Curious About Technology
Welcome to Coding Explorations, your go-to blog for all things software engineering, DevOps, CI/CD, and technology! Whether you're an experienced developer, a curious beginner, or simply someone with a passion for the ever-evolving world of technology, this blog is your gateway to valuable insights, practical tips, and thought-provoking discussions.
Recent Posts

Function Parameters Simplified: Option Structs vs. Variadic Parameters
In programming, functions are the building blocks that allow us to encapsulate behavior and reuse code. However, designing function parameters can be challenging, especially when a function needs to handle a variety of input configurations. Two common techniques to address this challenge are option structs and variadic parameters. Each approach has its own benefits and trade-offs.
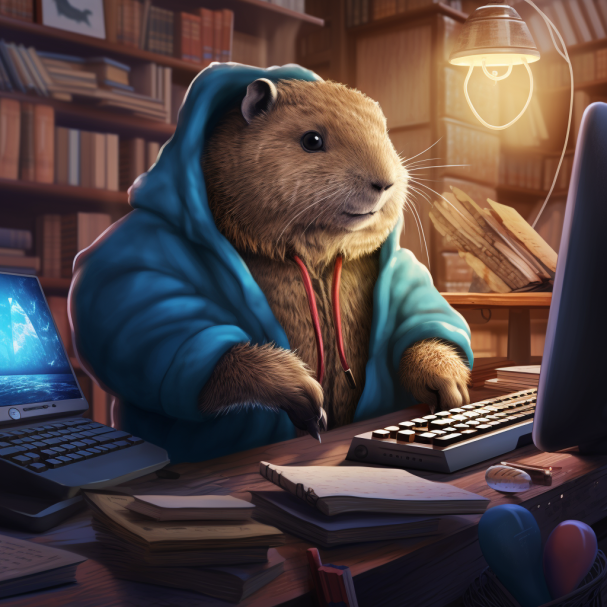
Implementing a Logger Factory in Go: A Comprehensive Guide
Learn to streamline logging in Golang with the factory pattern, creating flexible, scalable applications by dynamically selecting logger types based on your needs.
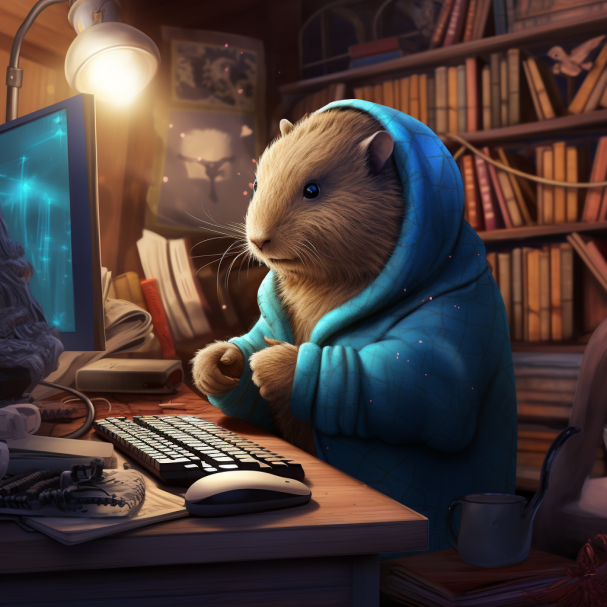
Exploring the Advantages of a Utility Layer in Layered Architecture Design
Layered architecture design has been a cornerstone of software engineering, and the utility layer plays a pivotal role in enhancing its effectiveness. This blog post delves into the numerous advantages of integrating a utility layer, from boosting code reuse to simplifying maintenance tasks.
