Curious About Technology
Welcome to Coding Explorations, your go-to blog for all things software engineering, DevOps, CI/CD, and technology! Whether you're an experienced developer, a curious beginner, or simply someone with a passion for the ever-evolving world of technology, this blog is your gateway to valuable insights, practical tips, and thought-provoking discussions.
Recent Posts
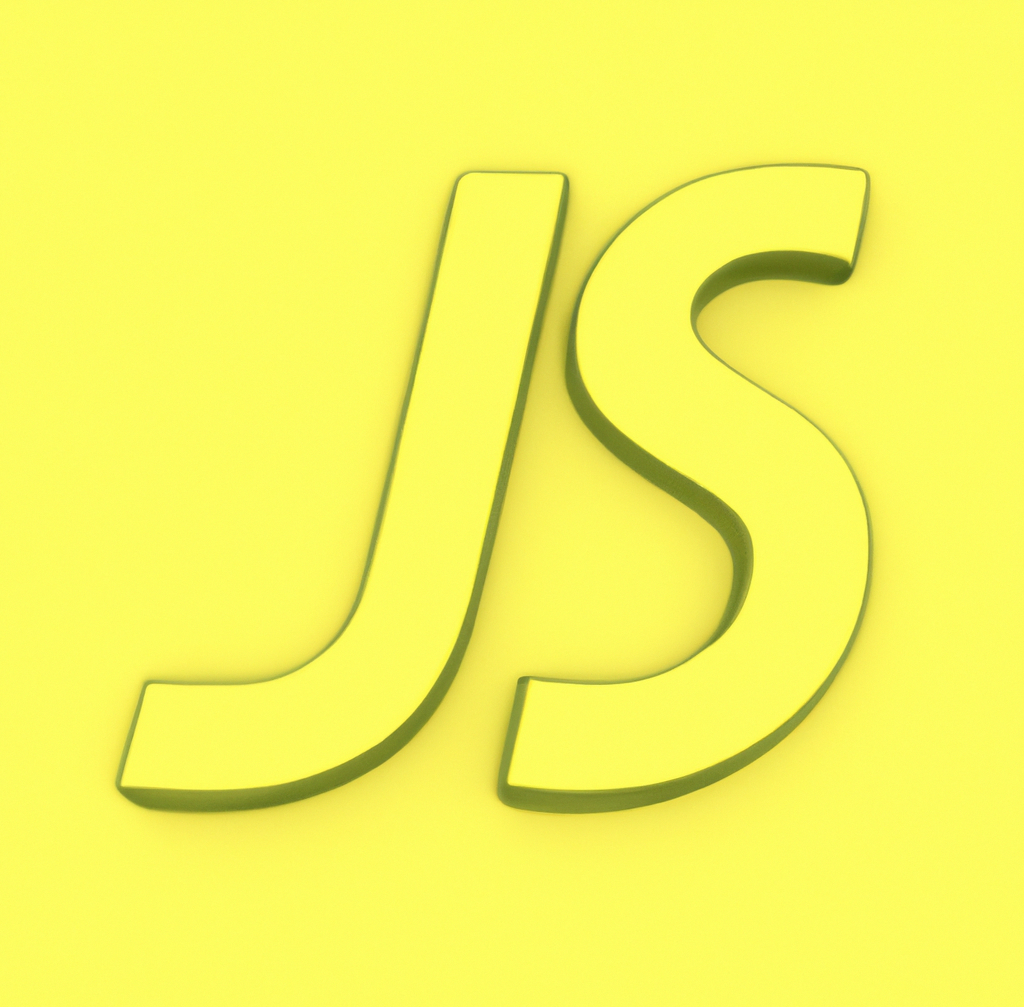
JavaScript's Prototype Chain Explained
JavaScript is a unique language in many ways, and one of its most distinctive features is its approach to inheritance. Unlike many other languages that use classical inheritance, JavaScript uses prototypal inheritance. In this post, we'll dive deep into the prototype chain, explore how it differs from classical inheritance, and look at some practical use cases.

