Curious About Technology
Welcome to Coding Explorations, your go-to blog for all things software engineering, DevOps, CI/CD, and technology! Whether you're an experienced developer, a curious beginner, or simply someone with a passion for the ever-evolving world of technology, this blog is your gateway to valuable insights, practical tips, and thought-provoking discussions.
Recent Posts

Mastering Nil Channels in Go: Enhance Your Concurrency Control
Using nil channels in Go can be a powerful tool for controlling the flow of concurrent operations, but it may initially seem counterintuitive. Here, we’ll explore what nil channels are, how Go treats them, and practical ways to utilize them effectively in your concurrent programming patterns.
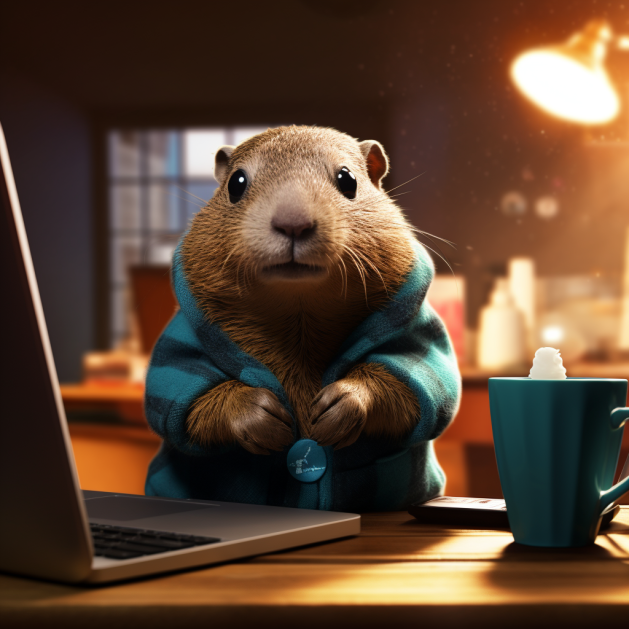
How to Effectively Unit Test Goroutines in Go: Tips & Tricks
Unit testing in Go, especially when it involves goroutines, presents unique challenges due to their concurrent nature. Goroutines are lightweight threads managed by the Go runtime, and they are used to perform tasks concurrently.

Understanding the Difference Between make and new in Go
In the Go programming language, memory allocation and initialization are crucial concepts that allow developers to manage data structures efficiently. Two built-in functions that play a significant role in this context are make and new. Although they might seem similar at first glance, they serve different purposes and are used in different scenarios.
