Curious About Technology
Welcome to Coding Explorations, your go-to blog for all things software engineering, DevOps, CI/CD, and technology! Whether you're an experienced developer, a curious beginner, or simply someone with a passion for the ever-evolving world of technology, this blog is your gateway to valuable insights, practical tips, and thought-provoking discussions.
Recent Posts
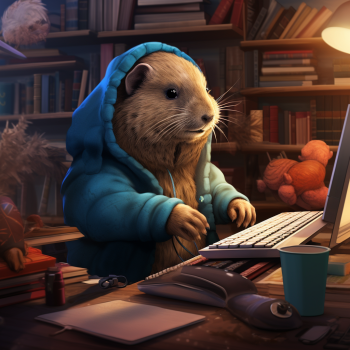
Goroutine Pools Explained: Maximize Efficiency in Go Applications
Goroutine pools are a critical component for efficient resource management in Go programming. This blog explains their importance, how to implement them, and best practices with actionable code examples.
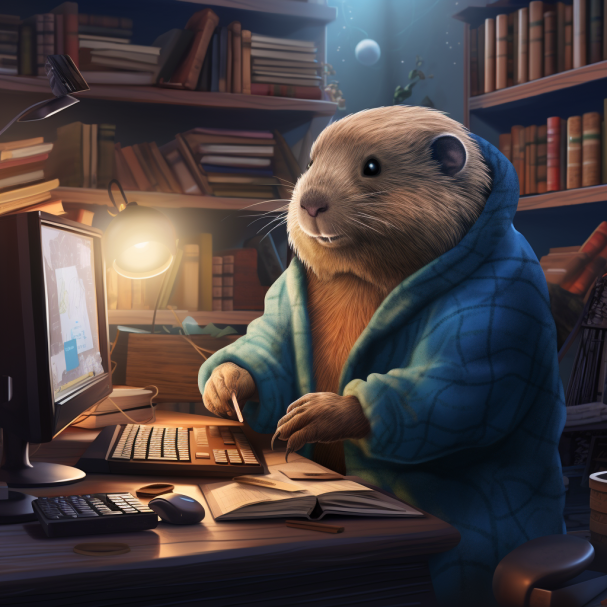
Leveraging Go's Concurrency for High-Performance Database Insertions
In the realm of software development, efficiently managing database operations is crucial for ensuring high performance and scalability. Go, with its built-in support for concurrency, offers an effective way to enhance the speed and efficiency of inserting records into a database.
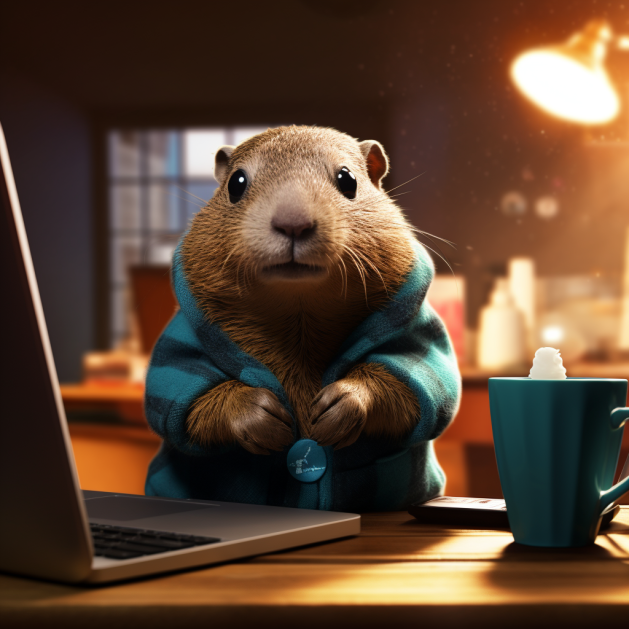
How to Effectively Unit Test Goroutines in Go: Tips & Tricks
Unit testing in Go, especially when it involves goroutines, presents unique challenges due to their concurrent nature. Goroutines are lightweight threads managed by the Go runtime, and they are used to perform tasks concurrently.

Understanding Variable Passing into Go Routines
Go is a statically typed, compiled programming language designed for simplicity and efficiency, with a particular emphasis on concurrent programming. One of the core features of Go's concurrency model is goroutines, which are functions capable of running concurrently with other functions. A common question among Go developers, especially those new to the language, is why and how to pass variables into a goroutine.
