Curious About Technology
Welcome to Coding Explorations, your go-to blog for all things software engineering, DevOps, CI/CD, and technology! Whether you're an experienced developer, a curious beginner, or simply someone with a passion for the ever-evolving world of technology, this blog is your gateway to valuable insights, practical tips, and thought-provoking discussions.
Recent Posts
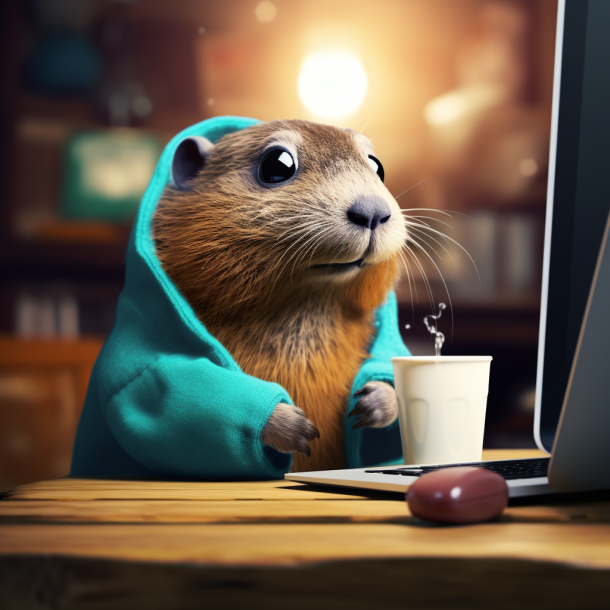
Understanding Empty Interfaces in Go
In many programming languages, interfaces are used to define a contract that types must fulfill. Go, commonly known as Golang, is no different. One unique and powerful feature in Go’s type system is the empty interface. For those coming from dynamically-typed languages or even some statically-typed languages, this can be a curious concept.
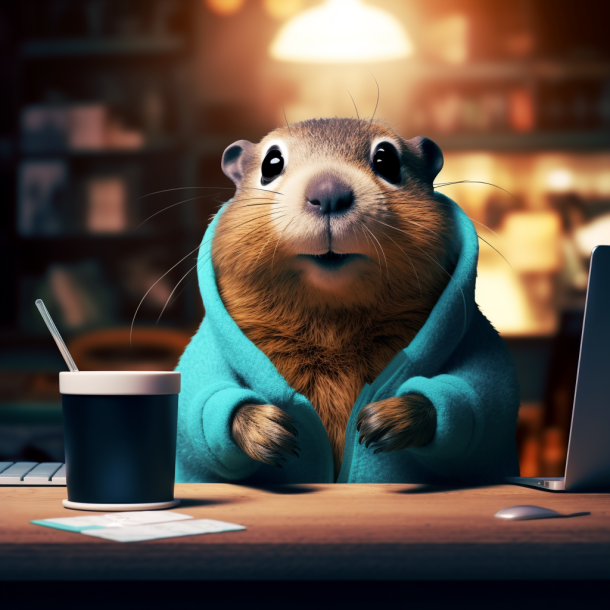
Understanding Golang's Atomic Package and Mutexes
Go is a modern programming language designed to simplify the development of scalable and concurrent software. Two of its major concurrency primitives are the atomic package and mutexes.

Manual Memory Management Techniques using unsafe in Go
Go is renowned for its simple and elegant design, particularly when it comes to memory management. The built-in garbage collector alleviates much of the manual memory management burdens found in languages like C and C++. However, there are times when developers might want to engage in manual memory management to extract more performance or for specific use-cases. This is where the unsafe package comes into play.