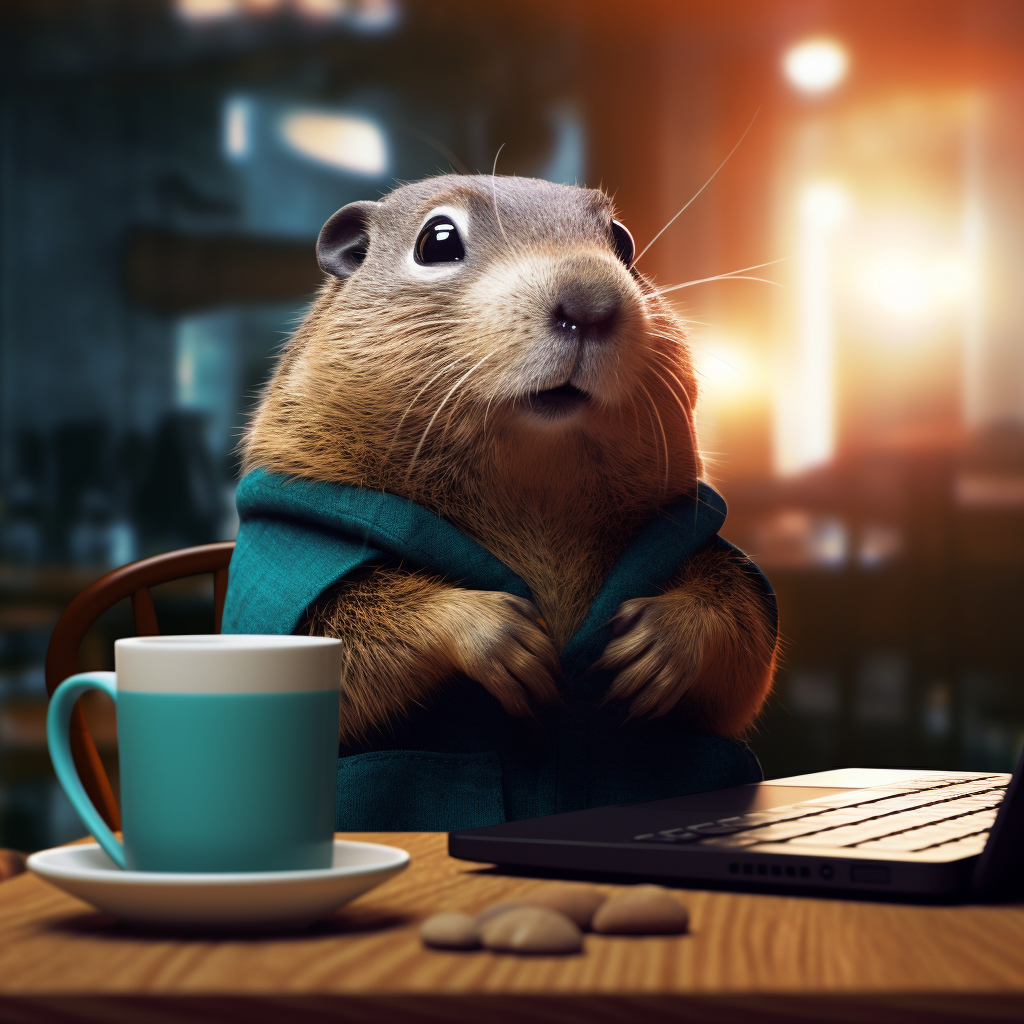
Understanding Type Aliases in Go: A Comprehensive Guide
Go is a statically typed programming language that has gained popularity for its simplicity, efficiency, and strong support for concurrent programming. One of the features that contribute to its simplicity and flexibility is the concept of type aliases.
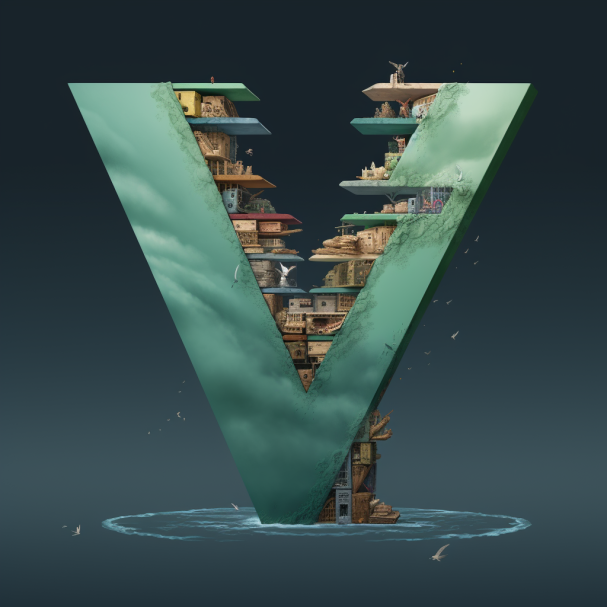
Vue 3 Slots Explained: Enhancing Component Flexibility
Vue 3 slots are a powerful feature that allow for more flexible and reusable components. Slots enable component templates to include "placeholders" that can be filled with custom content when the component is used, rather than having a fixed content structure. This makes slots particularly useful for creating a library of components that can be customized as needed.

Understanding Variable Passing into Go Routines
Go is a statically typed, compiled programming language designed for simplicity and efficiency, with a particular emphasis on concurrent programming. One of the core features of Go's concurrency model is goroutines, which are functions capable of running concurrently with other functions. A common question among Go developers, especially those new to the language, is why and how to pass variables into a goroutine.
