Curious About Technology
Welcome to Coding Explorations, your go-to blog for all things software engineering, DevOps, CI/CD, and technology! Whether you're an experienced developer, a curious beginner, or simply someone with a passion for the ever-evolving world of technology, this blog is your gateway to valuable insights, practical tips, and thought-provoking discussions.
Recent Posts

Introduction to Swift Programming Language
Swift is a powerful and intuitive programming language developed by Apple for iOS, macOS, watchOS, and tvOS. Since its introduction in 2014, Swift has gained immense popularity due to its performance, modern syntax, and safety features. It's designed to be more concise and resilient against erroneous code, making it an excellent choice for both beginners and experienced developers.

Implementing a Red-Black Tree in Golang
Red-Black Trees are a type of self-balancing binary search tree. Each node in the tree has an extra attribute for denoting the color of the node, either red or black.

Reflection and the reflect Package in Go
Reflection in programming refers to the ability of a program to inspect its own structure, particularly through types; it's a form of metaprogramming. In Go, this capability is provided by the reflect package. While powerful, reflection can be tricky and should be used judiciously.
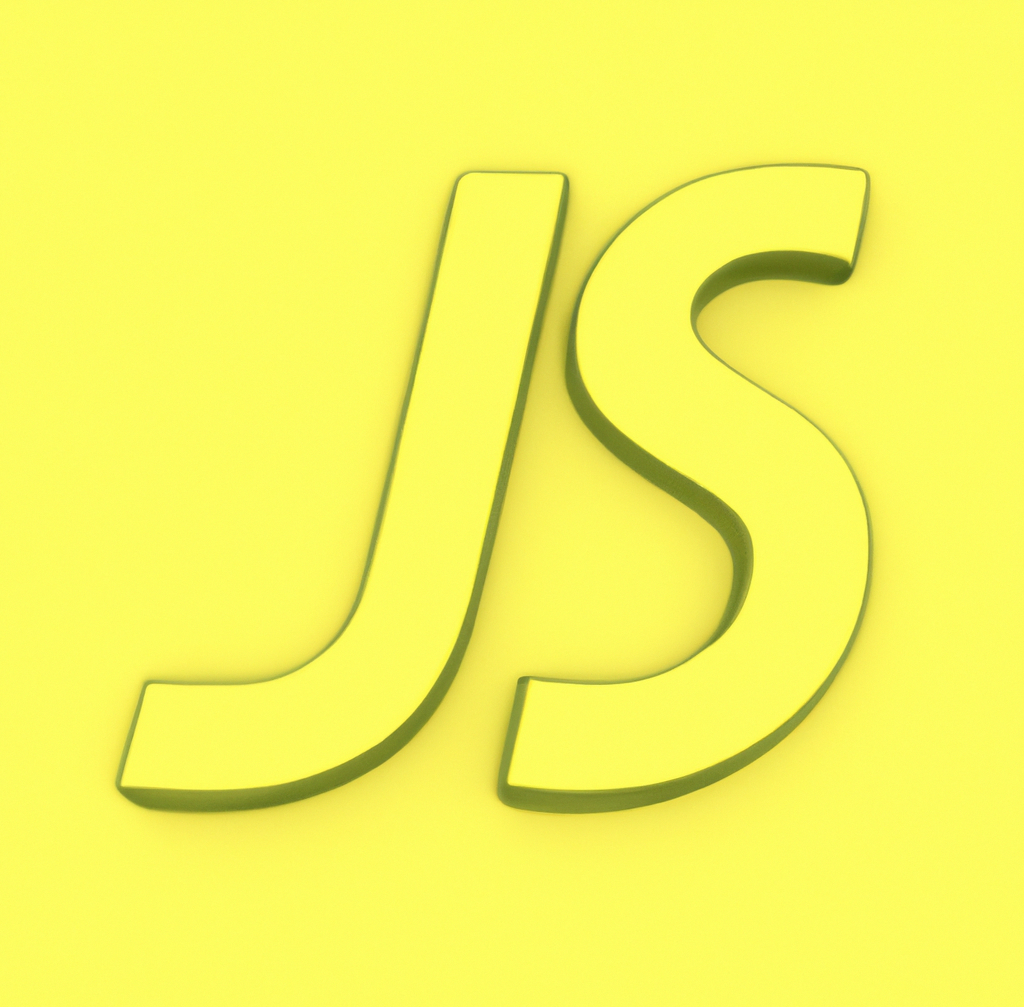
JavaScript's Prototype Chain Explained
JavaScript is a unique language in many ways, and one of its most distinctive features is its approach to inheritance. Unlike many other languages that use classical inheritance, JavaScript uses prototypal inheritance. In this post, we'll dive deep into the prototype chain, explore how it differs from classical inheritance, and look at some practical use cases.