Advanced Go WebAssembly: Exploring the Go Standard Library's Wasm Offerings
In the ever-evolving landscape of web development, WebAssembly (or Wasm for short) is making significant waves by allowing non-JavaScript languages to run in the browser. Go, a statically-typed, compiled language, has embraced this revolution and offers robust support for WebAssembly out of the box.

Getting Started with WebAssembly in Go: A Step-by-Step Guide
WebAssembly (Wasm) has emerged as a potent alternative to JavaScript for web applications. It offers performance improvements, compact binary format, and allows us to use languages other than JavaScript on the web. One of the languages that has extensive support for WebAssembly is Go.
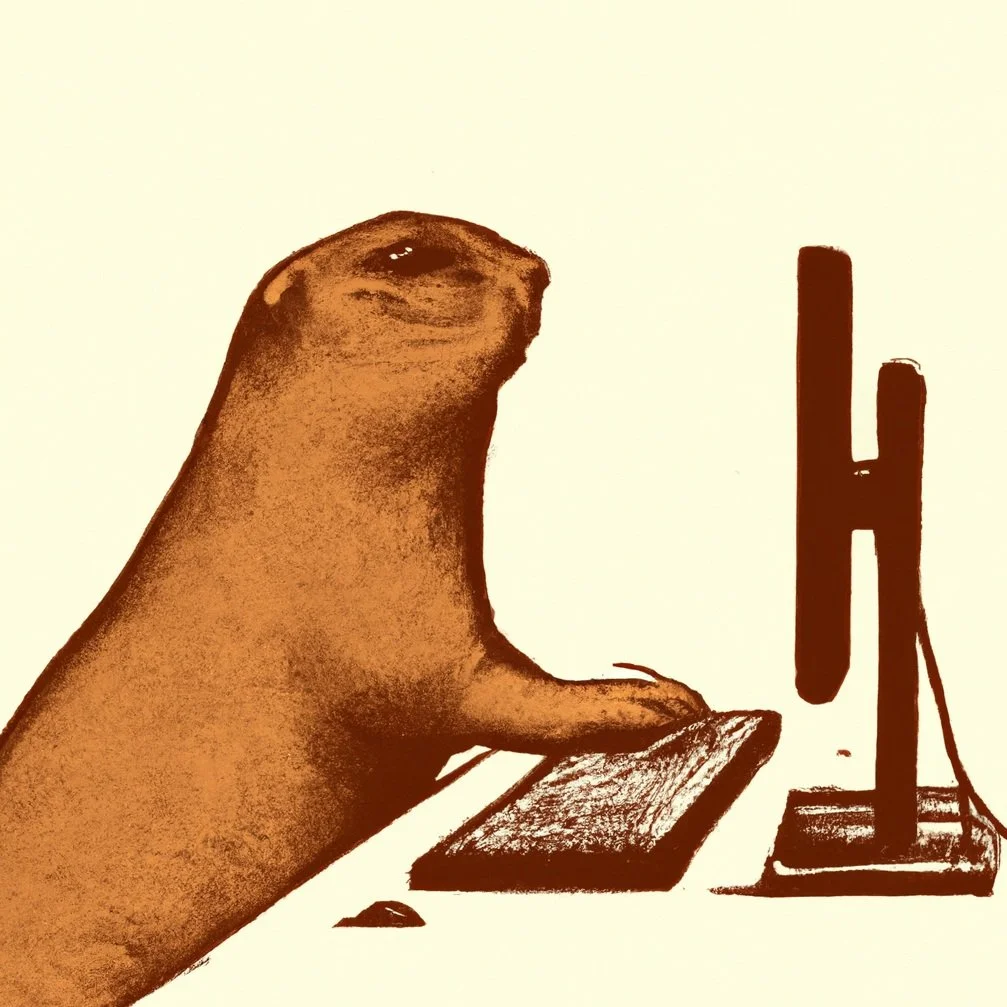
Performance Implications: Generics in Go
Generics, one of the most anticipated features in Go, have opened up a world of possibilities for developers, allowing for more flexible and type-safe code. However, as with any powerful tool, it's essential to understand the performance implications of using generics. In this blog post, we'll delve into how generics can impact the performance of Go programs and offer tips on optimizing code that uses generics.