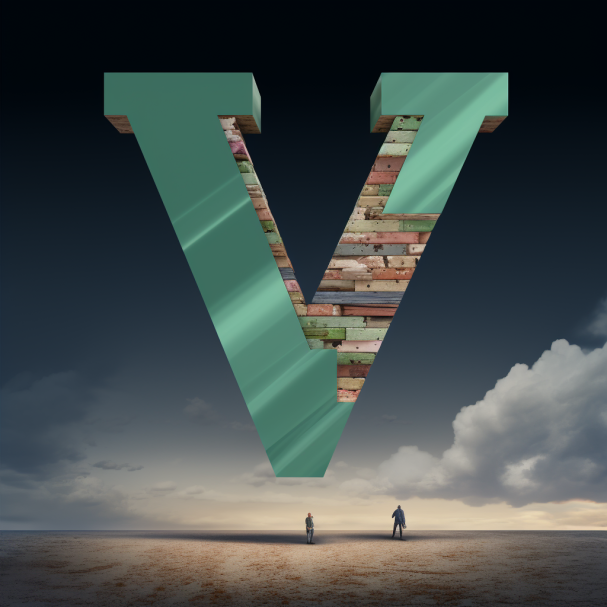
Mastering Vue 3: Harnessing nextTick for Seamless DOM Updates
Vue 3, the progressive JavaScript framework for building user interfaces, has introduced a variety of features and improvements over its predecessor. Among these enhancements, the Composition API, better TypeScript integration, and performance optimizations stand out. An important yet often overlooked tool in Vue 3's arsenal is the nextTick function.
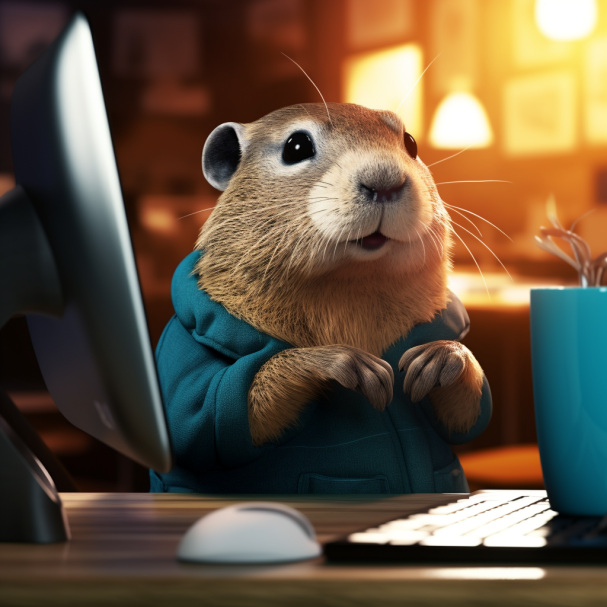
Using Go for Data Science: A Fresh Perspective
In the ever-evolving landscape of data science, Python has long been the reigning champion, largely due to its simplicity and the vast array of libraries available. However, the tide is slowly turning, and other languages are making their mark in the data science realm. One such contender is Go. Go is gaining popularity for its efficiency, performance, and ease of use.
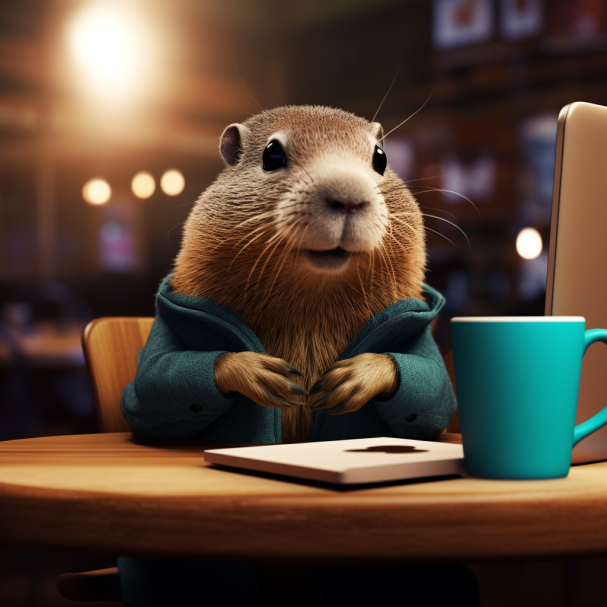
Understanding Go's Garbage Collection: A Deep Dive
Go is a statically typed, compiled programming language. Among its many features, Go's garbage collection mechanism stands out as a critical component for memory management.
