Curious About Technology
Welcome to Coding Explorations, your go-to blog for all things software engineering, DevOps, CI/CD, and technology! Whether you're an experienced developer, a curious beginner, or simply someone with a passion for the ever-evolving world of technology, this blog is your gateway to valuable insights, practical tips, and thought-provoking discussions.
Recent Posts
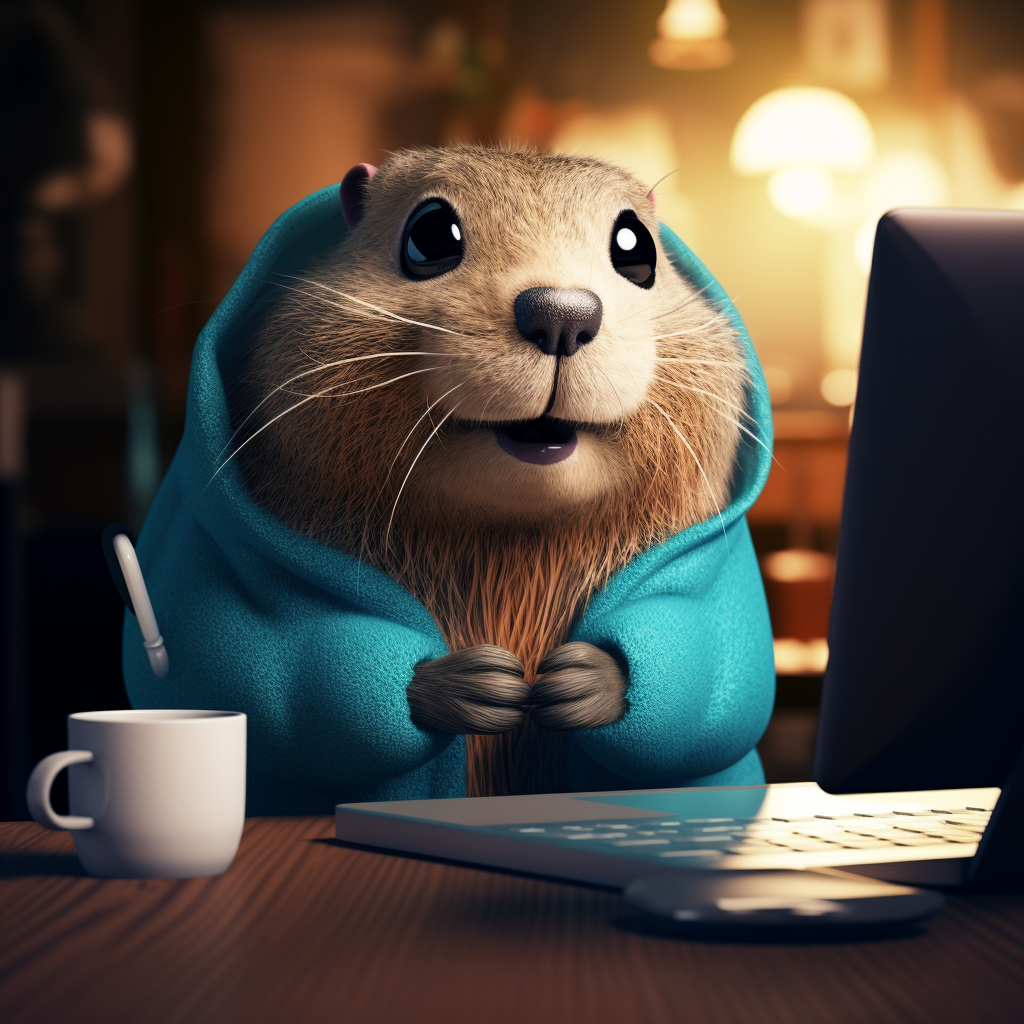
Golang Closures: From Mystery to Proficiency
The Go programming language has surged in popularity since its inception due to its simplicity, concurrency support, and strong standard library. Among its features, one that often intrigues new developers is the concept of closures. Let's take a deep dive into what closures are in Go and why they're so powerful.

Go Testing with Fake HTTP Requests and Responses
Go provides a robust testing framework right out of the box. When working with web applications or services, a common challenge developers face is testing code that involves HTTP requests and responses without actually hitting the real endpoints. In this post, we'll dive deep into creating and using fake HTTP requests and responses for testing in Go.