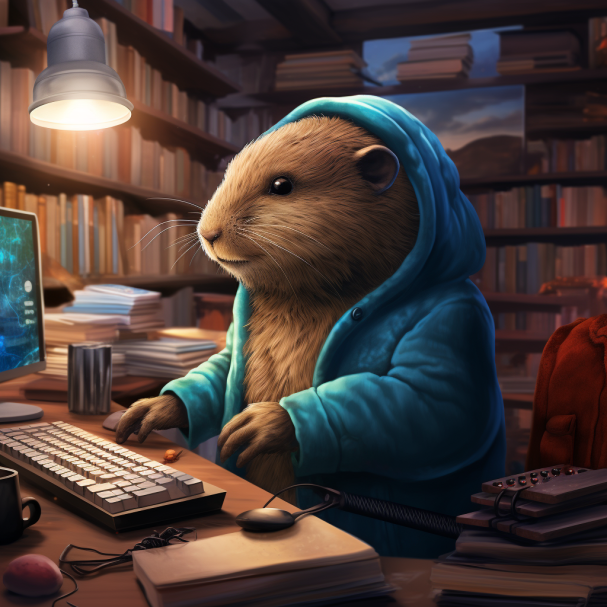
From Basics to Practical: Using Interface-Based Configuration in Go Programming
Discover how to implement the interface-based configuration pattern in Go. This guide includes a detailed code example to enhance your programming practices and streamline your configurations.
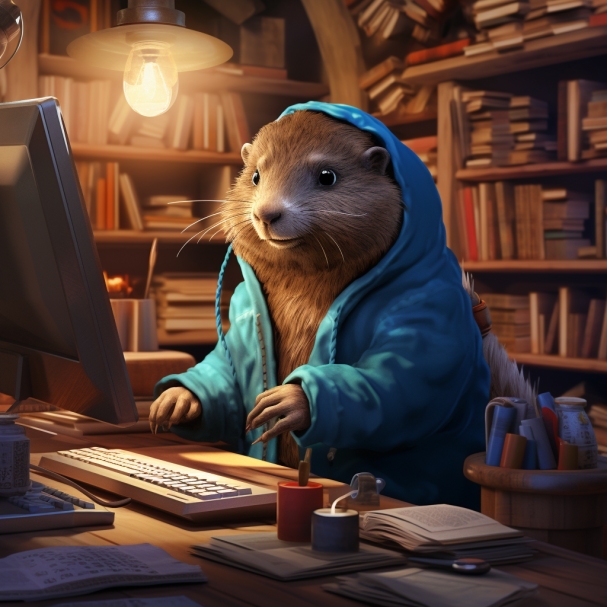
Unpacking the Functional Options Pattern in Go: Simplifying Configuration Complexity
The functional options pattern in Go provides a scalable and clean way to configure objects without overwhelming function signatures. Learn how to implement it with a practical example.

Understanding the Strangler Pattern: A Comprehensive Guide
In the ever-evolving landscape of software development, maintaining and upgrading legacy systems without disrupting existing services is a significant challenge. This is where the Strangler Pattern comes into play, offering a strategic approach to software evolution. This pattern, inspired by the strangler figs that gradually envelop and replace the trees in a forest, involves gradually refactoring and replacing parts of an old system with new functionalities, without the need to fully replace the entire system at once.

Implementing the Saga Pattern in Go: A Practical Guide
In the world of microservices, ensuring data consistency across services without resorting to traditional, heavyweight transaction mechanisms is a common challenge. Enter the Saga Pattern: a strategy designed to manage transactions and compensations across loosely coupled services.

Mastering the Result Pattern in Software Development
In the world of programming, there are countless languages to choose from, each with its unique strengths and applications. One language that has soared in popularity and established itself as a favorite among beginners and seasoned developers alike is Python. Renowned for its simplicity, versatility, and readability, Python has become a go-to choice for a wide range of projects, from web development and data analysis to artificial intelligence and automation.

Mastering the Open/Closed Principle in Go Programming
The Open/Closed Principle (OCP) is a fundamental concept in object-oriented programming, and it holds a vital place among the five SOLID principles. Initially formulated by Bertrand Meyer, this principle plays a pivotal role in enhancing the flexibility and maintainability of software applications.