Go Generics: An Introduction to Constraints and Type Lists
Ever since Go's inception, the community had been debating and contemplating the introduction of generics. After years of anticipation, generics are finally part of the language. One of the core features of generics in Go is the idea of 'Constraints and Type Lists'. This blog post aims to demystify this aspect of Go generics and provide an intuitive understanding of its use cases.
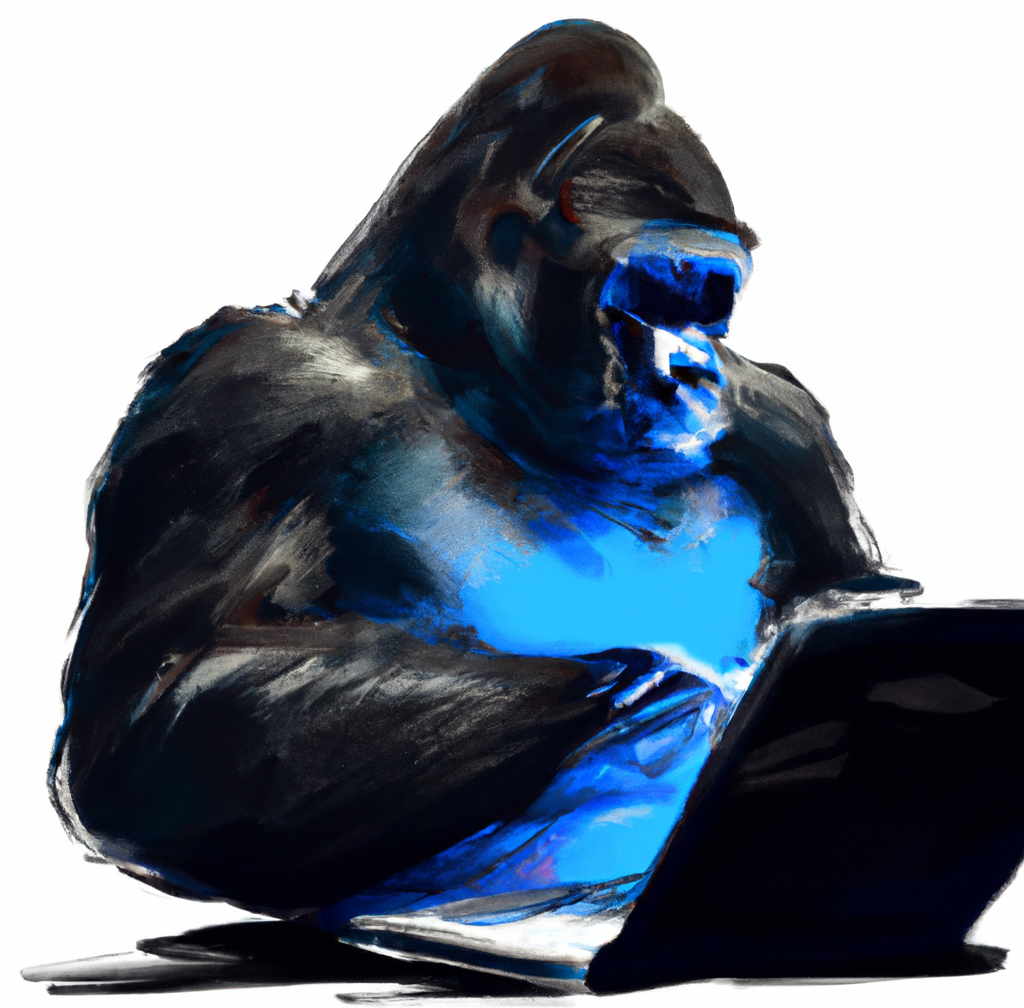
Kubernetes Networking: A Deep Dive with Examples
If you've ever used Kubernetes (or K8s, for the short form lovers), you know it's a powerful orchestration platform for containerized applications. One key aspect of Kubernetes that's fundamental to its operation yet can sometimes seem a bit mysterious is its networking model.