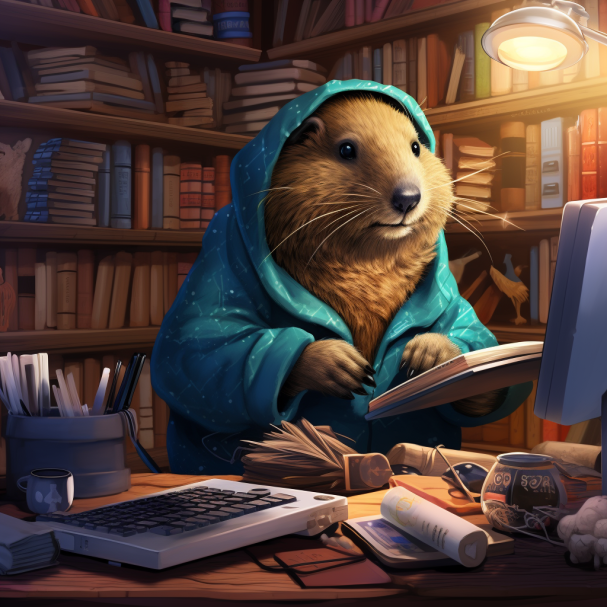
Efficient Data Management in Go: The Power of Struct Tags
In the world of programming, efficiency and organization are paramount. Go, a statically typed language developed by Google, provides a unique feature for structuring and managing data effectively: struct tags. These tags offer an elegant way to add metadata to the structure fields, making data serialization and validation more streamlined and robust.
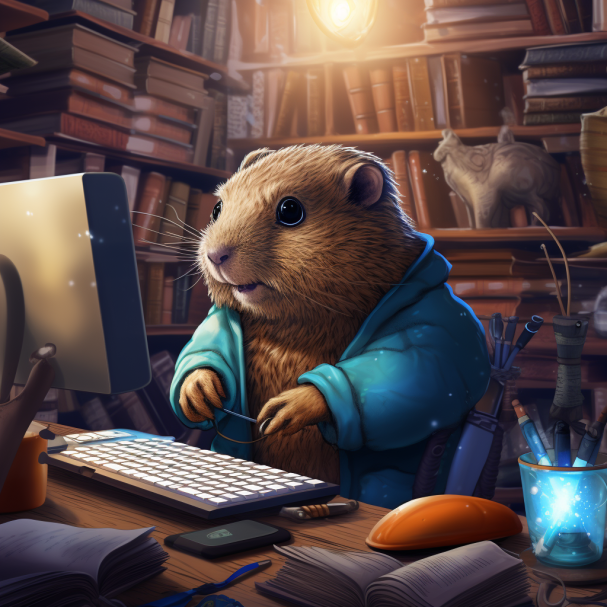
Mastering SQLMock for Effective Database Testing
Testing is a crucial aspect of software development, ensuring that applications perform as expected. For applications that interact with databases, mocking the database interactions is essential for isolated and efficient testing. SQLMock is a powerful tool in the Go ecosystem, designed to facilitate testing of database interactions without relying on a real database.
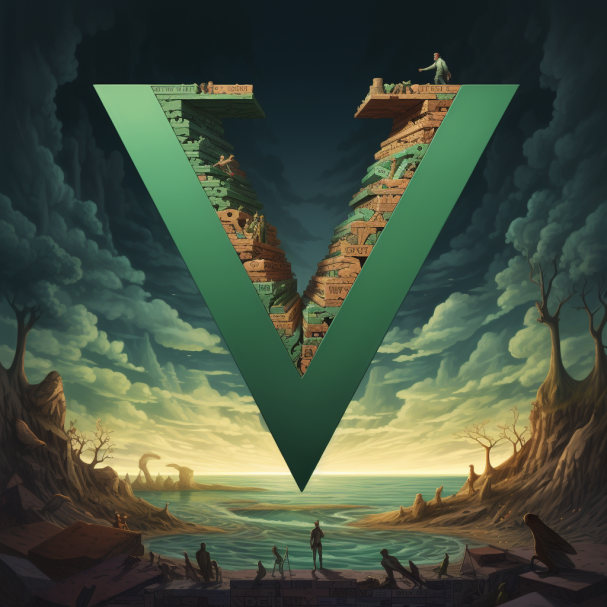
Understanding and Using "ref" in Vue 3
Vue 3, the latest iteration of the popular JavaScript framework, brings a multitude of features and improvements that enhance the development experience. Among these, the ref function stands out as a vital tool for Vue developers.