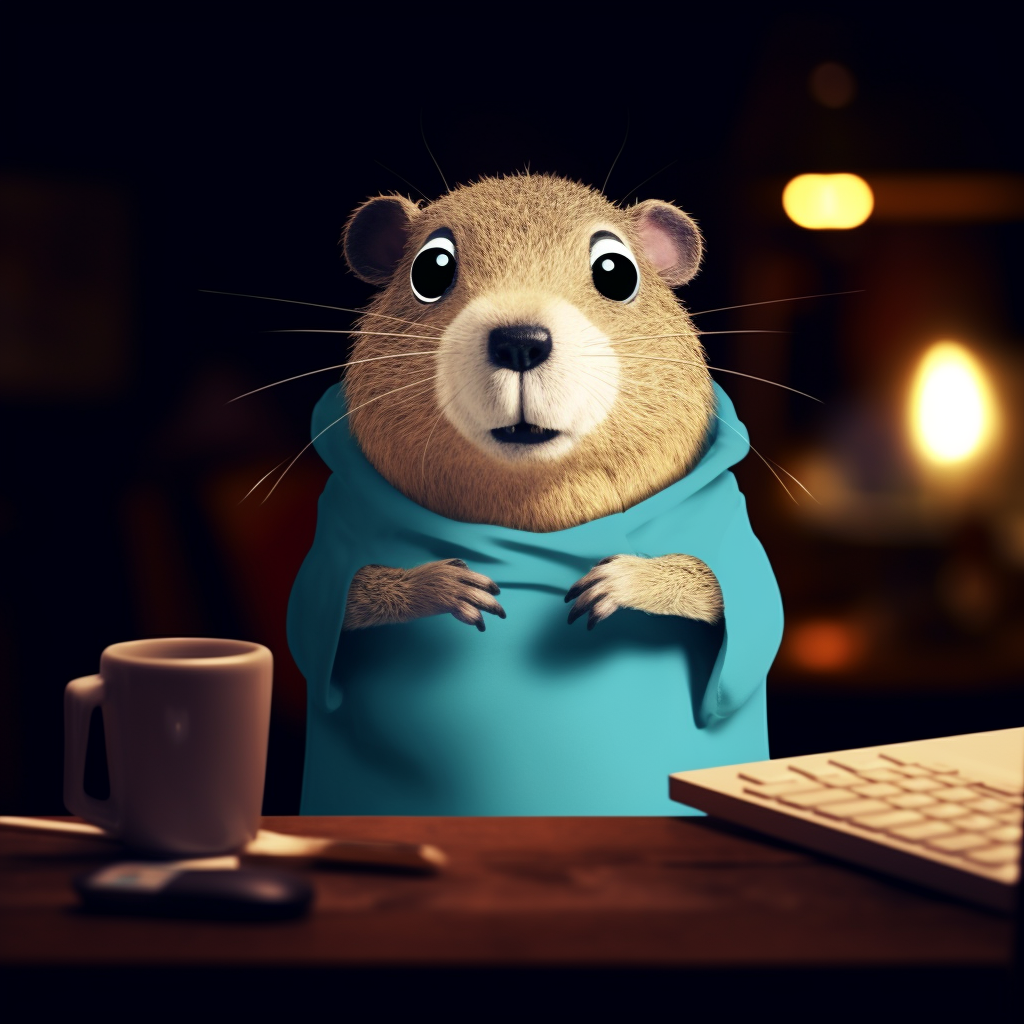
Table-Driven Testing in Go
In the realm of software development, particularly in Go, testing plays a pivotal role. One of the most effective and popular testing paradigms in the Go community is "Table-Driven Testing". Let's delve into what it is, its benefits, and how to effectively employ it.
