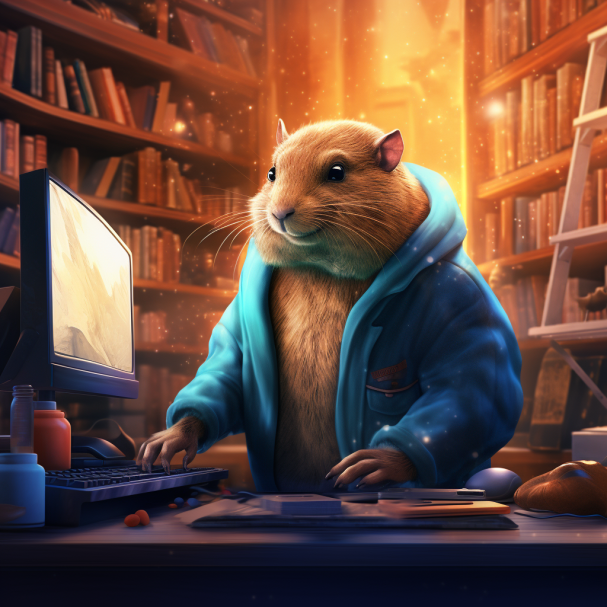
Harnessing Goroutines for Efficient AWS SQS Message Processing in Go
AWS Simple Queue Service (SQS) is a highly scalable and robust queuing service that helps developers decouple and scale microservices, distributed systems, and serverless applications. Integrating SQS with Go, particularly using goroutines, can significantly enhance the efficiency of message processing.
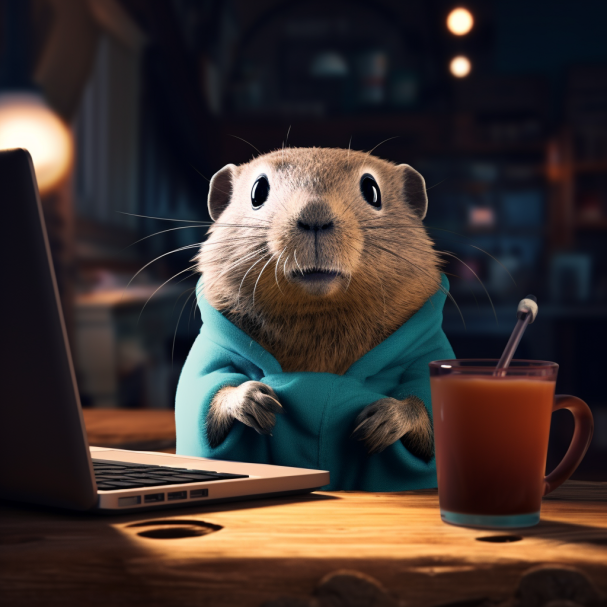
Unlocking Sophisticated Capabilities with Go Struct Tags
In the realm of Go programming, struct tags are powerful yet often underutilized tools that can dramatically enhance the functionality of your applications. These small pieces of metadata attached to struct fields allow for rich configuration and integration with other systems, making them essential for tasks such as data serialization, validation, and database interaction.

Implementing State Machine Patterns in Go
State machines are a fundamental design pattern in software engineering, used to manage complex states within applications. In Go, implementing a state machine can be both efficient and straightforward due to the language’s simplicity and robust features. In this blog post, we’ll explore how to design and implement a state machine in Go, leveraging its strong typing, interface, and concurrency features to create a clean and scalable solution.
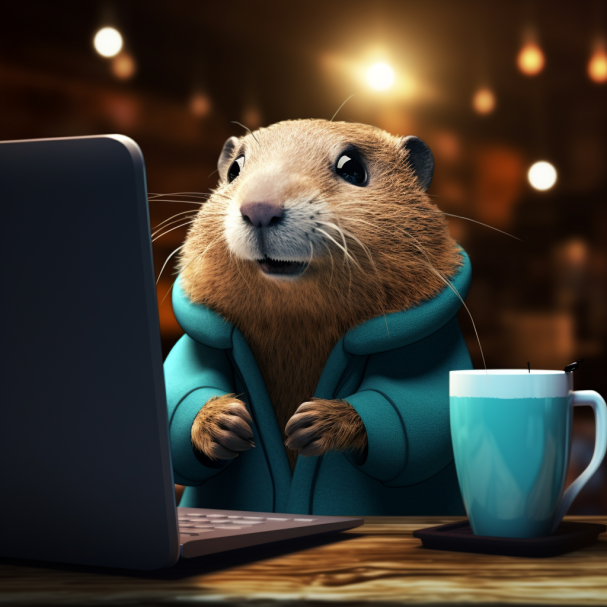
Leveraging Interface Checks in Go: Ensuring Type Safety
In this blog post, we delve into the importance of interface checks in Go programming. Using the example var _ App = (*Application)(nil), we demonstrate how to enforce type conformity to interfaces at compile time, thereby preventing potential runtime errors. Through a practical example, we illustrate how this technique serves not only as a safeguard but also as clear documentation for developers. Learn how to enhance your Go code's reliability and maintainability with interface checks.