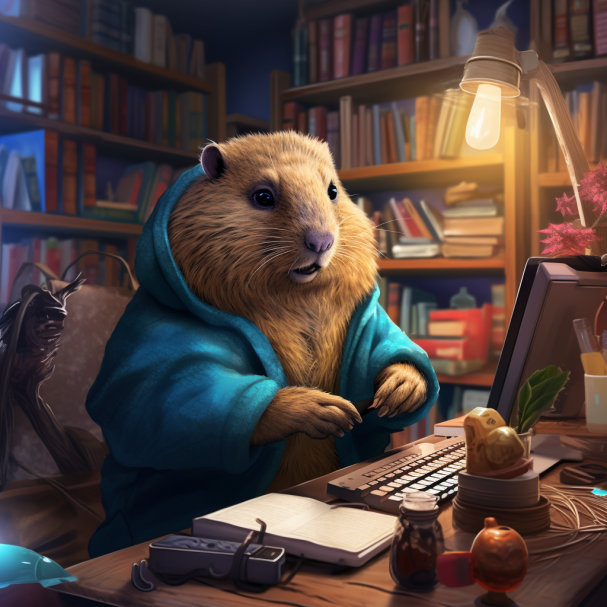
Mastering Performance Testing in Go with Vegeta
In the realm of software development, particularly in an era where web services and APIs reign supreme, ensuring that your application can handle high traffic and deliver consistent performance is paramount. For developers working in Go, a language renowned for its efficiency and concurrency capabilities, Vegeta emerges as a powerful tool for performance testing.

Mastering the Result Pattern in Software Development
In the world of programming, there are countless languages to choose from, each with its unique strengths and applications. One language that has soared in popularity and established itself as a favorite among beginners and seasoned developers alike is Python. Renowned for its simplicity, versatility, and readability, Python has become a go-to choice for a wide range of projects, from web development and data analysis to artificial intelligence and automation.
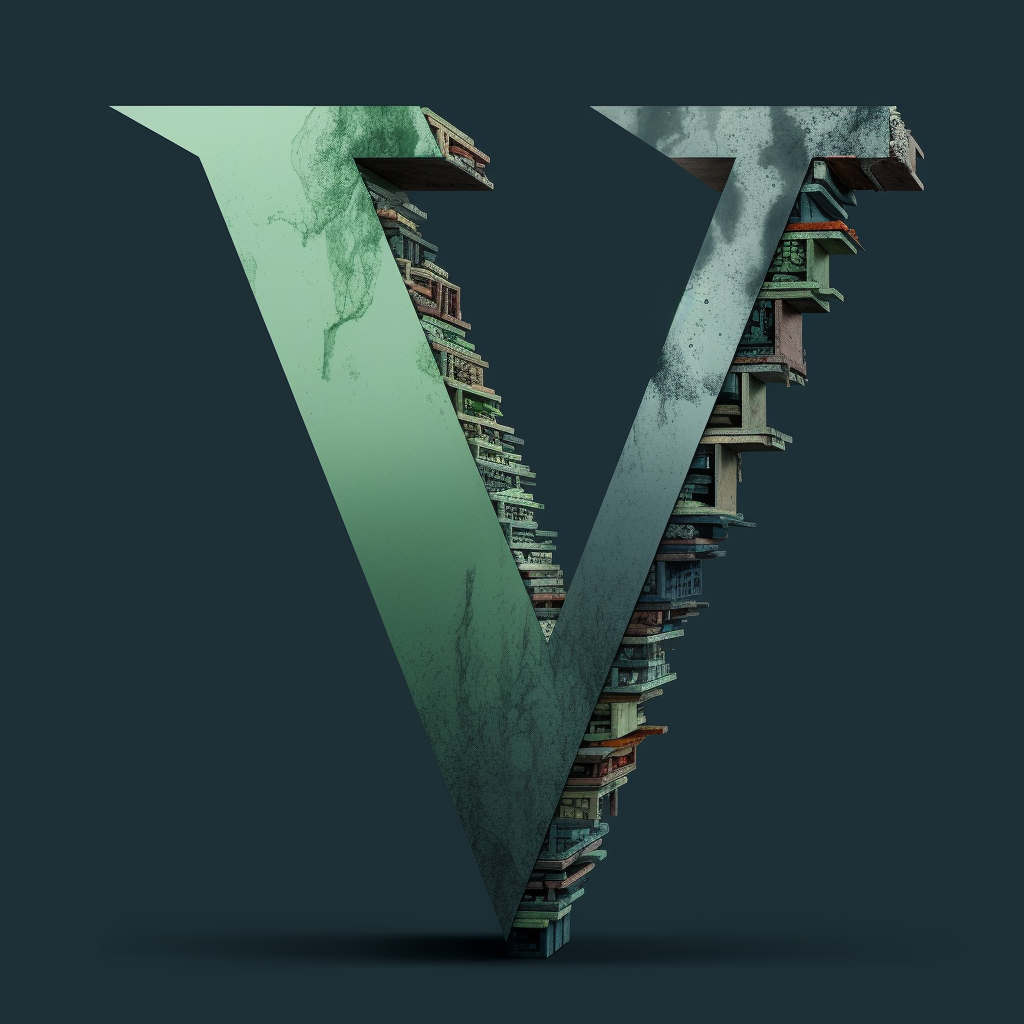
Mastering Two-Way Binding in Vue 3: A Comprehensive Guide