Advanced Go WebAssembly: Exploring the Go Standard Library's Wasm Offerings
In the ever-evolving landscape of web development, WebAssembly (or Wasm for short) is making significant waves by allowing non-JavaScript languages to run in the browser. Go, a statically-typed, compiled language, has embraced this revolution and offers robust support for WebAssembly out of the box.

Getting Started with WebAssembly in Go: A Step-by-Step Guide
WebAssembly (Wasm) has emerged as a potent alternative to JavaScript for web applications. It offers performance improvements, compact binary format, and allows us to use languages other than JavaScript on the web. One of the languages that has extensive support for WebAssembly is Go.
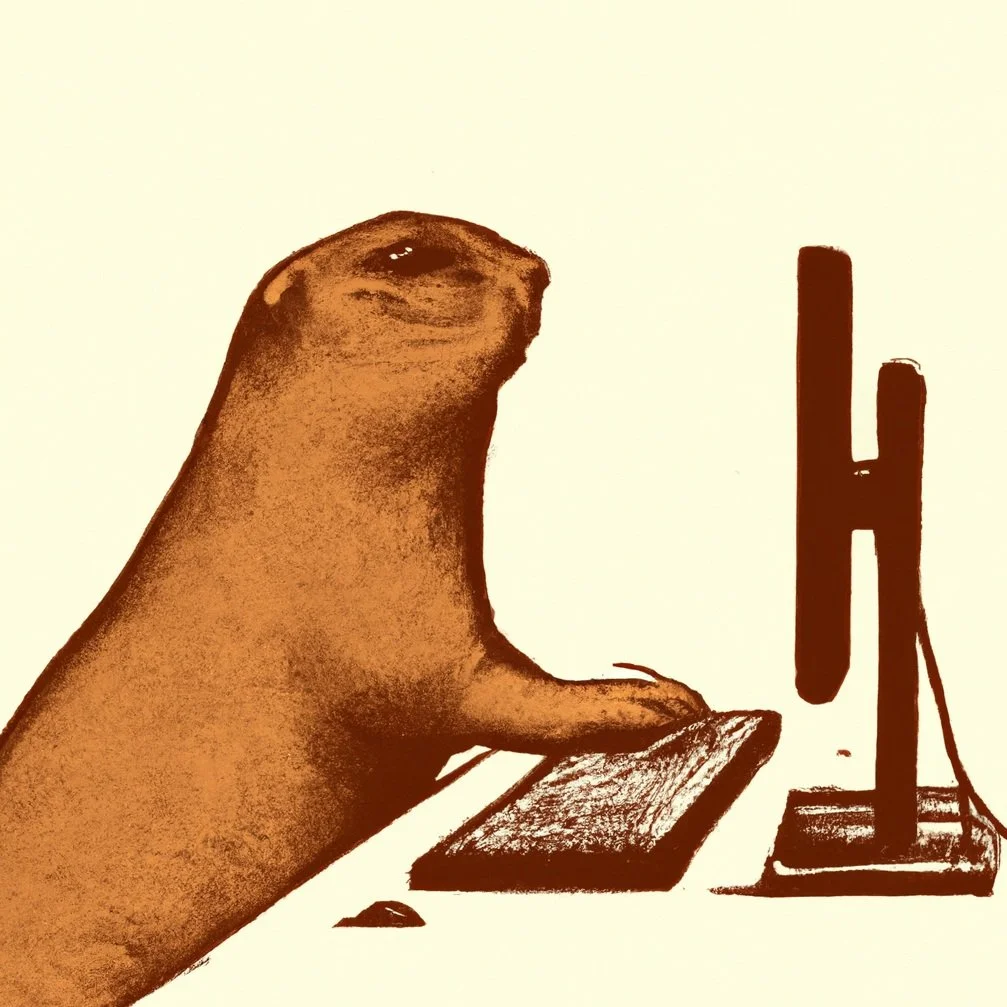
Performance Implications: Generics in Go
Generics, one of the most anticipated features in Go, have opened up a world of possibilities for developers, allowing for more flexible and type-safe code. However, as with any powerful tool, it's essential to understand the performance implications of using generics. In this blog post, we'll delve into how generics can impact the performance of Go programs and offer tips on optimizing code that uses generics.