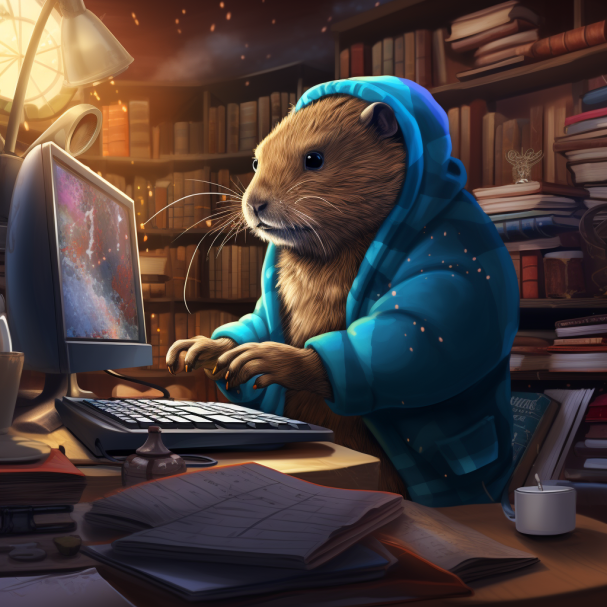
Advanced Implementation of Hexagonal Architecture in Go
In the realm of software design, Hexagonal Architecture, also known as Ports and Adapters Architecture, stands as a beacon for creating flexible, maintainable, and scalable applications. Its application in Go, a language celebrated for its straightforwardness and performance, can significantly enhance the adaptability and testability of software projects.
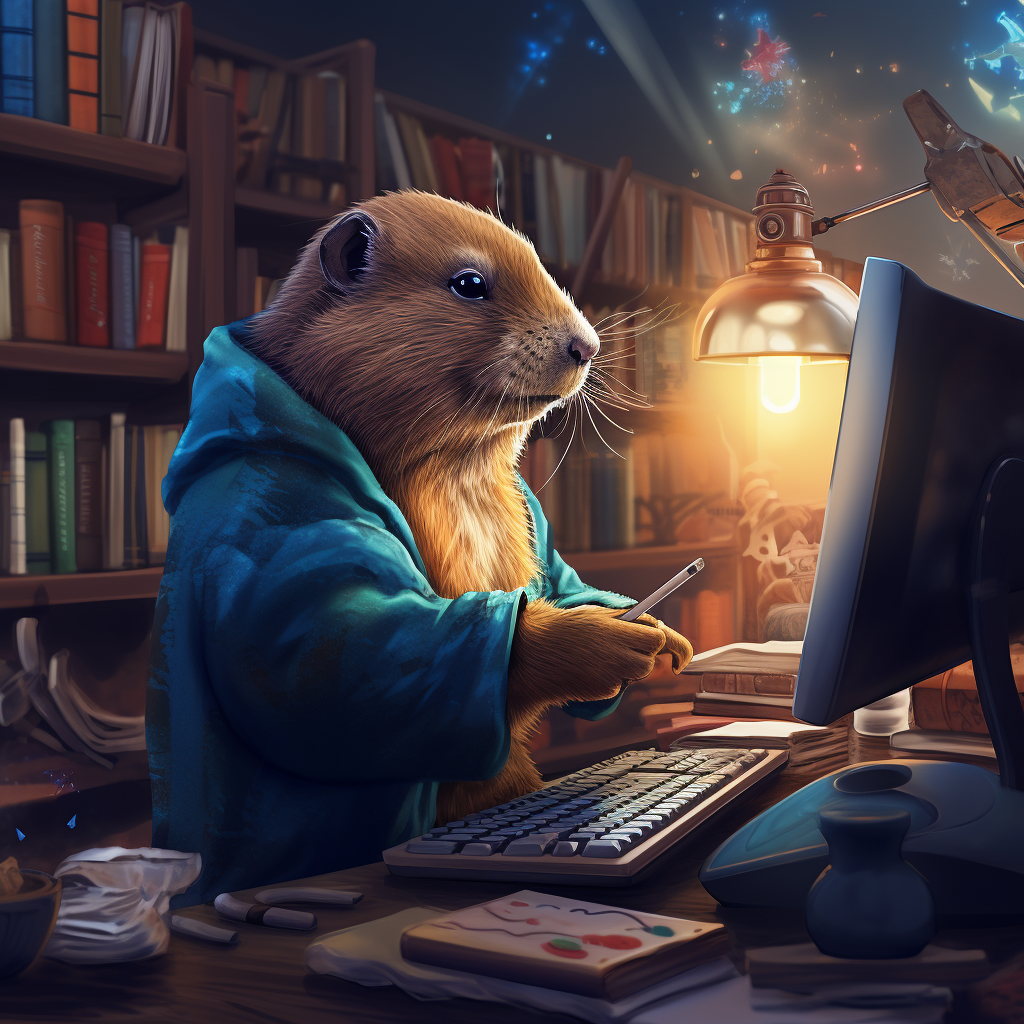
Understanding and Implementing the Semaphore Pattern in Go
In the realm of concurrent programming, managing access to shared resources is a critical aspect that can significantly impact the performance and reliability of an application. One effective technique to manage this is the Semaphore pattern.