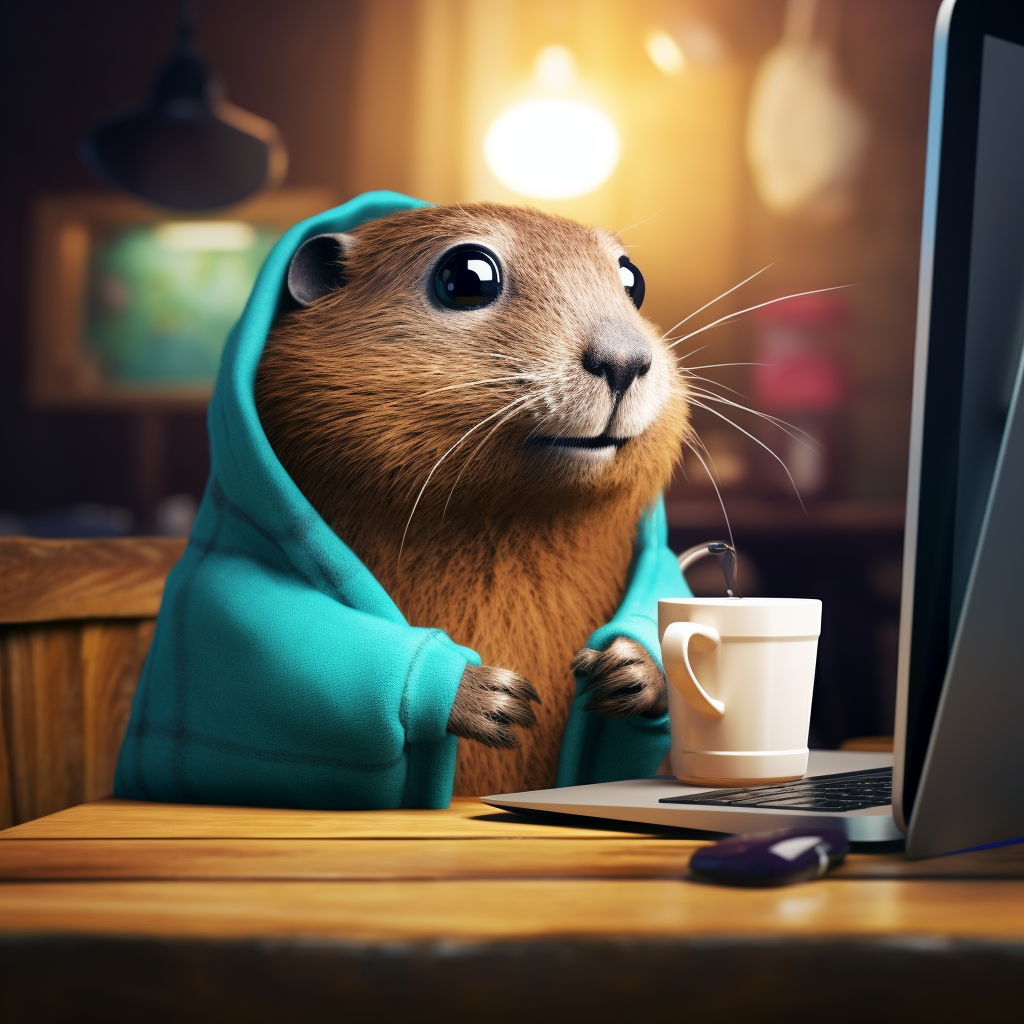
Exploring the Power of the "container" Package in Go
The Go programming language has gained significant popularity in recent years due to its simplicity, efficiency, and robust standard library. One lesser-known gem in Go's standard library is the "container" package. This package provides a set of useful data structures that can simplify and optimize various programming tasks.
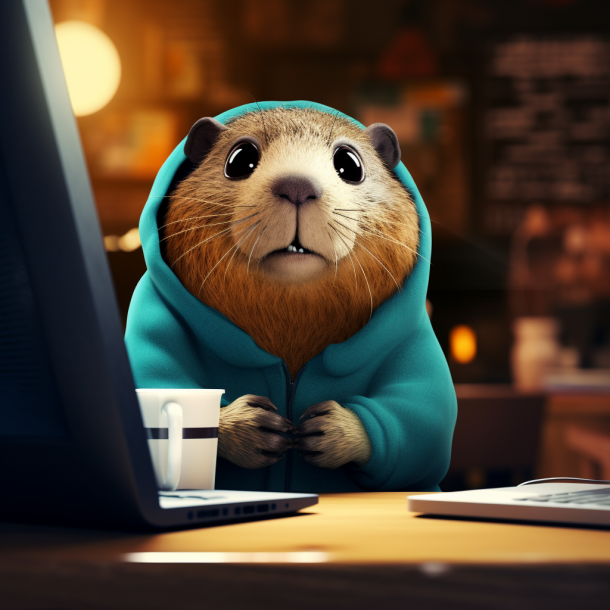
Understanding the make() Function in Go
Today, we'll delve into the intriguing world of Go's memory allocation and initialization with a focus on the make() function. Whether you're a beginner or just brushing up on Go's nuances, this post will address a few key questions around this essential function.

Calling C Functions from Go: A Quick Guide
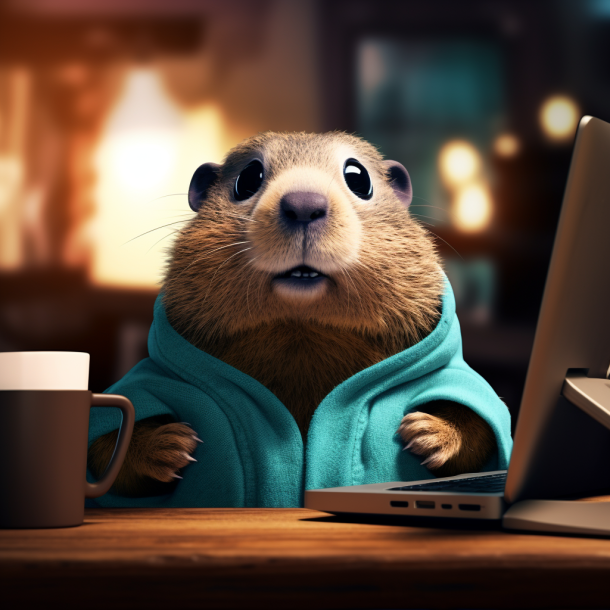
`defer` in Go: More Than Just Delayed Execution
Go is renowned for its simplicity and ease of understanding. One such feature that stands out in Go's toolbox is the defer statement. At a glance, it's a tool to postpone the execution of a function until the surrounding function returns. However, when used creatively, it can be much more.

Go Concurrency Patterns: Diving into Fan-in and Fan-out
One of the primary reasons developers love Go is its built-in concurrency support. When discussing concurrency in Go, two core patterns emerge: Fan-in and Fan-out. These concepts help in building scalable and efficient systems. Let's dive into what these patterns mean and how they're implemented in Go.