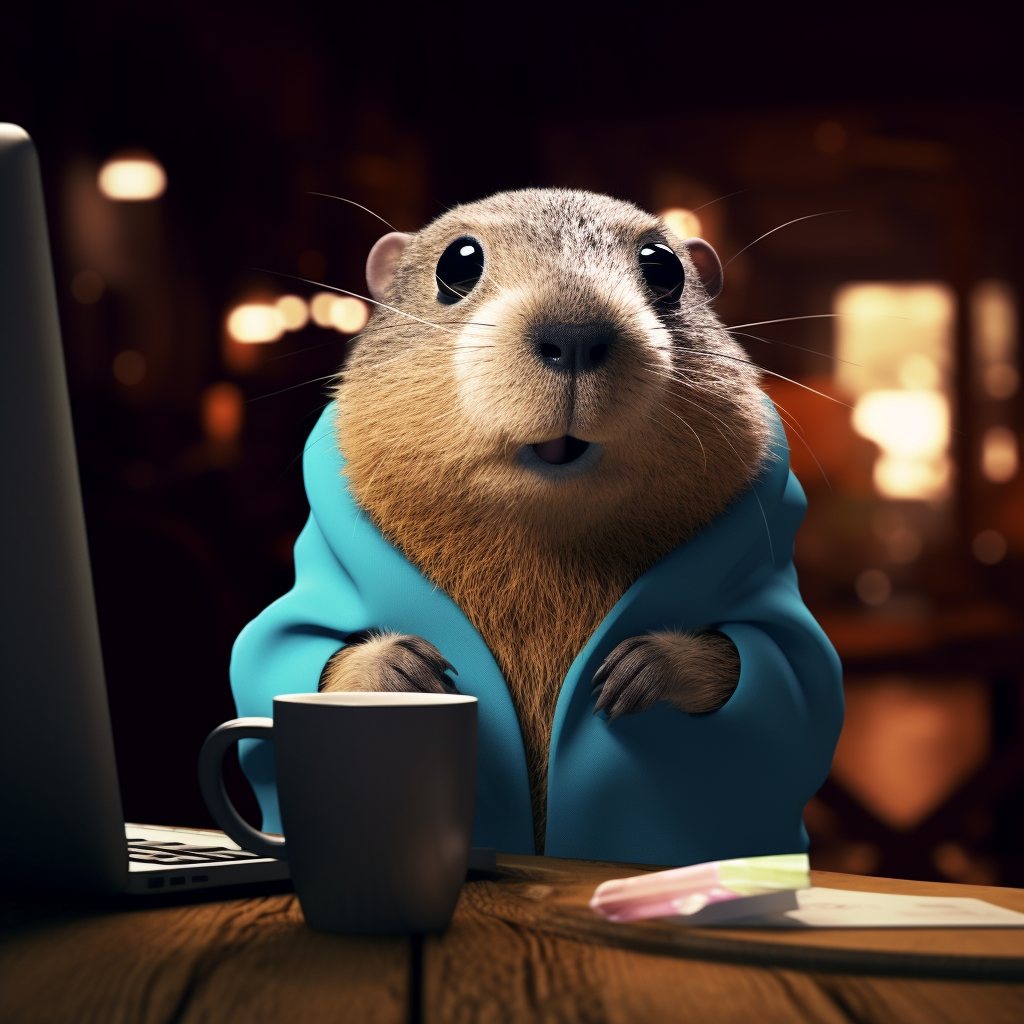
Dependency Injection in Go: A Primer
Dependency Injection (DI) is a software design pattern that allows for decoupling components and layers in a system. By providing dependencies from the outside rather than hard-coding them within a component, we achieve better modularity, testability, and flexibility. Go, despite its simplicity, can be enhanced with DI patterns to build scalable and maintainable applications. In this blog post, we'll explore what Dependency Injection is, why it's useful, and how to implement it in Go with code examples.

Implementing the Singleton Pattern in Go
The Singleton pattern ensures that a particular class has only one instance throughout the runtime of an application and provides a global point of access to that instance. In the Go programming language, often referred to as "Golang", implementing the Singleton pattern is relatively straightforward, thanks to its inherent concurrency and package-oriented nature.