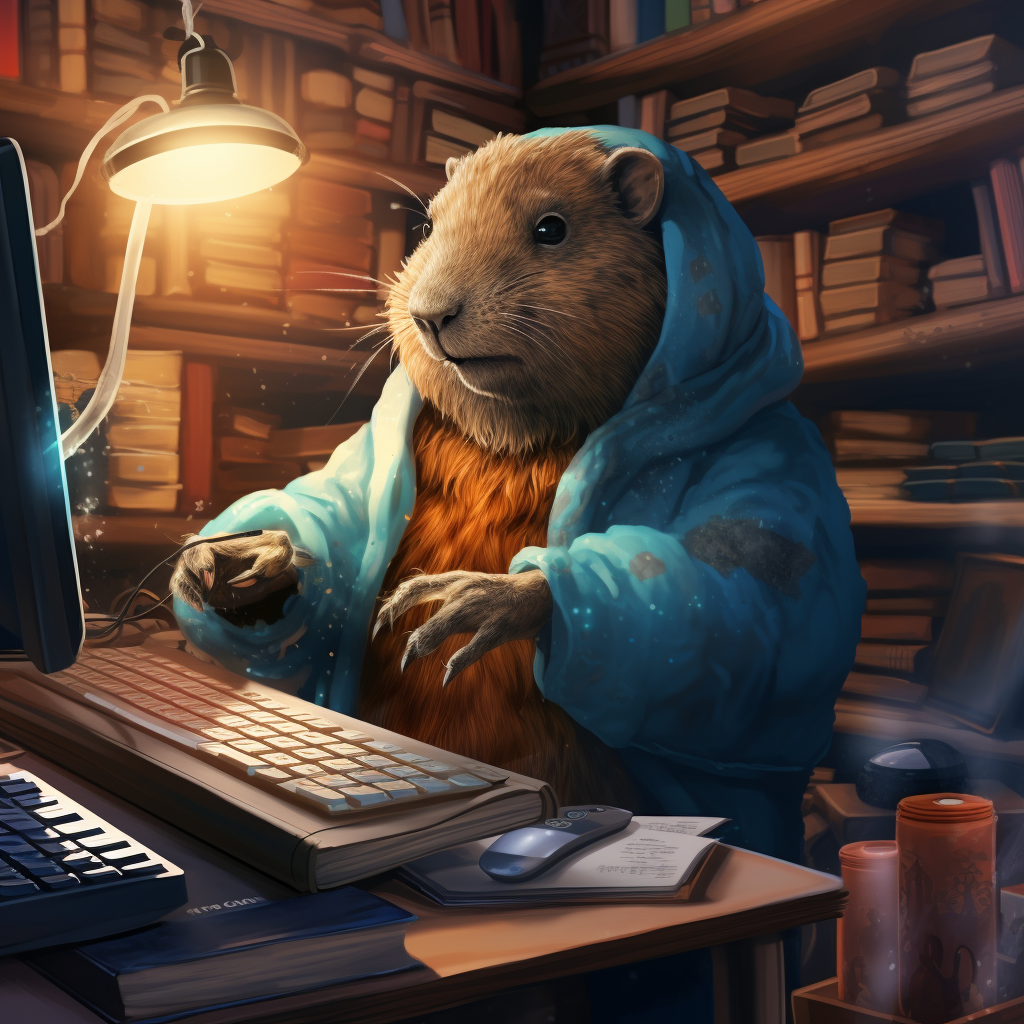
Demystifying the Extractor Pattern in Go
Go is a statically typed, compiled language that has gained immense popularity in recent years due to its simplicity, efficiency, and strong concurrency support. When it comes to designing clean and maintainable code in Go, developers often turn to design patterns to solve common problems. One such pattern is the "Extractor Pattern," which can be a powerful tool in your Go programming toolkit.