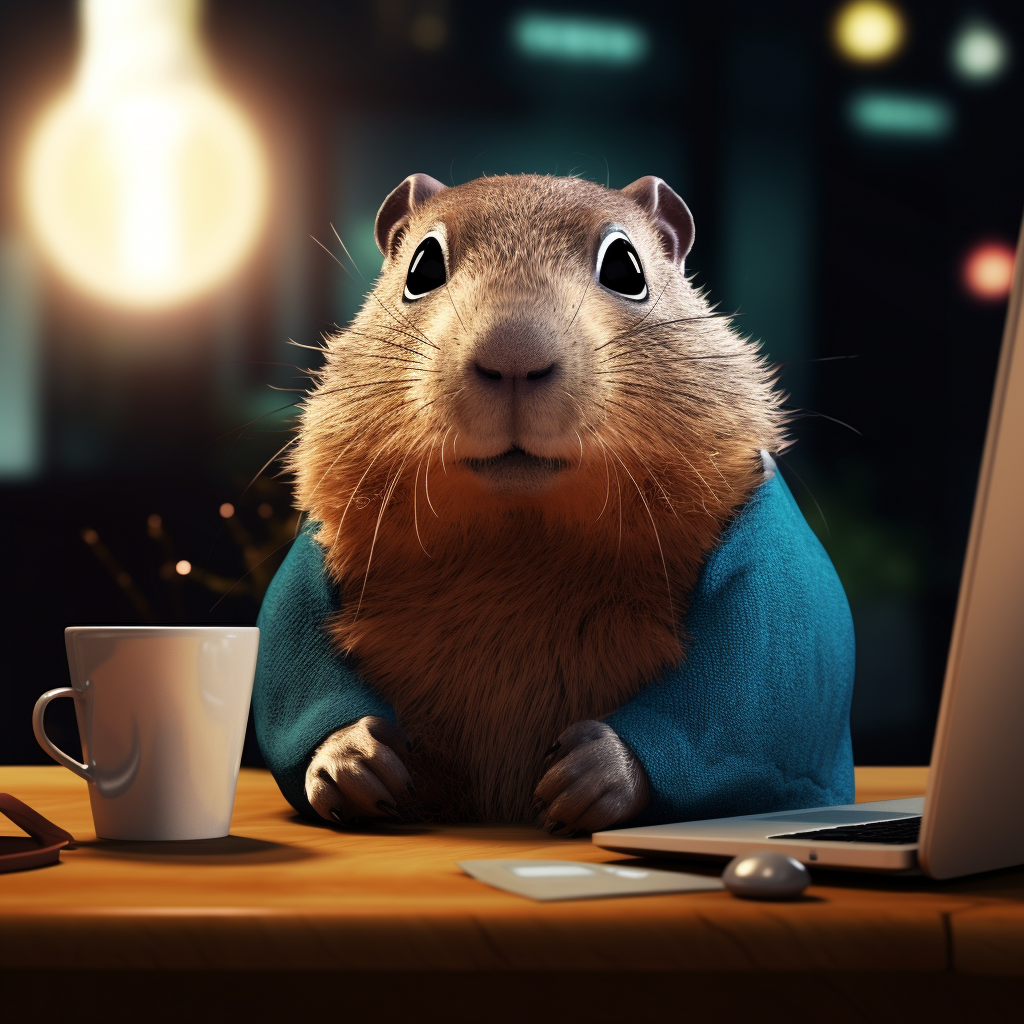
Golang Slice Iteration Techniques: From Basic to Advanced
When working with Go, you'll frequently encounter the need to loop over an array or a slice. An array is a fixed-size collection of elements of the same type, while a slice is a dynamically-sized segment of an array.

Taming Errors in Go with Panic and Recover
In the world of software, errors are inevitable. In Go (or Golang), handling errors gracefully is fundamental to building resilient applications. One unique aspect of error handling in Go is the use of panic and recover. Understanding these mechanisms will give you a deeper insight into Go's philosophy and provide you with tools to write more robust code.

Understanding Function Variables in Go
In many programming languages, functions are first-class citizens. This means that they can be passed around just like any other value. Go, also known as Golang, is no exception. In Go, we can assign a function to a variable, pass it as an argument, or even return it from another function. This provides immense power to developers, enabling patterns like callbacks, higher-order functions, and more.