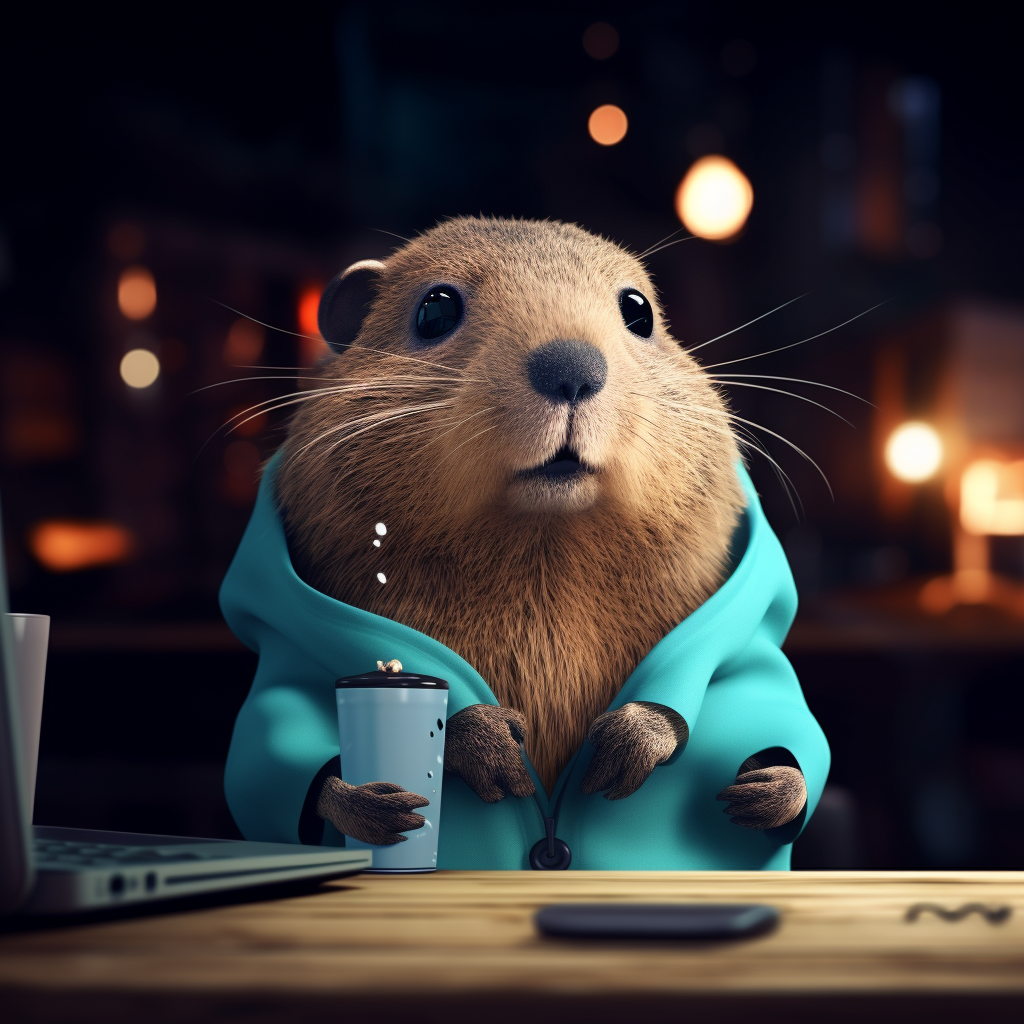
WaitGroup in Go: How to Coordinate Your Goroutines Effectively
Concurrency is one of the hallmarks of the Go programming language. While there are many tools and mechanisms in Go to help you harness the power of concurrency, one of the simplest yet most powerful tools at your disposal is the WaitGroup from the sync package.