Mastering the Repository Pattern in Go: A Comprehensive Guide
The Repository pattern is a widely used design pattern in software development, especially useful when dealing with data access layers. In the world of Go, implementing the Repository pattern can significantly enhance the maintainability, testing, and modularity of your applications.

Boosting Code Modularity in Go Using Wire for Dependency Injection
Dependency injection is a fundamental concept in software engineering that promotes modularity and maintainability in your code. In the world of Go, where simplicity and efficiency are highly valued, using a tool like Wire can make dependency injection easier and more manageable.
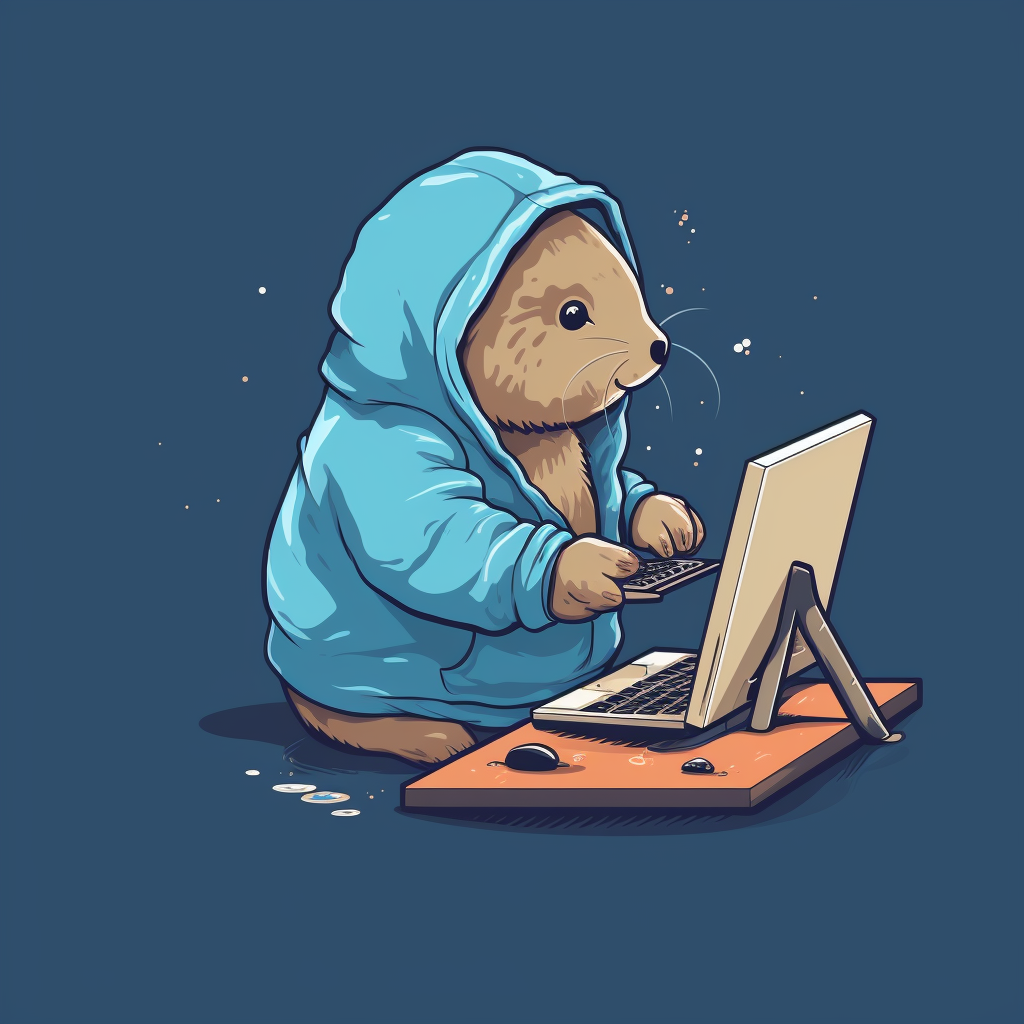
Design Pattern Series: Demystifying the Bridge Design Pattern in Go
Design patterns are essential tools in a programmer's toolbox. They provide solutions to common software design problems and promote code reusability, maintainability, and scalability. One such design pattern is the Bridge pattern, which separates an object's abstraction from its implementation.