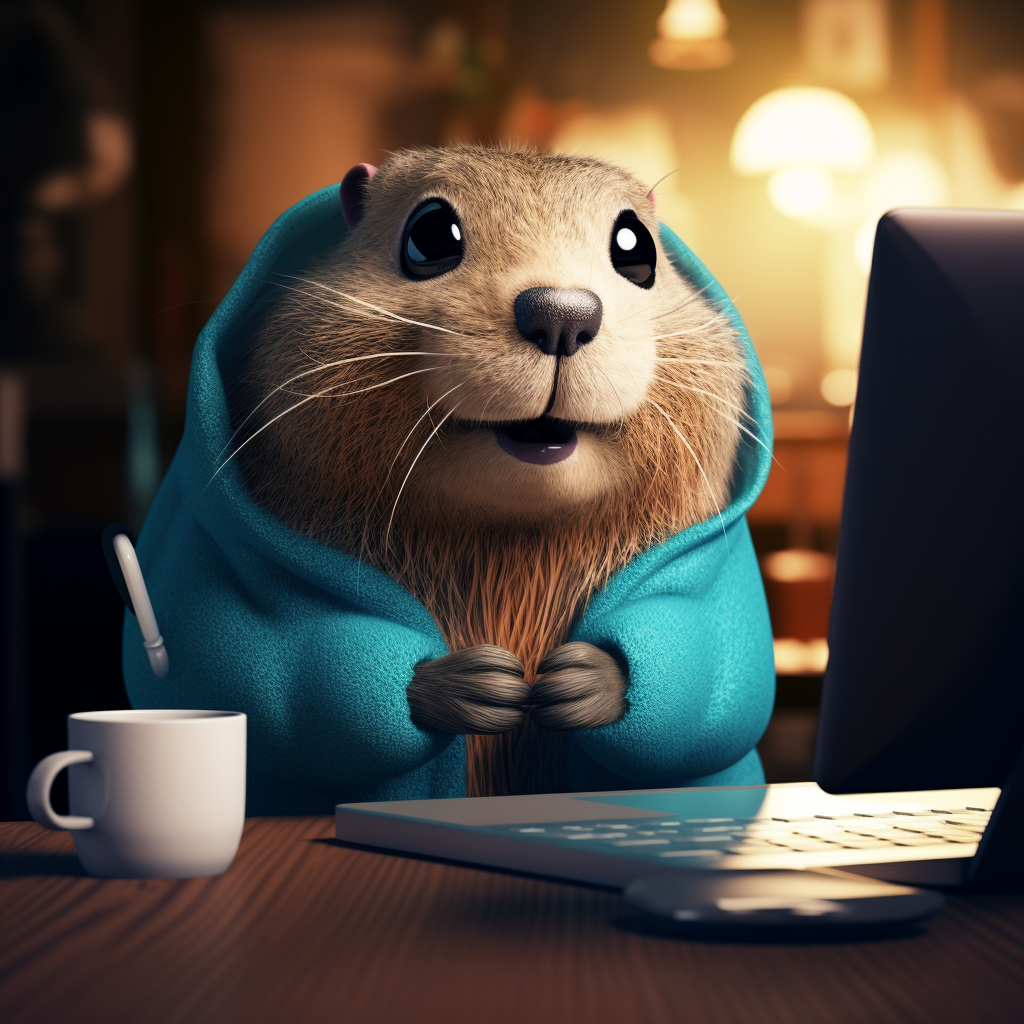
Golang Closures: From Mystery to Proficiency
The Go programming language has surged in popularity since its inception due to its simplicity, concurrency support, and strong standard library. Among its features, one that often intrigues new developers is the concept of closures. Let's take a deep dive into what closures are in Go and why they're so powerful.
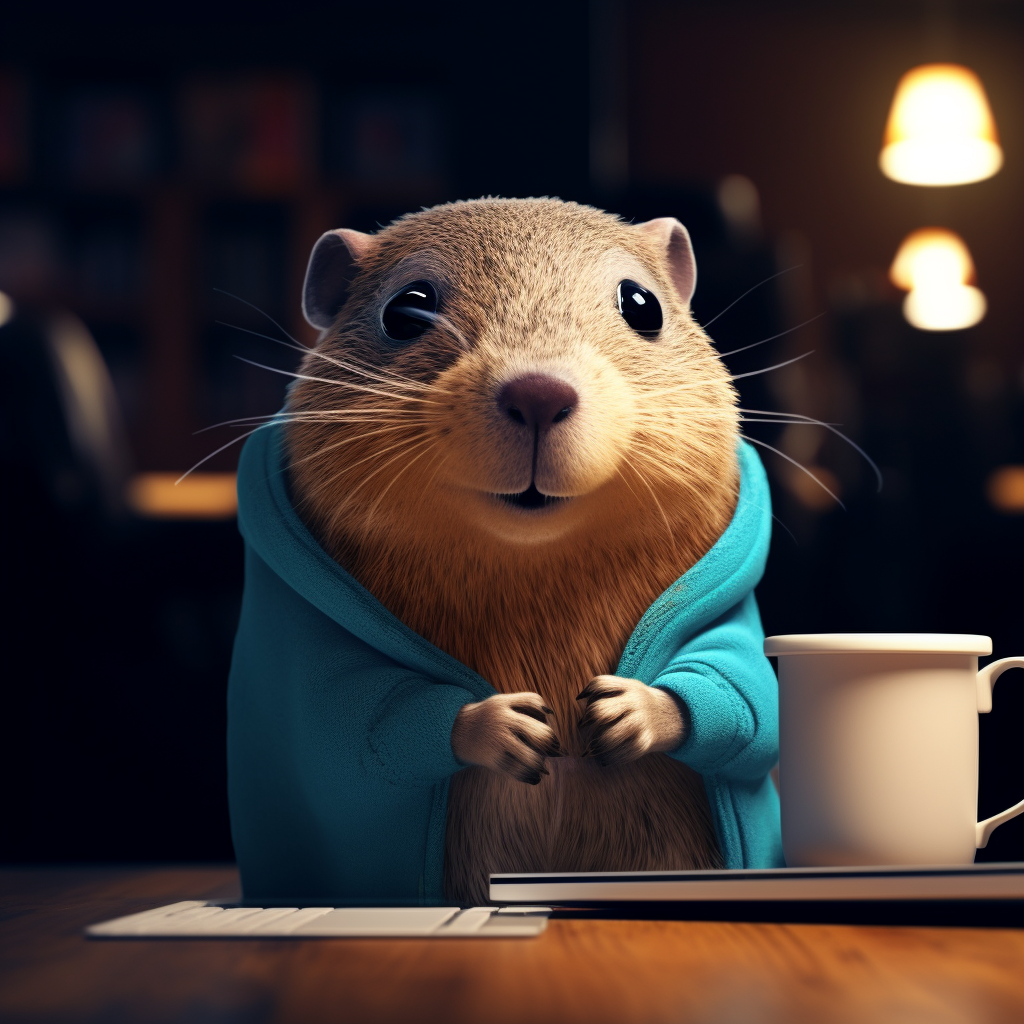
Testing GORM with SQLMock
GORM is one of the most popular ORM (Object-Relational Mapper) libraries in Go, providing a simplified and consistent way to interact with databases. As with all application code, it's imperative to ensure that your database operations are thoroughly tested. However, hitting a real database during unit tests isn't ideal. This is where SQLMock comes in. SQLMock provides a way to mock out your SQL database so you can test your GORM code without needing a live database.