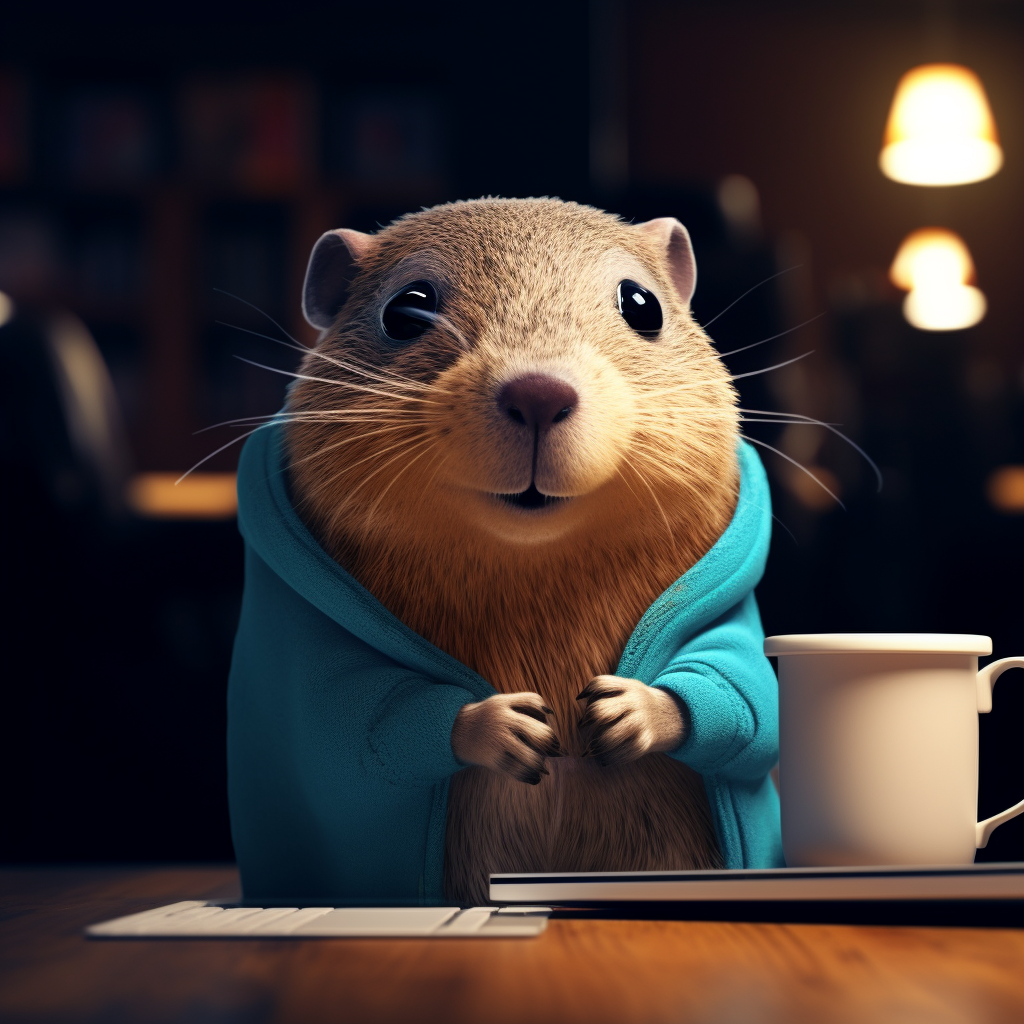
Testing GORM with SQLMock
GORM is one of the most popular ORM (Object-Relational Mapper) libraries in Go, providing a simplified and consistent way to interact with databases. As with all application code, it's imperative to ensure that your database operations are thoroughly tested. However, hitting a real database during unit tests isn't ideal. This is where SQLMock comes in. SQLMock provides a way to mock out your SQL database so you can test your GORM code without needing a live database.

Defensive Programming in Go: The Power of defer and Nil Checks
In the vast ecosystem of software development, safety and robustness are two of the primary goals every developer should aspire to achieve. As our software becomes an integral part of modern infrastructure, the margin for error narrows. Ensuring that our software behaves predictably even under unexpected conditions is crucial. In this blog post, we’ll delve into two core techniques that bolster safety in the Go programming language: using the defer statement to ensure resources are cleaned up and guarding against nil pointer dereferences.