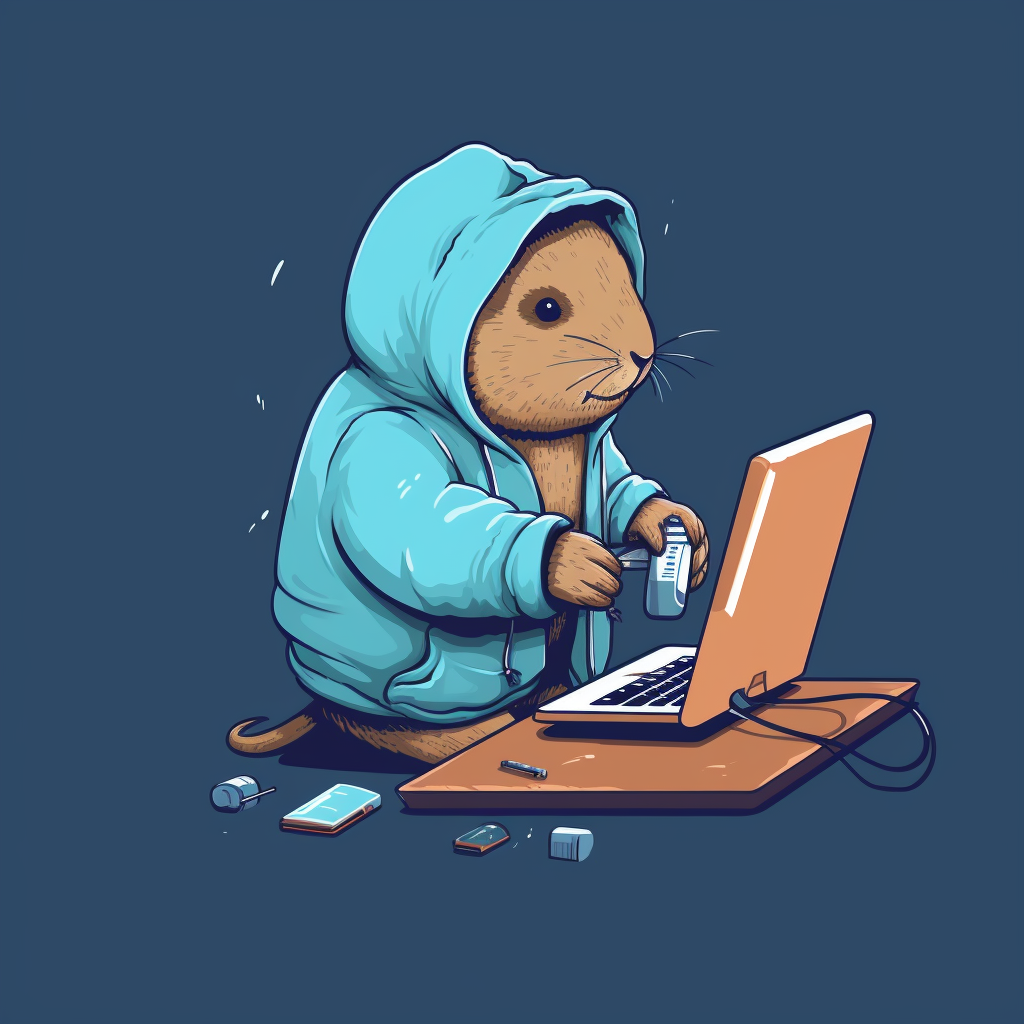
Design Pattern Series: Understanding the Facade Design Pattern in Go
Design patterns are essential tools in the software developer's toolkit, helping us solve common problems in elegant and maintainable ways. One such pattern is the Facade design pattern.