Scaling Applications with Docker Swarm
Containers have revolutionized how applications are developed, packaged, and deployed. They allow us to package our applications along with their dependencies in consistent environments, ensuring that they run the same regardless of where they are deployed. But as our application grows in complexity and traffic, simply running it in a container isn't enough. That's where container orchestration tools like Docker Swarm come into play.
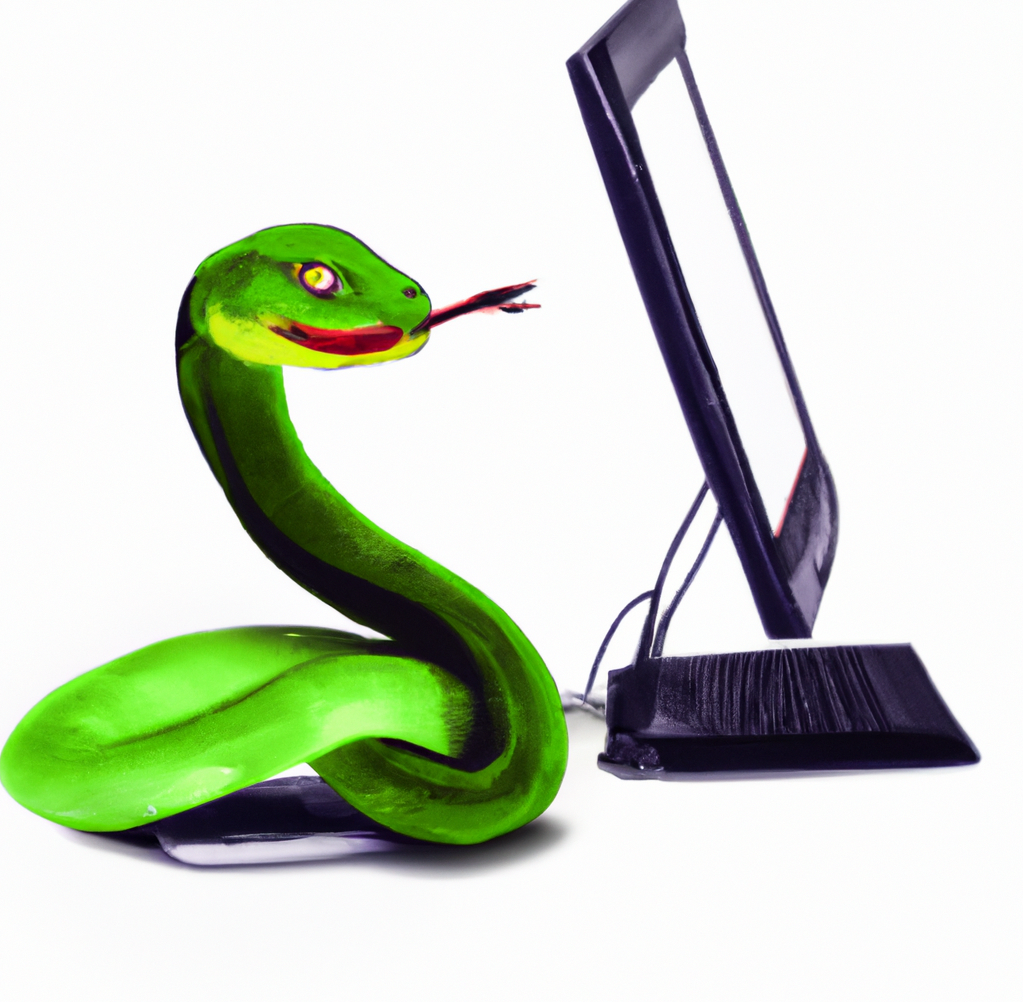
Understanding the Differences between Lists and Tuples in Python
Python, one of the world's most popular programming languages, offers a rich set of data structures to handle collections of items. Two of these structures, lists and tuples, are frequently used by Python developers. At a glance, they might seem similar since both can be used to store collections of items. However, they have key differences that make them unique in their own right. In this blog post, we'll delve deep into the differences between lists and tuples.