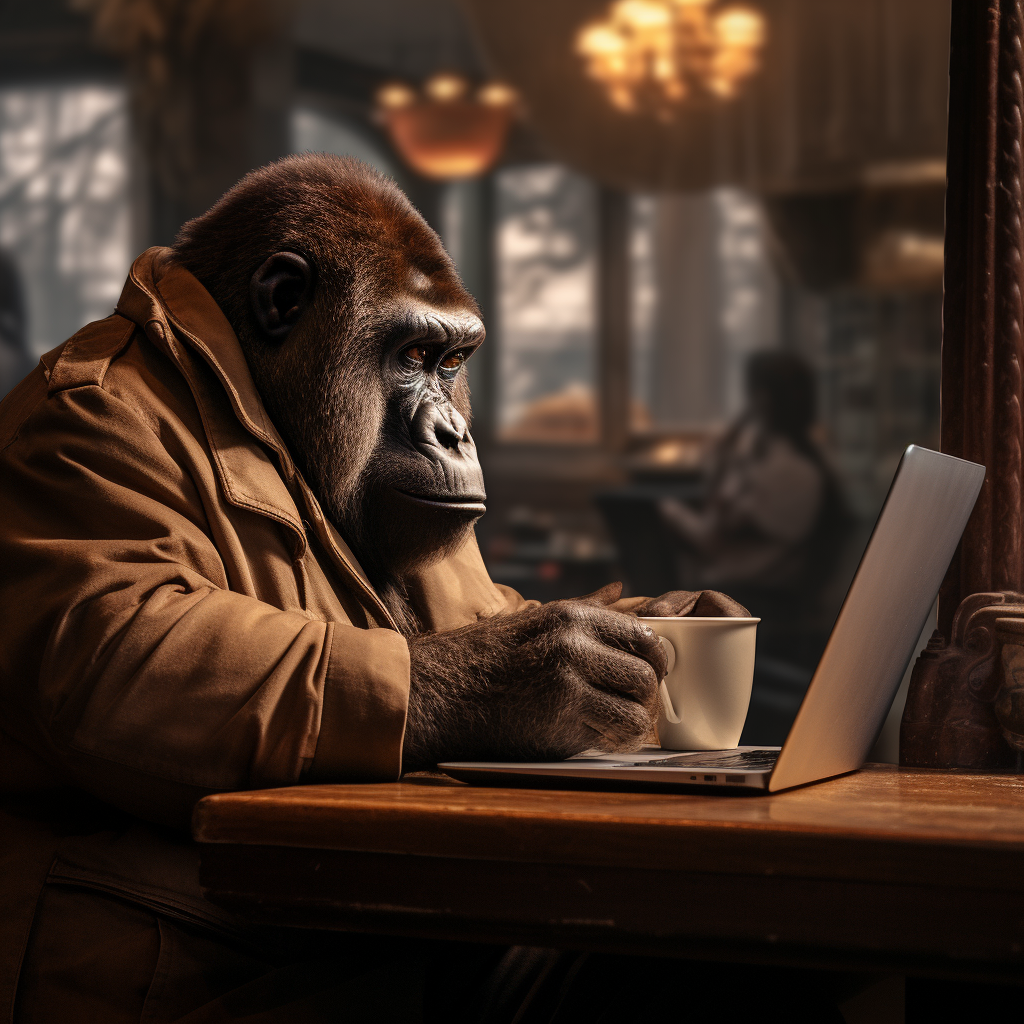
Package Design: Crafting Clear and Encapsulated Code
Software development isn't just about writing functional code; it's about structuring that code in a manner that promotes maintainability, scalability, and comprehension. One of the pivotal methods to achieve this structure is through effective package design. The art of packaging can be likened to the compartments in a well-organized closet. You wouldn't want to jumble up your winter clothes with summer wear, would you? Similarly, in software, coherent organization is the key to sanity and efficiency.