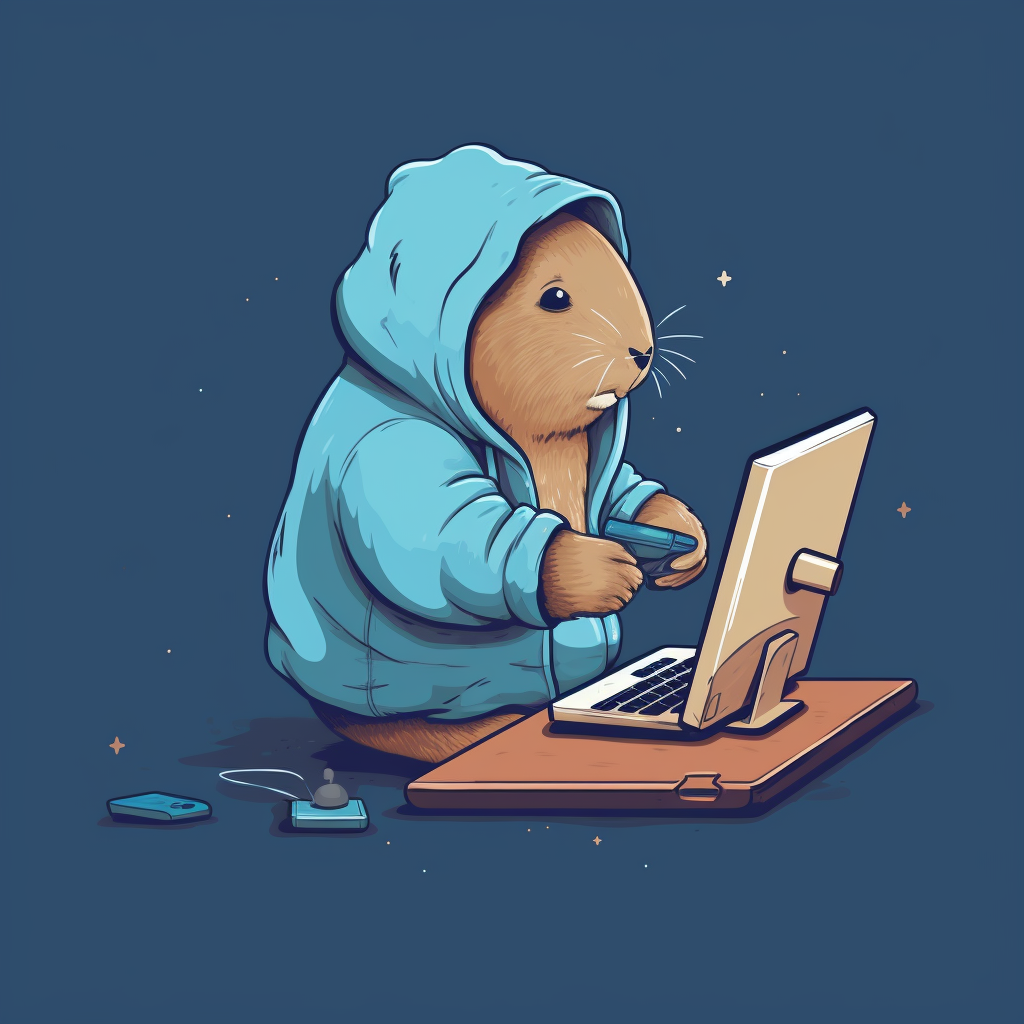
Design Pattern Series: Enhancing Functionality Elegantly with the Decorator Pattern
In the world of software design, patterns play a crucial role in solving common problems in a structured and efficient manner. Among these, the Decorator pattern stands out for its ability to add new functionality to objects dynamically. In Go, a language known for its simplicity and efficiency, implementing the Decorator pattern can significantly enhance the functionality of your code without cluttering the core logic.