Vue.js, a progressive JavaScript framework, has been a game-changer for developers looking to build dynamic and interactive web applications. One of its core features, the two-way data binding, has significantly streamlined the way developers handle form inputs and user interactions. With the release of Vue 3, there are more efficient and versatile ways to implement this feature.

Mastering the Open/Closed Principle in Go Programming
The Open/Closed Principle (OCP) is a fundamental concept in object-oriented programming, and it holds a vital place among the five SOLID principles. Initially formulated by Bertrand Meyer, this principle plays a pivotal role in enhancing the flexibility and maintainability of software applications.
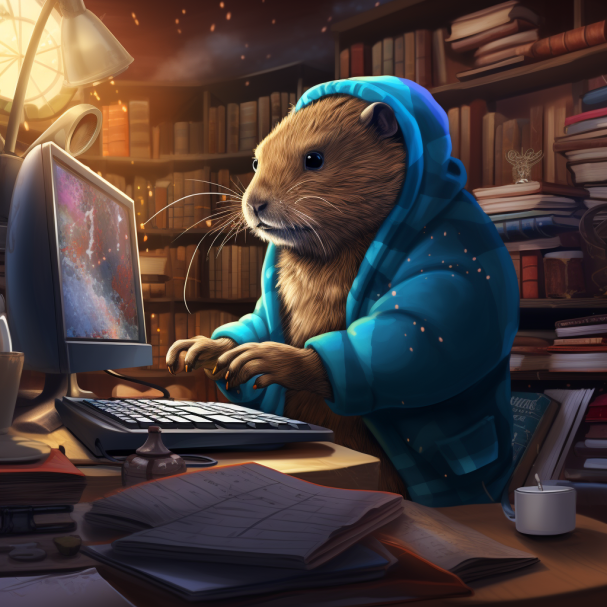
Advanced Implementation of Hexagonal Architecture in Go
In the realm of software design, Hexagonal Architecture, also known as Ports and Adapters Architecture, stands as a beacon for creating flexible, maintainable, and scalable applications. Its application in Go, a language celebrated for its straightforwardness and performance, can significantly enhance the adaptability and testability of software projects.