Defensive Programming in Go: The Power of defer and Nil Checks
In the vast ecosystem of software development, safety and robustness are two of the primary goals every developer should aspire to achieve. As our software becomes an integral part of modern infrastructure, the margin for error narrows. Ensuring that our software behaves predictably even under unexpected conditions is crucial. In this blog post, we’ll delve into two core techniques that bolster safety in the Go programming language: using the defer statement to ensure resources are cleaned up and guarding against nil pointer dereferences.
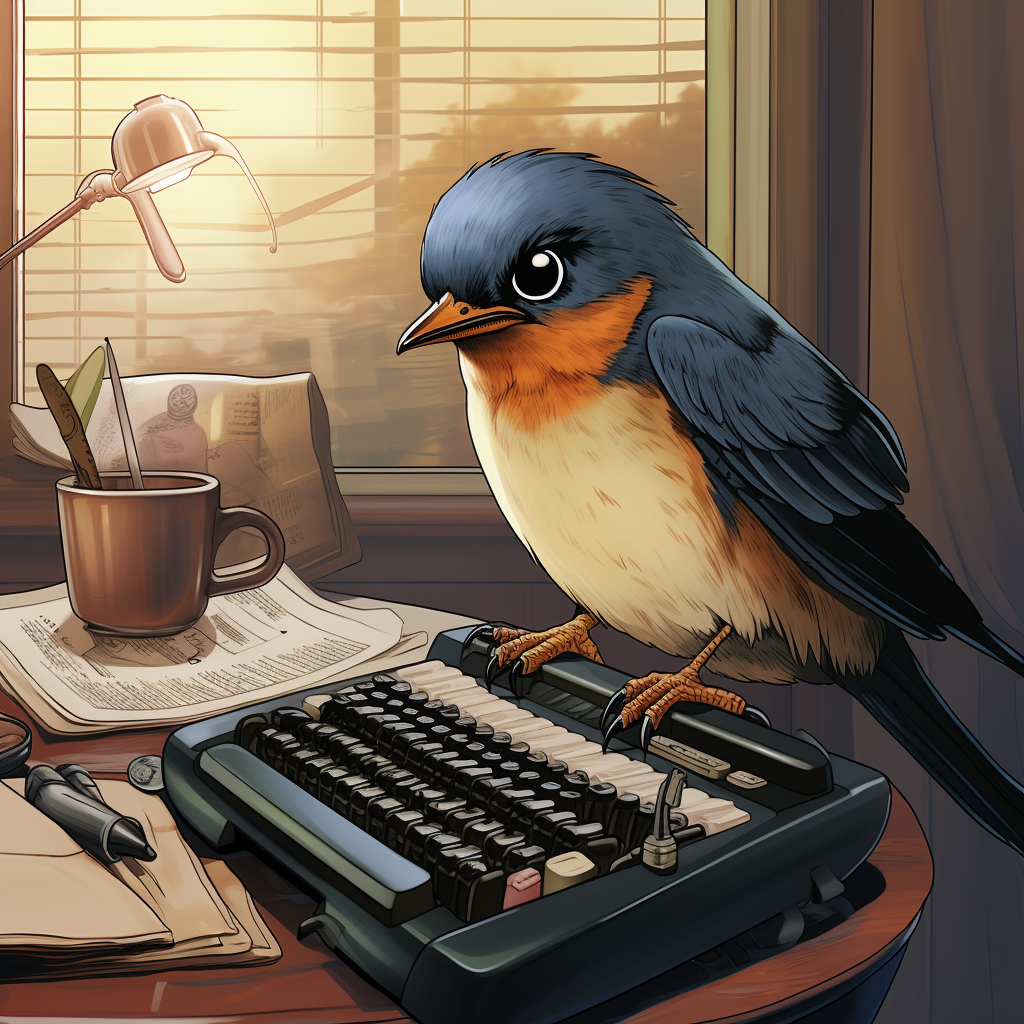
Object-Oriented Programming in Swift
Object-Oriented Programming (OOP) is a programming paradigm that relies on the concept of “objects” to design and organize code. Swift, the language developed by Apple for iOS, macOS, and other platforms, fully supports OOP concepts.

A Closer Look at Python Collections: Making the Most of namedtuple, deque, and Counter
Python, a versatile and widely used programming language, boasts a rich standard library that caters to a plethora of use cases. One such module that stands out for its utility is the collections module. This module provides alternatives to built-in types that offer additional functionality and performance benefits.