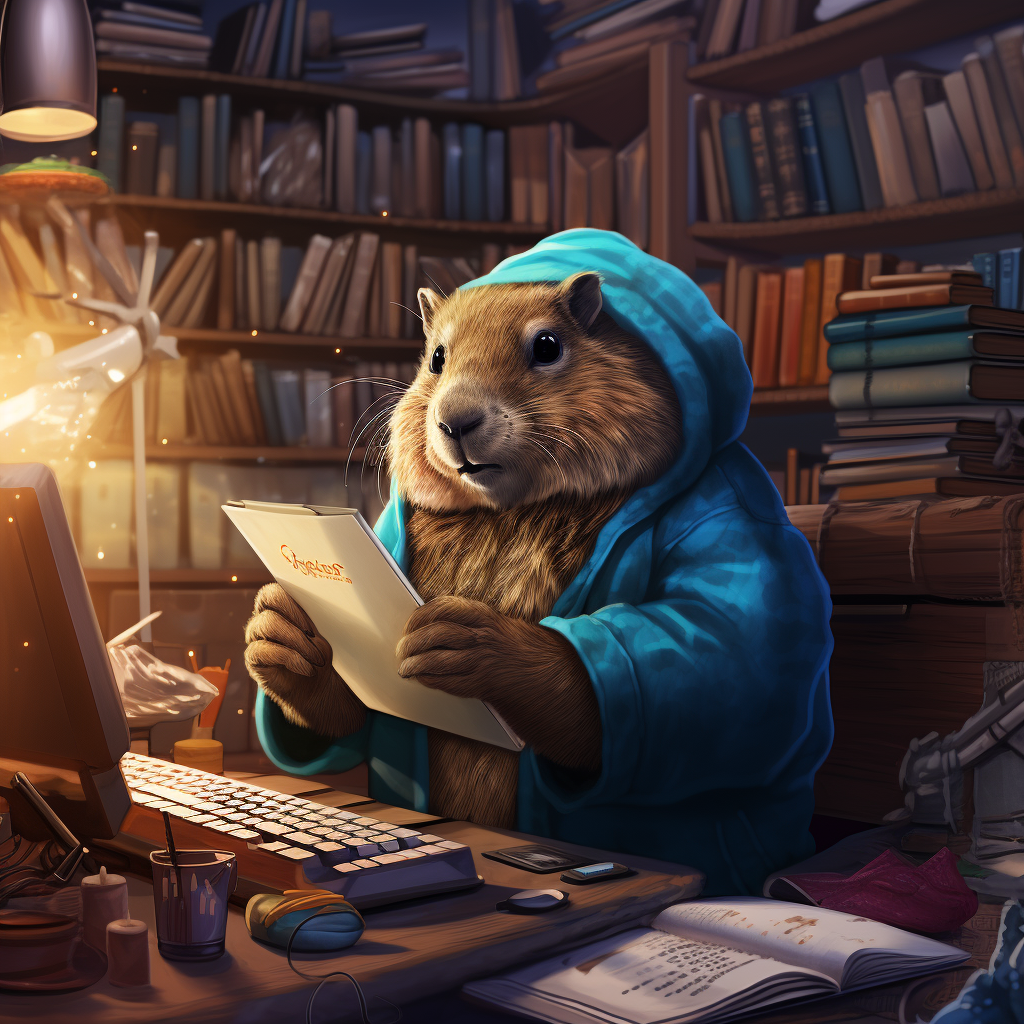
Efficient File Processing in Go: Leveraging Worker Groups and Goroutines
In today's data-driven world, efficient file processing is a critical task for many applications. Go, with its robust concurrency model, offers a powerful way to handle such tasks efficiently.