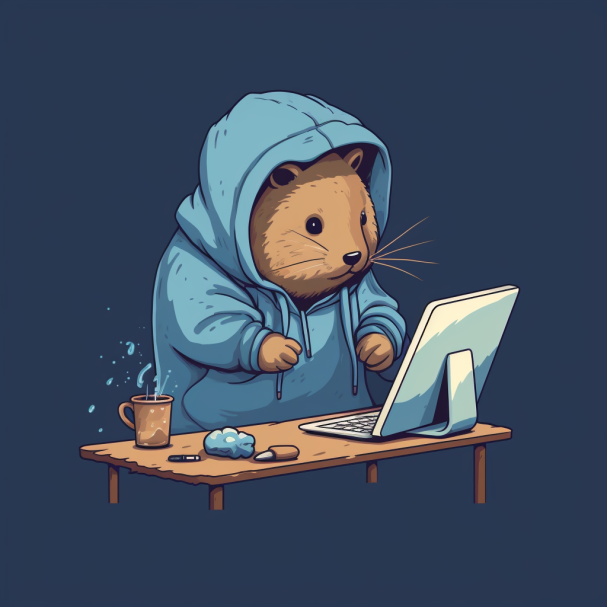
Design Pattern Series: Simplifying Complex Interfaces with the Adapter Pattern
In the world of software design, the Adapter pattern is a crucial tool for ensuring that different parts of a system can work together smoothly. This is particularly relevant in Go, a language known for its simplicity and efficiency.
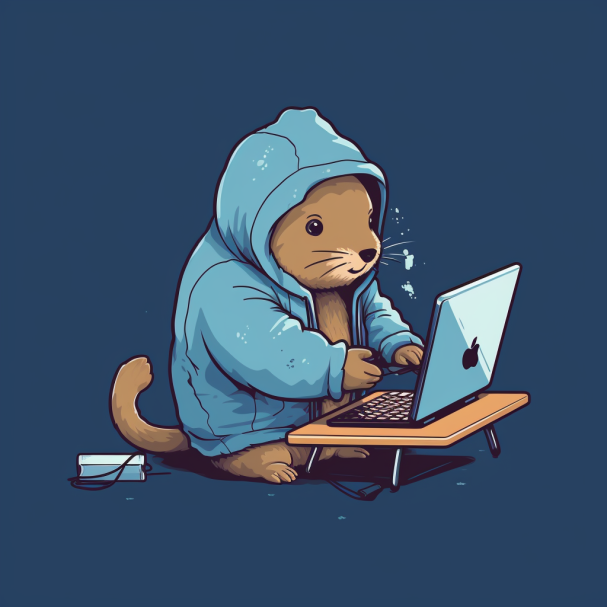
Design Pattern Series: Understanding the Abstract Factory Pattern
In the world of software design, design patterns serve as blueprints for solving common design problems. Among these, the Abstract Factory Pattern is a creational pattern used to create families of related or dependent objects without specifying their concrete classes. In Go, a language known for its simplicity and efficiency, implementing the Abstract Factory Pattern can streamline your development process, particularly when dealing with complex object creation.
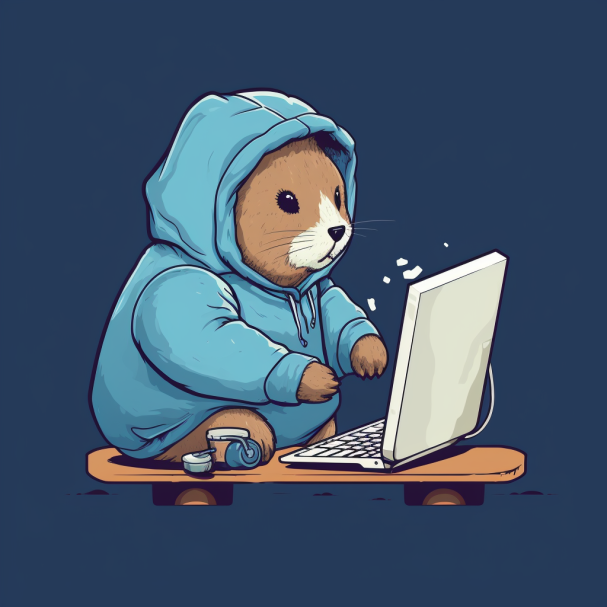
Design Pattern Series: Simplifying Object Creation with the Prototype Pattern
In the world of software design, patterns play a crucial role in solving common design problems. One such pattern, the Prototype pattern, is particularly useful in scenarios where object creation is a costly affair. This blog post delves into the Prototype pattern, its significance, and its implementation in Go, a popular statically typed, compiled programming language known for its simplicity and efficiency.