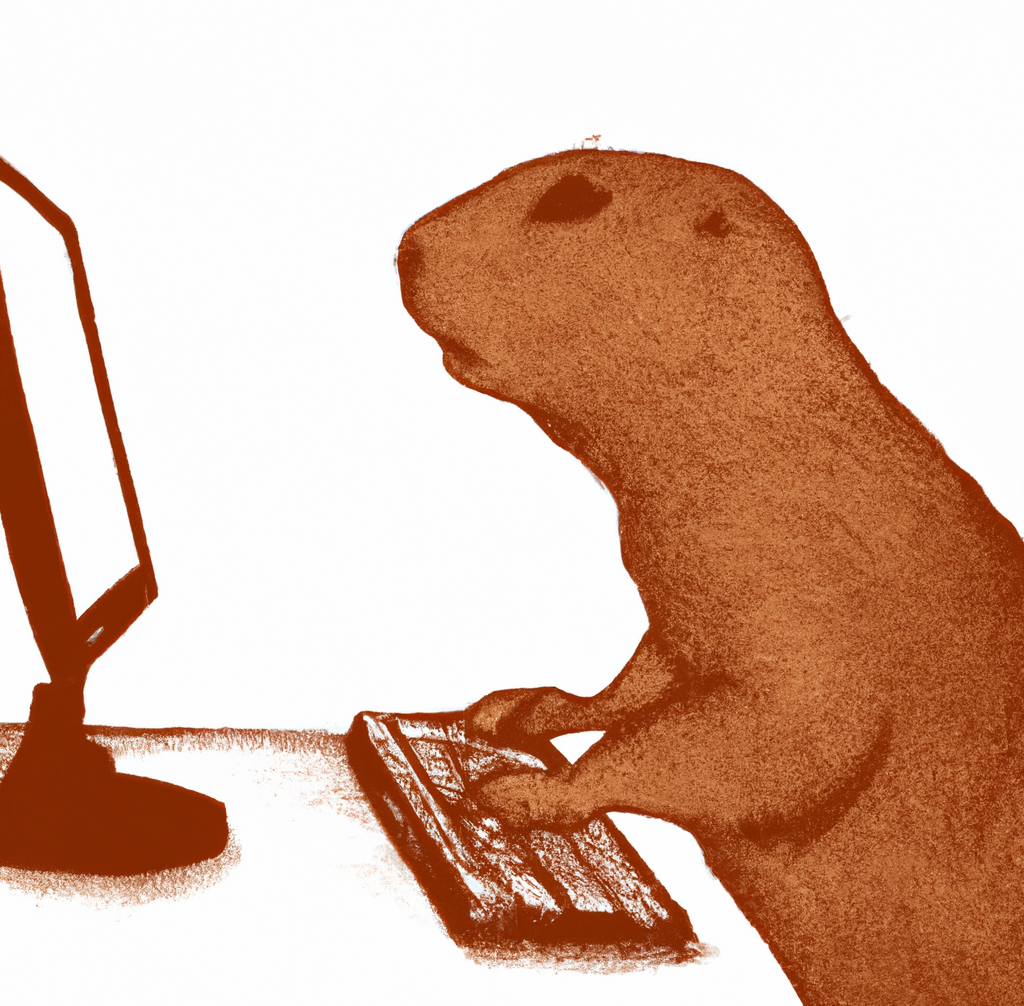
Graceful Shutdown in Go: Safeguarding Containerized Applications
In today's container-driven world, applications must be prepared to handle interruptions and terminations with grace and elegance. Especially in orchestrated environments like Kubernetes, an application could be rescheduled, moved, or terminated due to a myriad of reasons. For developers, this emphasizes the importance of ensuring their application can handle shutdown signals gracefully. Go (or Golang), with its lightweight concurrency model and robust standard library, offers tools to achieve a graceful shutdown. Let's delve into how we can implement this in a Go application.
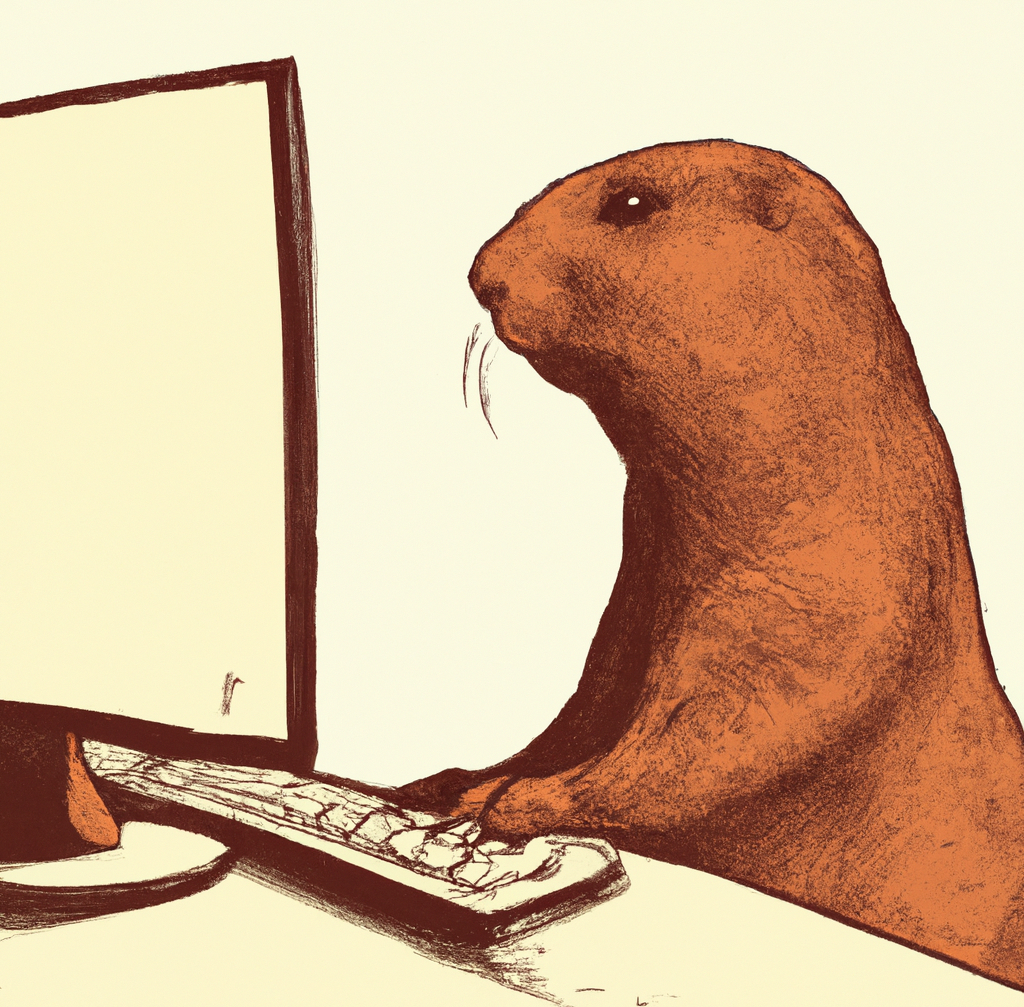
Packages and Modularization in Go: A Comprehensive Guide