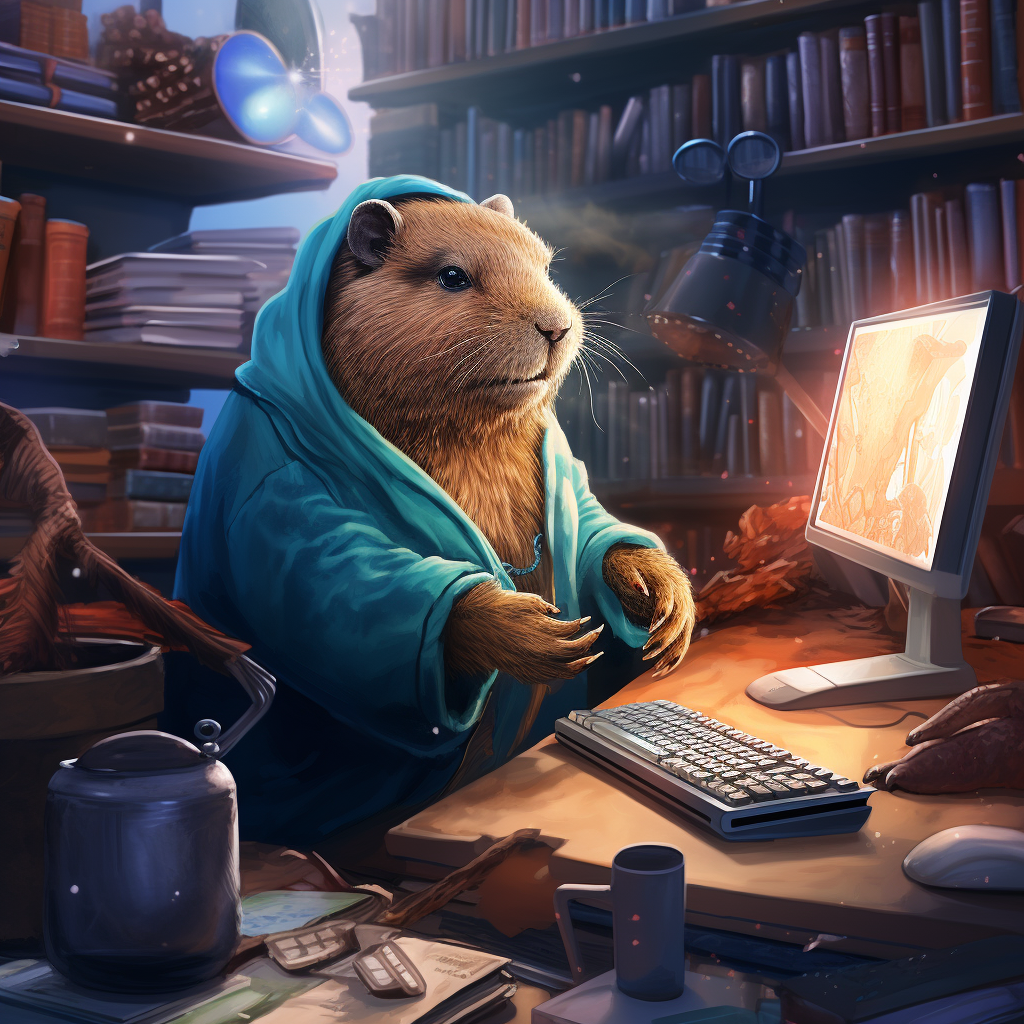
Harnessing the Power of the Yield Function in Go
o is a statically typed, compiled language that has gained immense popularity for its simplicity, performance, and concurrency support. While Go offers a wide range of features to make concurrent programming more manageable, one of its lesser-known gems is the 'yield' function.
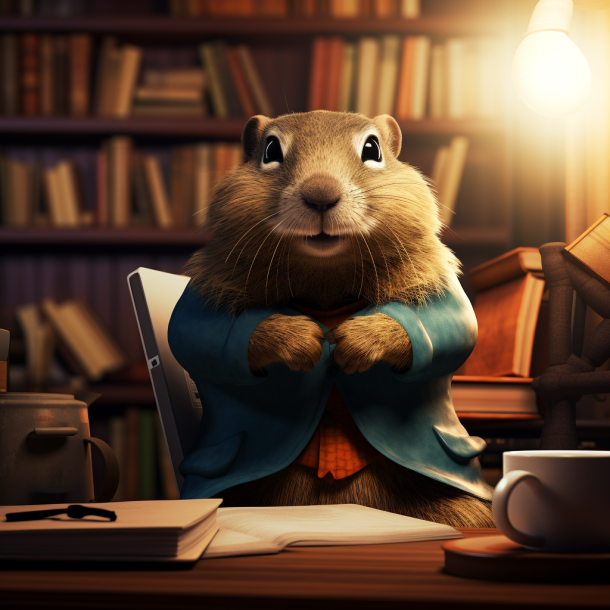
Interview Series: Understanding Goroutines in Go
When it comes to concurrent programming, the concept of 'threads' is often one of the first that comes to mind. However, if you've dipped your toes into the Go programming language, you may have come across a curious term: 'goroutine'. This unique approach to concurrency is one of the features that sets Go apart from other programming languages. But what exactly is a goroutine, and how does it differ from a thread in traditional threading models?

Go Concurrency Patterns: Diving into Fan-in and Fan-out
One of the primary reasons developers love Go is its built-in concurrency support. When discussing concurrency in Go, two core patterns emerge: Fan-in and Fan-out. These concepts help in building scalable and efficient systems. Let's dive into what these patterns mean and how they're implemented in Go.