Unraveling CGo: Bridging the Gap Between Go and C
While Go (often referred to as Golang) is revered for its simplicity and concurrency model, there are instances when developers may need to interoperate with C libraries, mainly due to performance, leveraging existing libraries, or for compatibility reasons. This is where CGo comes into play. CGo provides a mechanism to integrate Go code with C code seamlessly.

Merge Sort Using Concurrency
Concurrency can significantly enhance the performance of many algorithms, and merge sort is no exception. By parallelizing specific tasks, we can often gain faster results by harnessing the power of multiple processing units. This blog post will dive into the idea of improving the merge sort algorithm using concurrency and then illustrate it with an example written in Go.

Understanding Memory Escape in Golang
One of the strongest selling points for Go (often referred to as Golang) is its powerful and transparent memory management, backed by a built-in garbage collector. However, as with many high-level languages, understanding the nuances of how memory is managed can lead to more performant code. A key concept in this area for Go developers is "memory escape."

Working with Lists in Python: A Guide
Lists are one of the most basic and widely used data structures in Python. They are versatile, simple to use, and offer a wide range of methods that allow for various manipulations. In this blog post, we will explore some fundamental operations that can be performed on lists in Python.

Understanding Memory Leaks in Go and How to Avoid Them
Go (or Golang) is admired for its simplicity, efficiency, and robustness. One of the reasons behind its rapid adoption is its built-in garbage collector which automatically frees memory that is no longer being used. Yet, despite having a garbage collector, memory leaks can still plague Go applications. In this post, we'll delve into memory leaks in Go: what they are, how to identify them, and, most importantly, how to prevent them.

Scaling Applications with Docker Swarm: Achieving Horizontally Scalable and Highly Available Systems
In the ever-evolving landscape of modern software development, the need for efficient and scalable solutions has become paramount. Enter Docker Swarm, a powerful container orchestration platform that allows developers to manage, scale, and deploy applications seamlessly. In this blog post, we'll delve into the world of Docker Swarm and explore how it empowers us to scale applications horizontally, handle load balancing, and ensure high availability.

Websockets and Real-Time Applications in Go: Building Interactive Experiences
In the ever-evolving landscape of web development, real-time communication has become a quintessential feature for creating interactive and engaging user experiences. Websockets have emerged as a powerful solution for enabling real-time communication between clients and servers. In this blog post, we will delve into the wo

Advanced Concurrency Patterns in Go
When developers start exploring Go (or "Golang"), they're often drawn to its simplicity and elegance, especially in handling concurrent programming. Thanks to goroutines and channels, Go makes concurrency a lot more manageable. But to truly tap into the power of Go's concurrency model, we need to dive deeper. This article will discuss advanced concurrency patterns like worker pools, fan-out/fan-in, and rate limiting.

Memory Management and Profiling in Go
Go, often referred to as Golang, is recognized for its simplicity and performance. A key part of maintaining that performance is understanding how memory is managed within a Go application. In this blog post, we'll dive deep into Go's memory management model, examine garbage collection, and explore tools and techniques to profile memory usage.