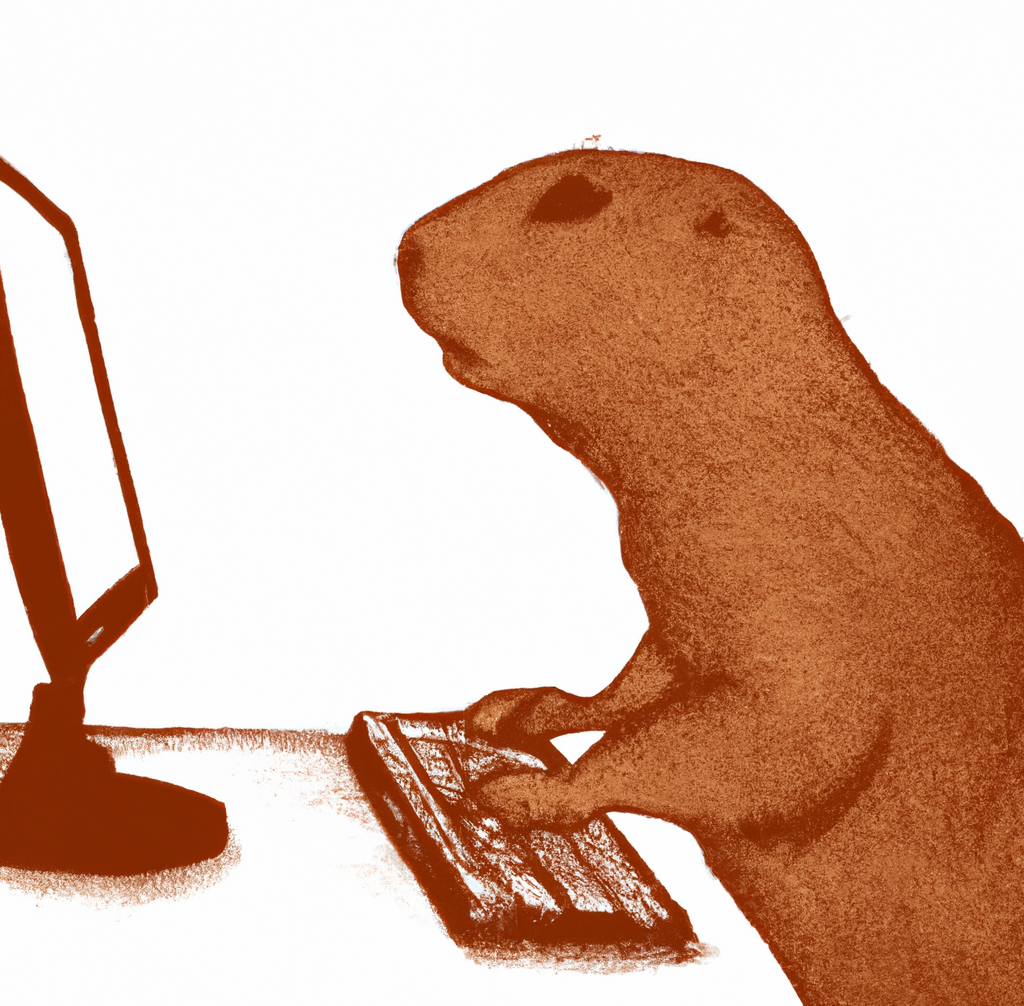
Graceful Shutdown in Go: Safeguarding Containerized Applications
In today's container-driven world, applications must be prepared to handle interruptions and terminations with grace and elegance. Especially in orchestrated environments like Kubernetes, an application could be rescheduled, moved, or terminated due to a myriad of reasons. For developers, this emphasizes the importance of ensuring their application can handle shutdown signals gracefully. Go (or Golang), with its lightweight concurrency model and robust standard library, offers tools to achieve a graceful shutdown. Let's delve into how we can implement this in a Go application.

Reducing Binary Size in Go: Strip Unnecessary Data with -ldflags "-w -s"
When building Go applications, the generated binary can sometimes be larger than what you might expect, especially when it contains debug information for the Go runtime and symbols for the linker. Fortunately, Go provides a handy way to reduce the binary size by stripping away unnecessary data. In this post, we'll dive into how to use the -ldflags "-w -s" option with go build to achieve this.

Deploying Go Applications: Options and Best Practices
Go is quickly becoming a popular choice among developers for building fast, reliable, and scalable applications. Due to its efficiency and lightweight nature, Go apps often require unique deployment considerations. Let's delve into some of the options available and best practices for deploying Go applications.

Creating a RESTful API with JWT Authentication in Go
Go has been a favorite among many developers when it comes to backend development. Its simplicity, combined with performance benefits, makes it a powerful tool for web services. In this tutorial, we'll guide you through creating a RESTful API using Go, with a focus on adding JWT (JSON Web Tokens) for authentication.