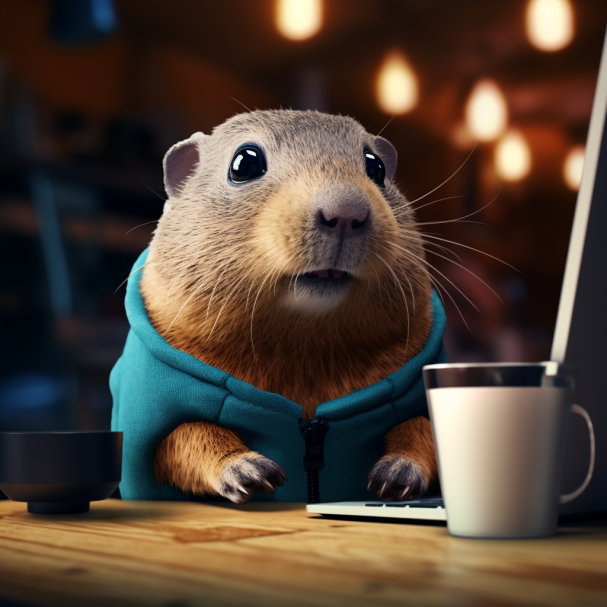
How to Write and Execute Parallel Tests in Go - A Comprehensive Guide
Discover the secrets to effective parallel testing in Go. This guide provides everything you need to write and execute tests faster, enhancing your development workflow.
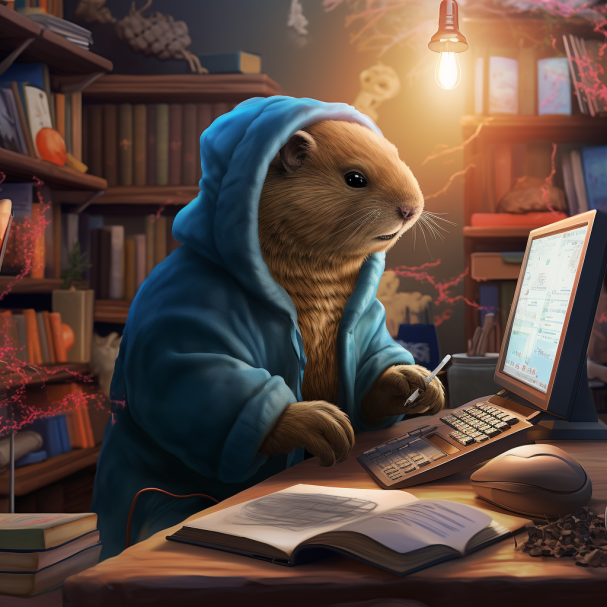
Pointer vs. Value Receivers in Go: Maximizing Efficiency in Your Code
Learn about the fundamental distinctions between pointer and value receivers in Go programming, and how these affect memory management, method performance, and code organization.
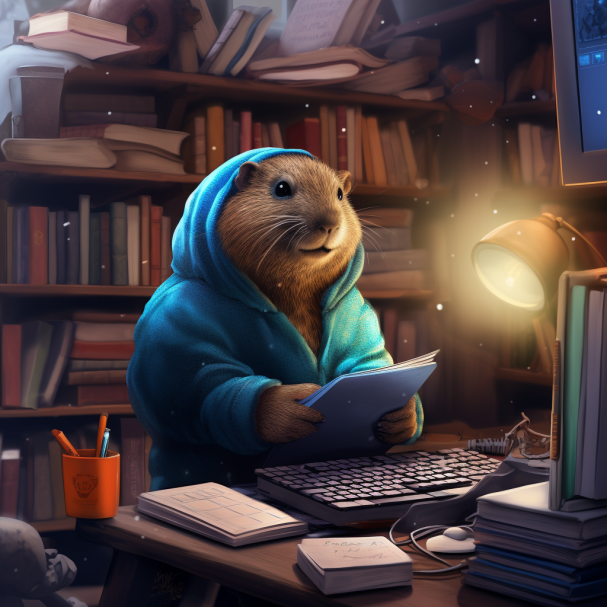
How to Use AWS SQS with Go Channels - A Developer's Guide
Discover how leveraging Go channels can simplify and streamline your interactions with AWS SQS, improving message processing efficiency in your applications.