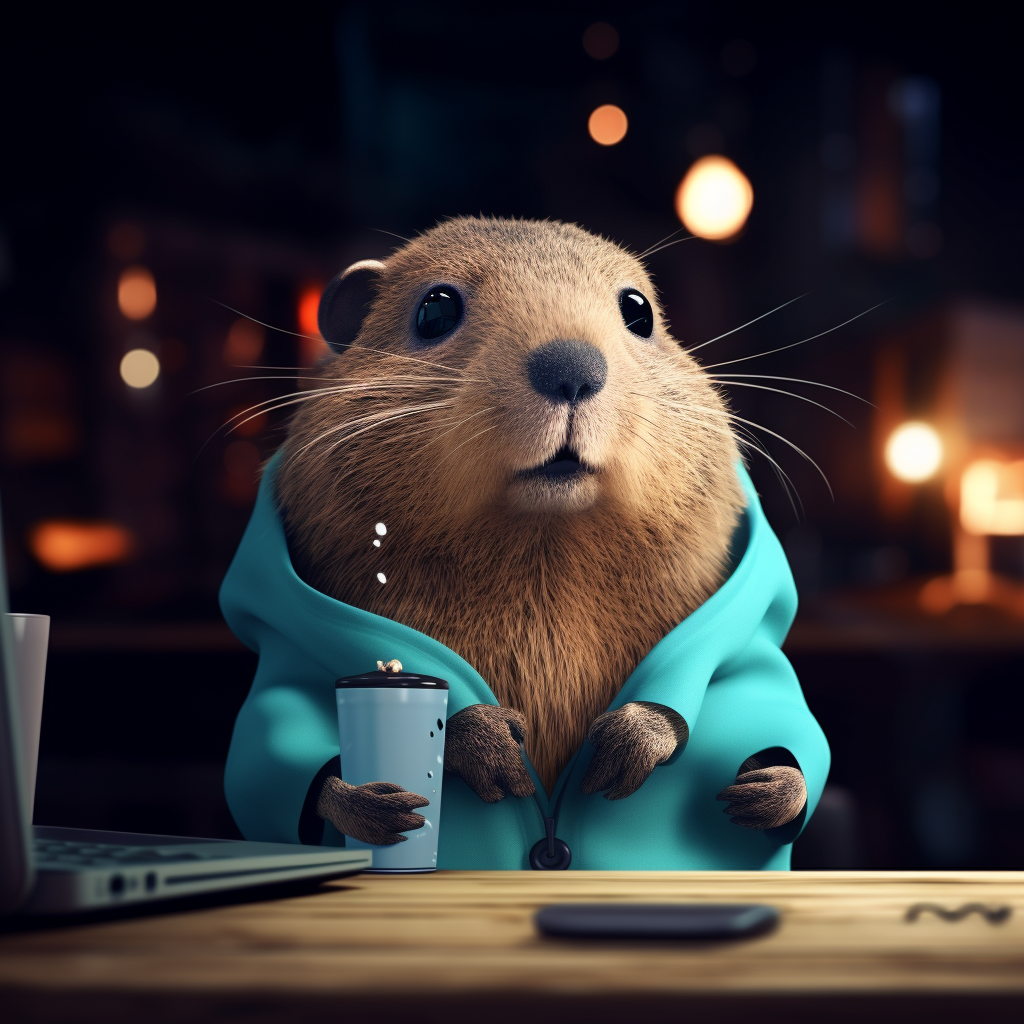
WaitGroup in Go: How to Coordinate Your Goroutines Effectively
Concurrency is one of the hallmarks of the Go programming language. While there are many tools and mechanisms in Go to help you harness the power of concurrency, one of the simplest yet most powerful tools at your disposal is the WaitGroup from the sync package.

Understanding Go's Goroutine, Mutex, and Channel (GMP) Model
One of the standout features that make Go so popular is its ability to handle concurrent programming efficiently. The Go runtime introduces a powerful concurrency model known as the GMP model, which comprises Goroutines, Mutexes, and Channels. In this blog, we'll delve into the GMP model and understand how it enables developers to write concurrent programs that are reliable, safe, and performant.

Unveiling the Magic of Goroutines: How Concurrency Works in Go
Concurrency is a crucial aspect of modern software development, enabling programs to efficiently execute multiple tasks simultaneously. In the Go programming language, concurrency is achieved through Goroutines. Goroutines are lightweight, independently executing functions or methods that can run concurrently with other Goroutines within the same program.