Go Generics: An Introduction to Constraints and Type Lists
Ever since Go's inception, the community had been debating and contemplating the introduction of generics. After years of anticipation, generics are finally part of the language. One of the core features of generics in Go is the idea of 'Constraints and Type Lists'. This blog post aims to demystify this aspect of Go generics and provide an intuitive understanding of its use cases.
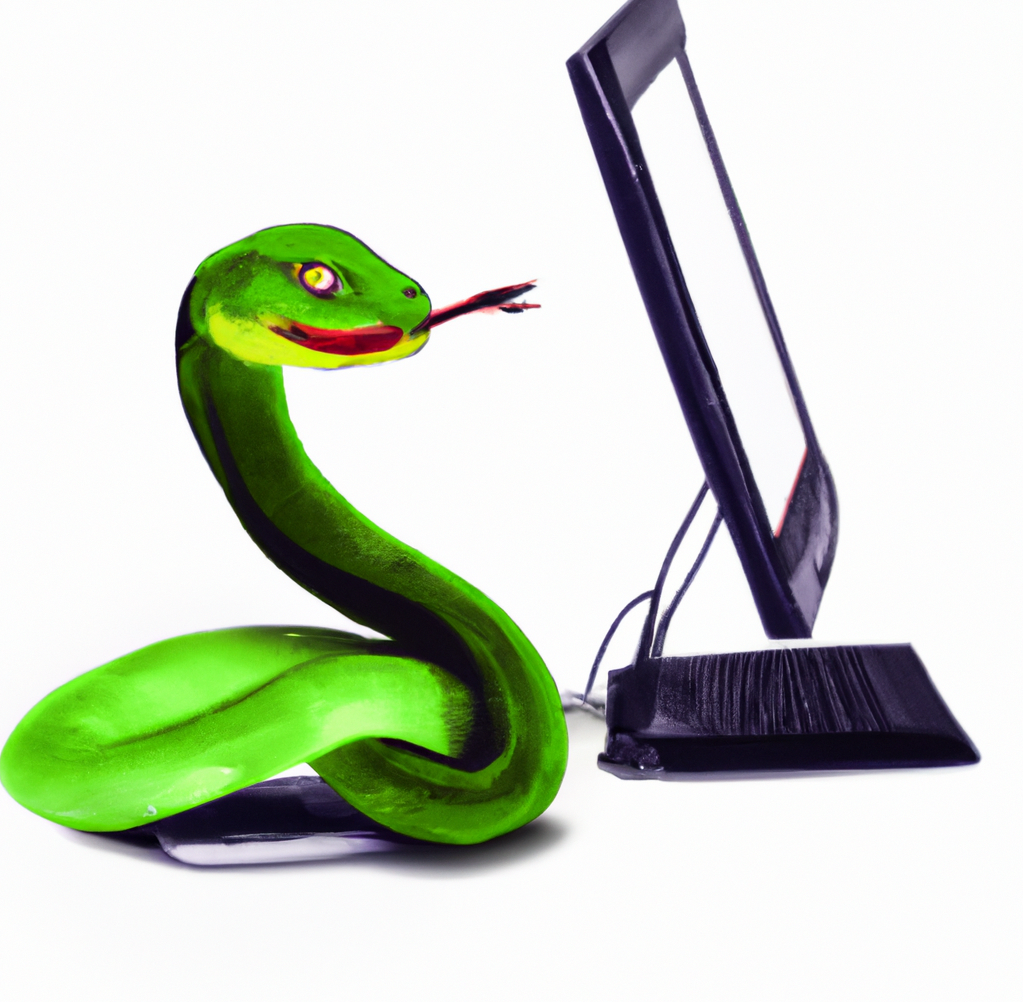
Understanding the Differences between Lists and Tuples in Python
Python, one of the world's most popular programming languages, offers a rich set of data structures to handle collections of items. Two of these structures, lists and tuples, are frequently used by Python developers. At a glance, they might seem similar since both can be used to store collections of items. However, they have key differences that make them unique in their own right. In this blog post, we'll delve deep into the differences between lists and tuples.

Unraveling CGo: Bridging the Gap Between Go and C
While Go (often referred to as Golang) is revered for its simplicity and concurrency model, there are instances when developers may need to interoperate with C libraries, mainly due to performance, leveraging existing libraries, or for compatibility reasons. This is where CGo comes into play. CGo provides a mechanism to integrate Go code with C code seamlessly.

Merge Sort Using Concurrency
Concurrency can significantly enhance the performance of many algorithms, and merge sort is no exception. By parallelizing specific tasks, we can often gain faster results by harnessing the power of multiple processing units. This blog post will dive into the idea of improving the merge sort algorithm using concurrency and then illustrate it with an example written in Go.

Understanding Memory Escape in Golang
One of the strongest selling points for Go (often referred to as Golang) is its powerful and transparent memory management, backed by a built-in garbage collector. However, as with many high-level languages, understanding the nuances of how memory is managed can lead to more performant code. A key concept in this area for Go developers is "memory escape."