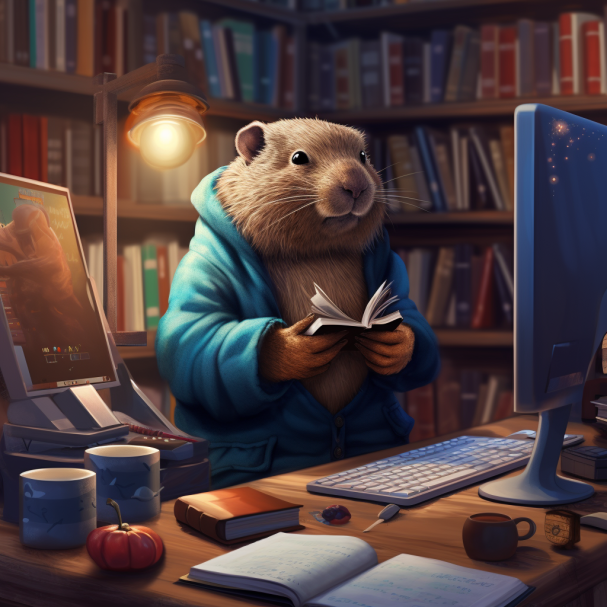
How to Index Documents in Elasticsearch Using the Go-Elasticsearch Library
Learn how to use the Go-Elasticsearch library to index documents efficiently in Elasticsearch, enhancing your data search and retrieval capabilities.

Avoiding Resource Leaks: Safe File Handling with Readers and Writers in Go
Understanding the use of readers and writers in Go can greatly enhance your coding efficiency and capability. This blog post dives deep into how you can leverage these tools with practical code examples.
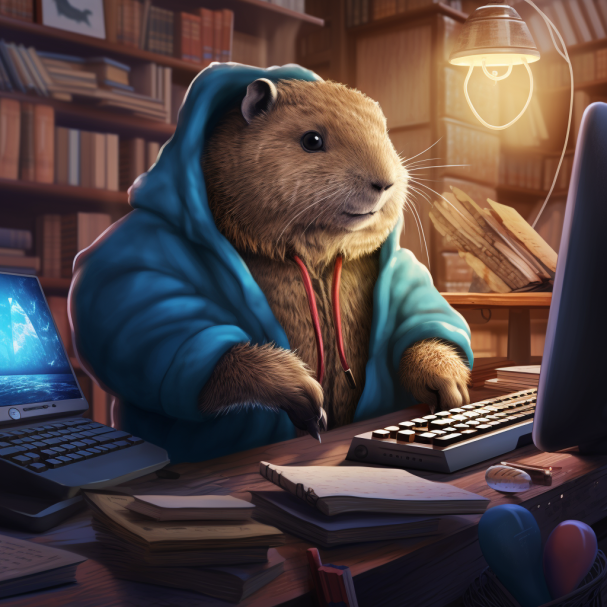
Implementing a Logger Factory in Go: A Comprehensive Guide
Learn to streamline logging in Golang with the factory pattern, creating flexible, scalable applications by dynamically selecting logger types based on your needs.
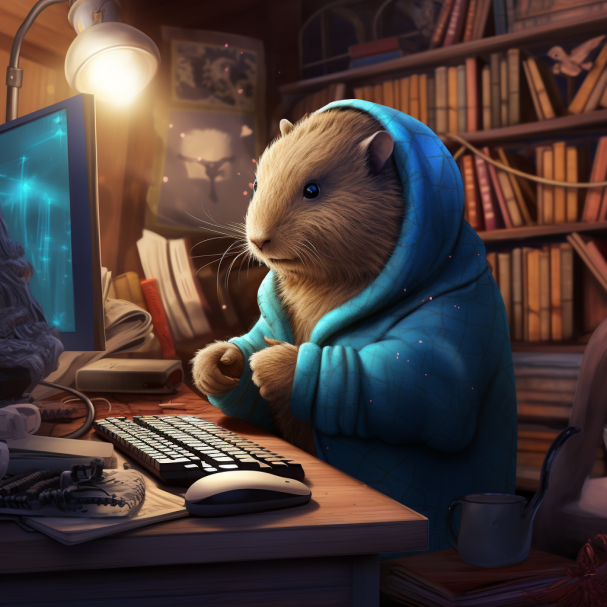
Exploring the Advantages of a Utility Layer in Layered Architecture Design
Layered architecture design has been a cornerstone of software engineering, and the utility layer plays a pivotal role in enhancing its effectiveness. This blog post delves into the numerous advantages of integrating a utility layer, from boosting code reuse to simplifying maintenance tasks.