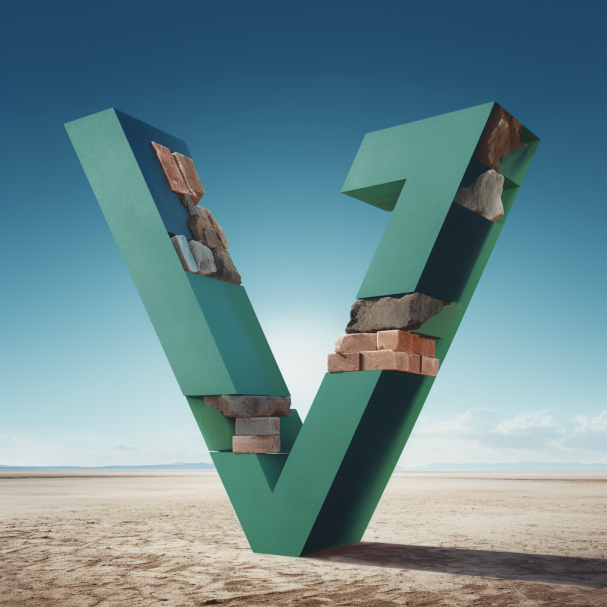
Mastering Custom Directives in Vue 3
Vue 3, with its improved performance and powerful Composition API, has brought significant advancements to the Vue.js ecosystem. Among these improvements, custom directives continue to be a crucial feature, offering developers a way to directly interact with the DOM.
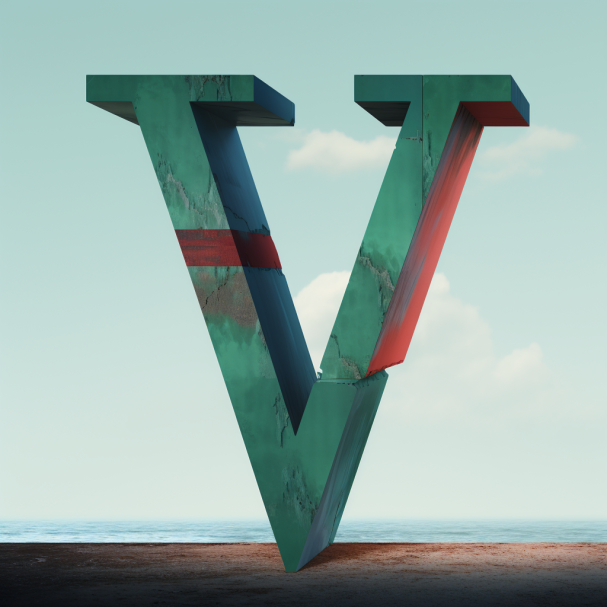
Understanding and Implementing Teleport in Vue.js
Vue.js, a progressive JavaScript framework, is known for its flexibility and ease of integration. One of the intriguing features introduced in Vue 3 is the Teleport component. This feature is a game-changer for handling modals, notifications, and more. It allows developers to control where components are rendered in the DOM, independent of where they are declared in the Vue component tree. Let's dive into the concept of Teleport and see it in action with code examples.
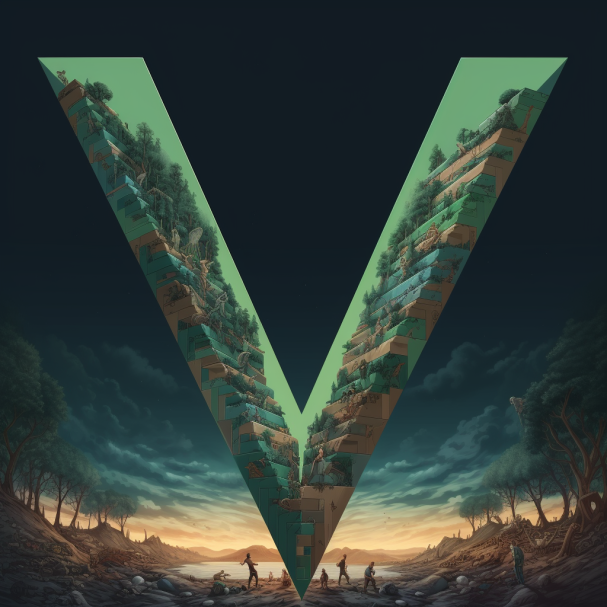
Understanding the Differences: Vue's Options API vs Composition API
Vue.js, a popular JavaScript framework for building user interfaces, offers two different APIs for composing components: the Options API and the Composition API. Both have their unique features and use cases, and understanding their differences is key to writing efficient and manageable Vue applications.