Building Command-Line Tools with Cobra: Mastering Aspects of Go
Command-line tools have been an integral part of software development since the earliest days of computing. They provide a quick and efficient way to run operations, scripts, or even entire applications, all from the comfort of your terminal. Today, we'll dive into how to create feature-rich command-line applications using the Cobra library in Go.
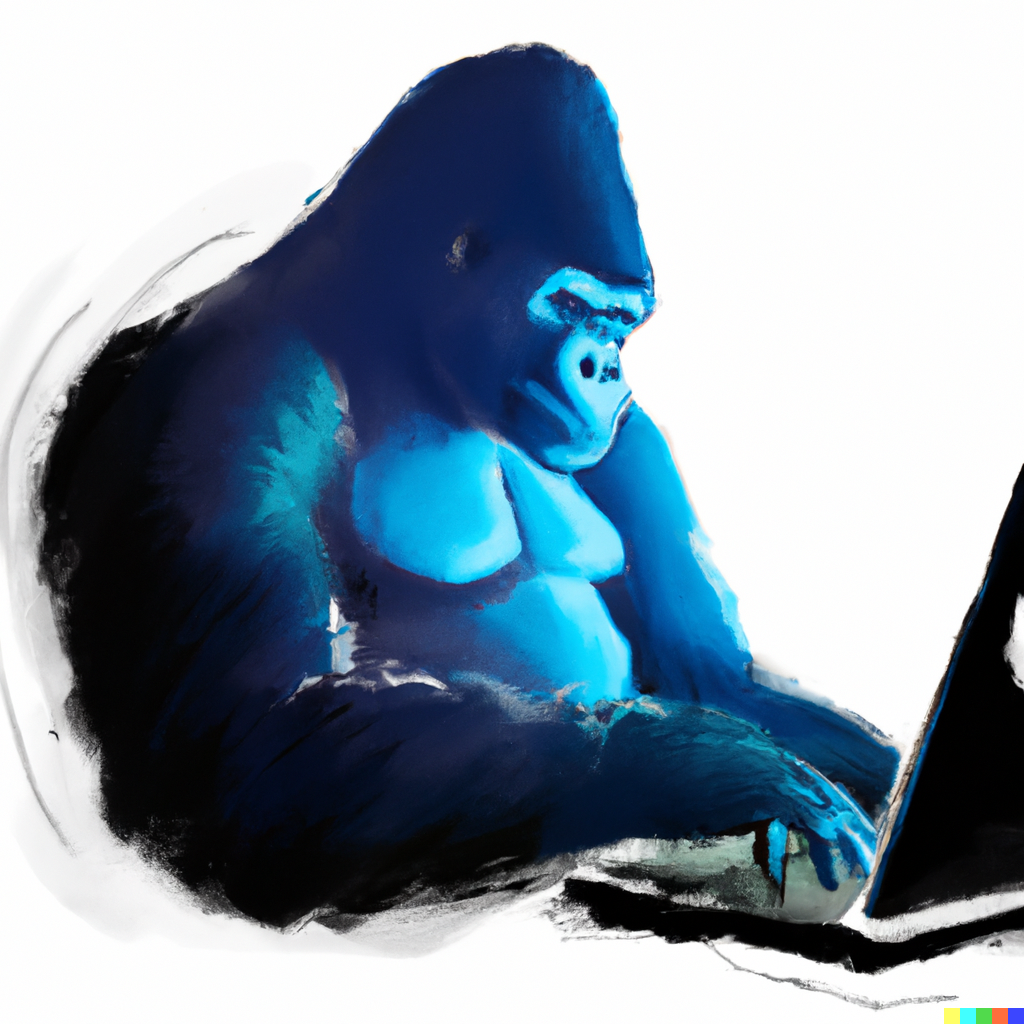
Advanced Use Cases with AWS Services: EC2, S3, and SQS
AWS, or Amazon Web Services, is the most comprehensive and widely adopted cloud platform in the world. It offers a vast range of cloud services, including computing power, storage