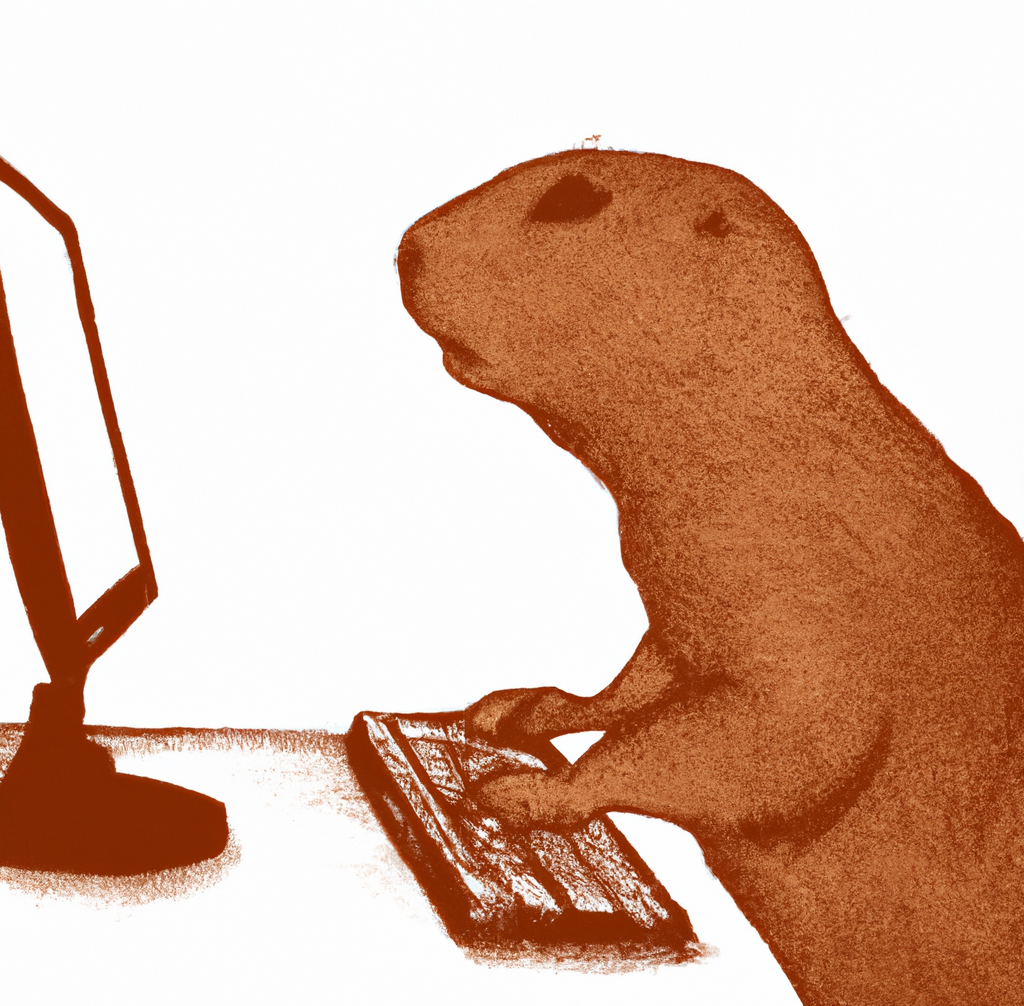
Using Docker to Run Unit and Integration Tests with Go
Docker has revolutionized the way we develop, build, and ship applications. It offers consistent, repeatable, and isolated environments, which makes it an ideal choice for running tests. In this blog post, we'll explore how to use Docker to run unit and integration tests for a Go application.

Utilizing Protocol Buffers (Protobuf) to Create API Contracts Between Web Server and Client
As web applications continue to evolve, so does the necessity for efficient, scalable, and clear communication protocols between the server and the client. Traditional methods like RESTful APIs using JSON or XML have been popular, but there's another player in the arena: Protocol Buffers, or "Protobuf" for short. In this blog post, we'll explore how Protobuf can be used to establish clear API contracts between a web server and a client.