In the world of software development, modularization is a key principle that ensures maintainability, reusability, and clarity. In the Go programming language, this principle is embodied through the use of packages and modules. In this blog post, we'll delve deep into the world of Go packages, Go Modules, and the best practices surrounding them.
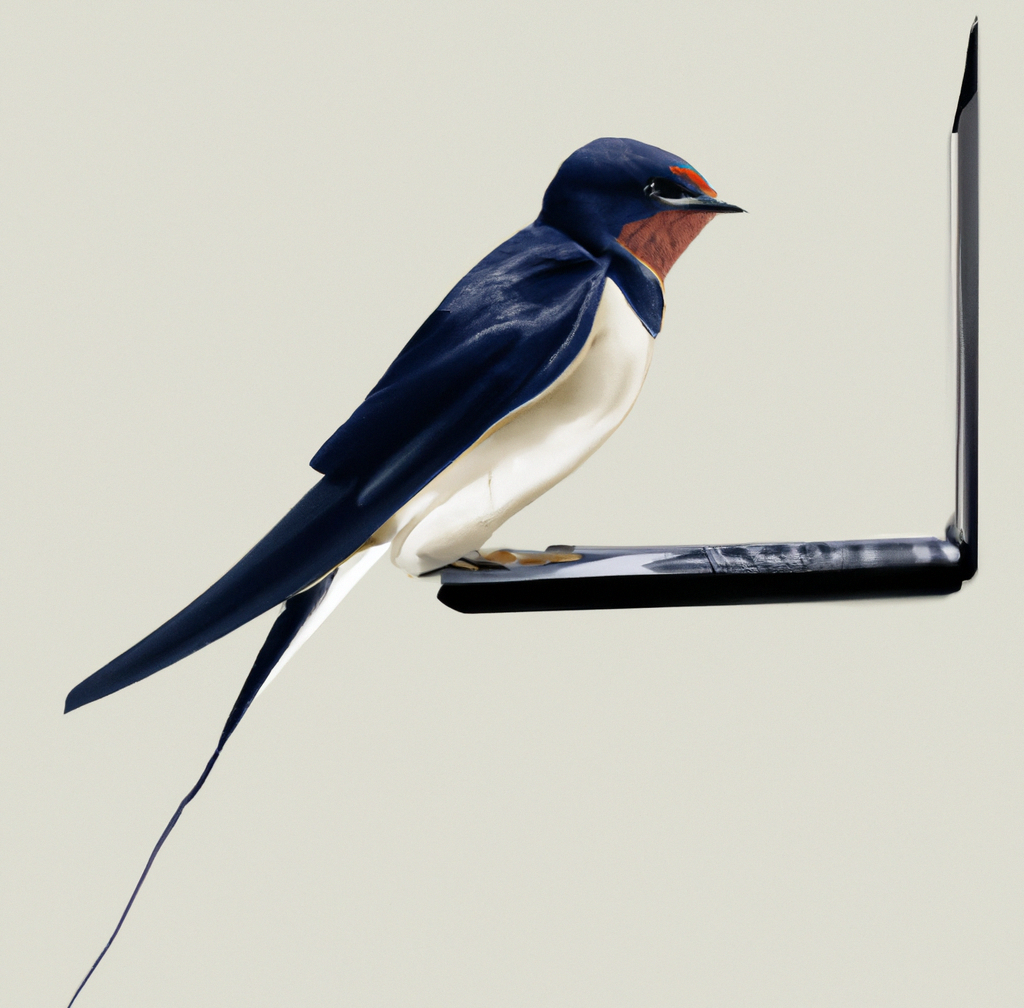
Swift Functions and Closures: A Comprehensive Guide
Swift, Apple's powerful and intuitive programming language, has gained immense popularity since its inception. One of the core components of Swift that makes it so versatile is its approach to functions and closures. In this blog post, we'll dive deep into the world of Swift functions and closures, exploring their definitions, usages, and intricacies.