options, and networking capabilities that help businesses scale and grow. In this blog post, we'll delve deeper into advanced uses of specific AWS services, including EC2 (Elastic Compute Cloud), S3 (Simple Storage Service), and SQS (Simple Queue Service). We will also address some specific questions about these services.
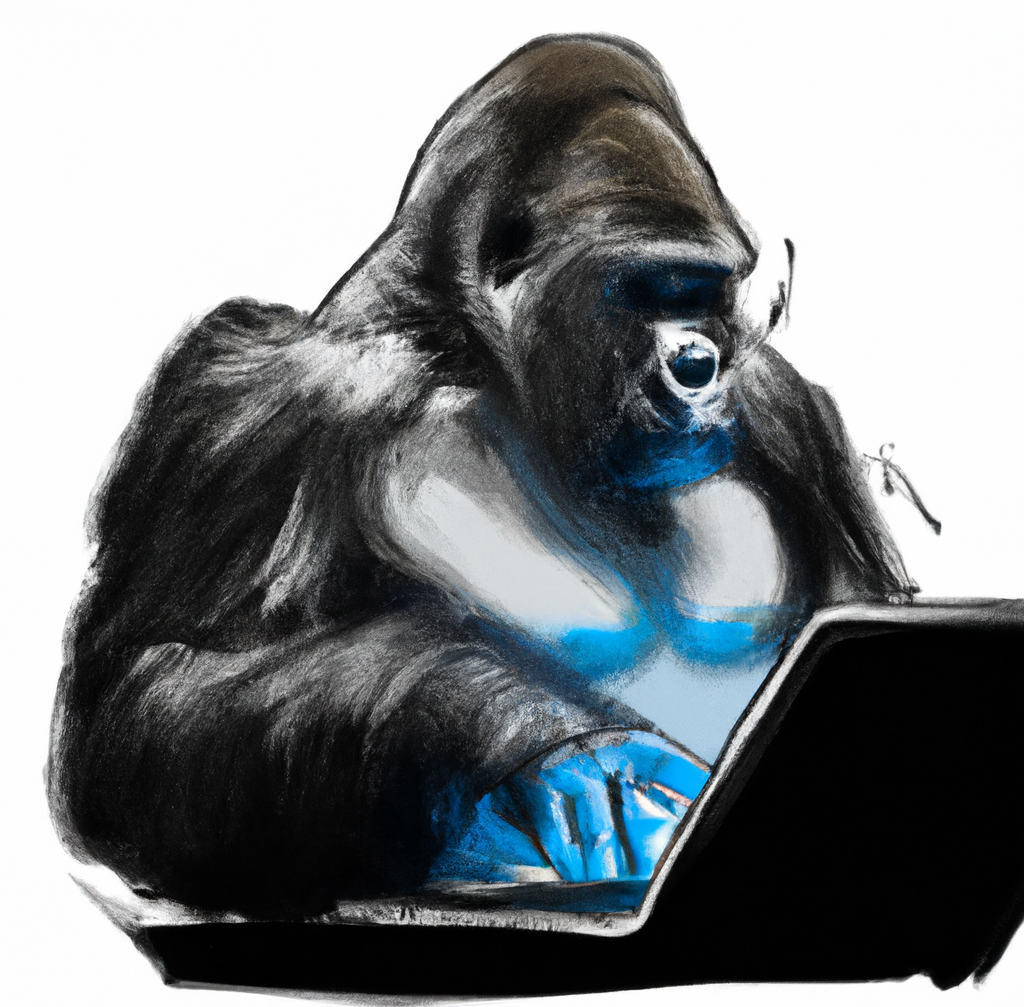
Using Bash to Build an AWS S3 Tool
Amazon S3, a reliable and scalable object storage service by AWS, is extensively used to store and manage data. In this blog post, we will walk through creating a simple bash script that allows you to list objects in an S3 bucket and download them using arguments passed through the command line.