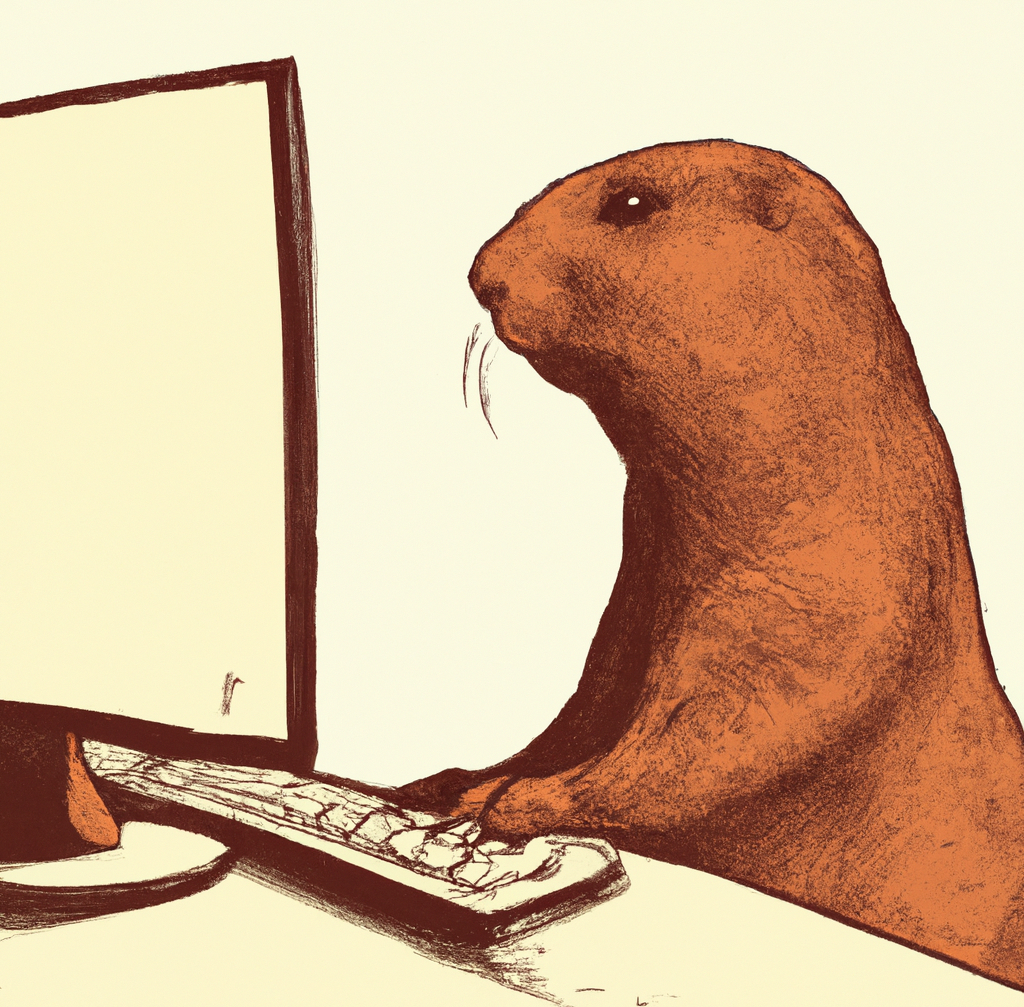
Packages and Modularization in Go: A Comprehensive Guide
In the world of software development, modularization is a key principle that ensures maintainability, reusability, and clarity. In the Go programming language, this principle is embodied through the use of packages and modules. In this blog post, we'll delve deep into the world of Go packages, Go Modules, and the best practices surrounding them.

Go and Machine Learning: An Emerging Duo
Machine Learning (ML) has been synonymous with Python for a long time. Libraries such as TensorFlow, PyTorch, and Scikit-learn have made Python the go-to language for ML. However, the landscape is ever-evolving, and there's a budding interest in using Go (or Golang) in the ML space.

Implementing a Red-Black Tree in Golang
Red-Black Trees are a type of self-balancing binary search tree. Each node in the tree has an extra attribute for denoting the color of the node, either red or black.

Reflection and the reflect Package in Go
Reflection in programming refers to the ability of a program to inspect its own structure, particularly through types; it's a form of metaprogramming. In Go, this capability is provided by the reflect package. While powerful, reflection can be tricky and should be used judiciously.

Advanced Go WebAssembly: Exploring the Go Standard Library's Wasm Offerings
In the ever-evolving landscape of web development, WebAssembly (or Wasm for short) is making significant waves by allowing non-JavaScript languages to run in the browser. Go, a statically-typed, compiled language, has embraced this revolution and offers robust support for WebAssembly out of the box.

Getting Started with WebAssembly in Go: A Step-by-Step Guide
WebAssembly (Wasm) has emerged as a potent alternative to JavaScript for web applications. It offers performance improvements, compact binary format, and allows us to use languages other than JavaScript on the web. One of the languages that has extensive support for WebAssembly is Go.