Working with Lists in Python: A Guide
Lists are one of the most basic and widely used data structures in Python. They are versatile, simple to use, and offer a wide range of methods that allow for various manipulations. In this blog post, we will explore some fundamental operations that can be performed on lists in Python.

Understanding Memory Leaks in Go and How to Avoid Them
Go (or Golang) is admired for its simplicity, efficiency, and robustness. One of the reasons behind its rapid adoption is its built-in garbage collector which automatically frees memory that is no longer being used. Yet, despite having a garbage collector, memory leaks can still plague Go applications. In this post, we'll delve into memory leaks in Go: what they are, how to identify them, and, most importantly, how to prevent them.

Effortless Concurrency with sync and context in Go
In the world of concurrent programming, Go has earned its stripes as a language that excels in handling concurrency and parallelism gracefully. With its built-in concurrency primitives, such as goroutines and channels, Go simplifies the process of creating concurrent applications.
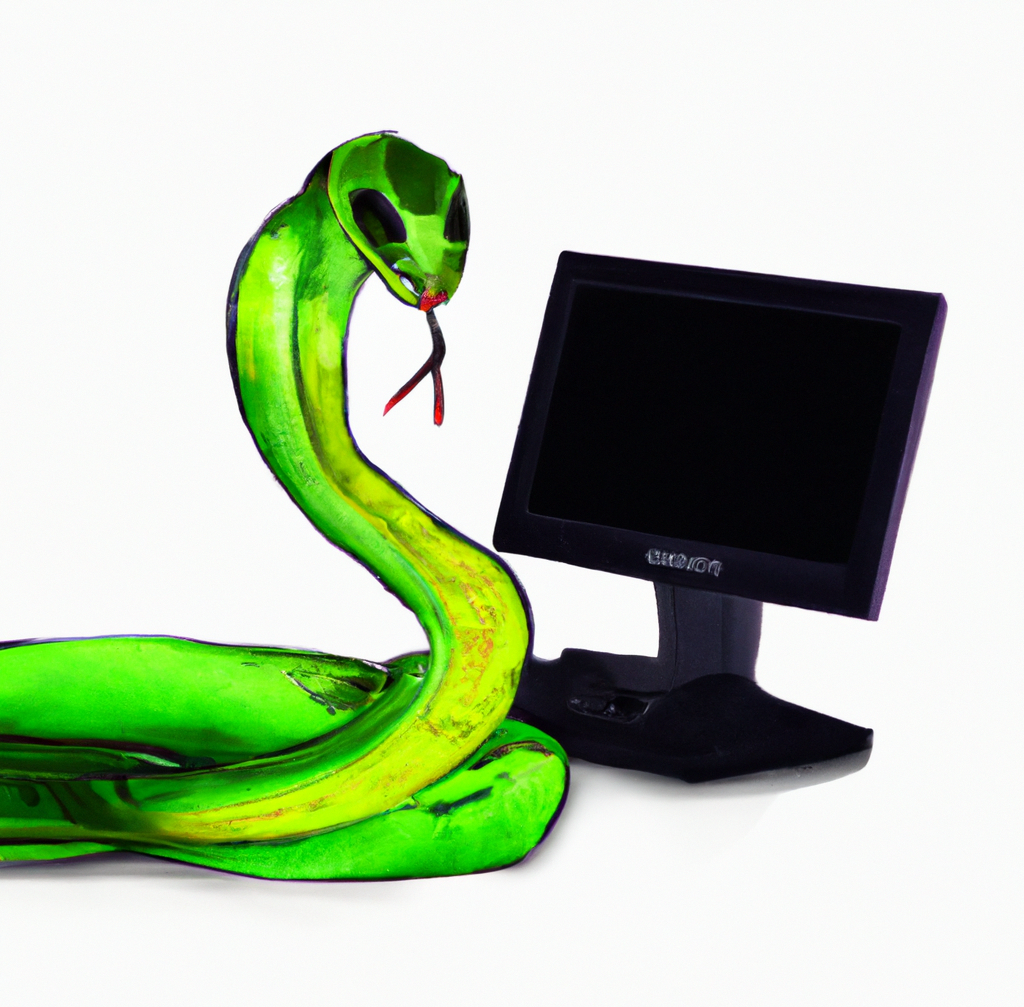
Exploring the Importance and Benefits of the Factory Pattern in Software Engineering
In the realm of software engineering, design patterns play a pivotal role in ensuring code quality, maintainability, and scalability. One such pattern that stands out for its importance and widespread applicability is the Factory Pattern. The Factory Pattern is a creational design pattern that provides an elegant way to create objects without exposing the instantiation logic to the client.
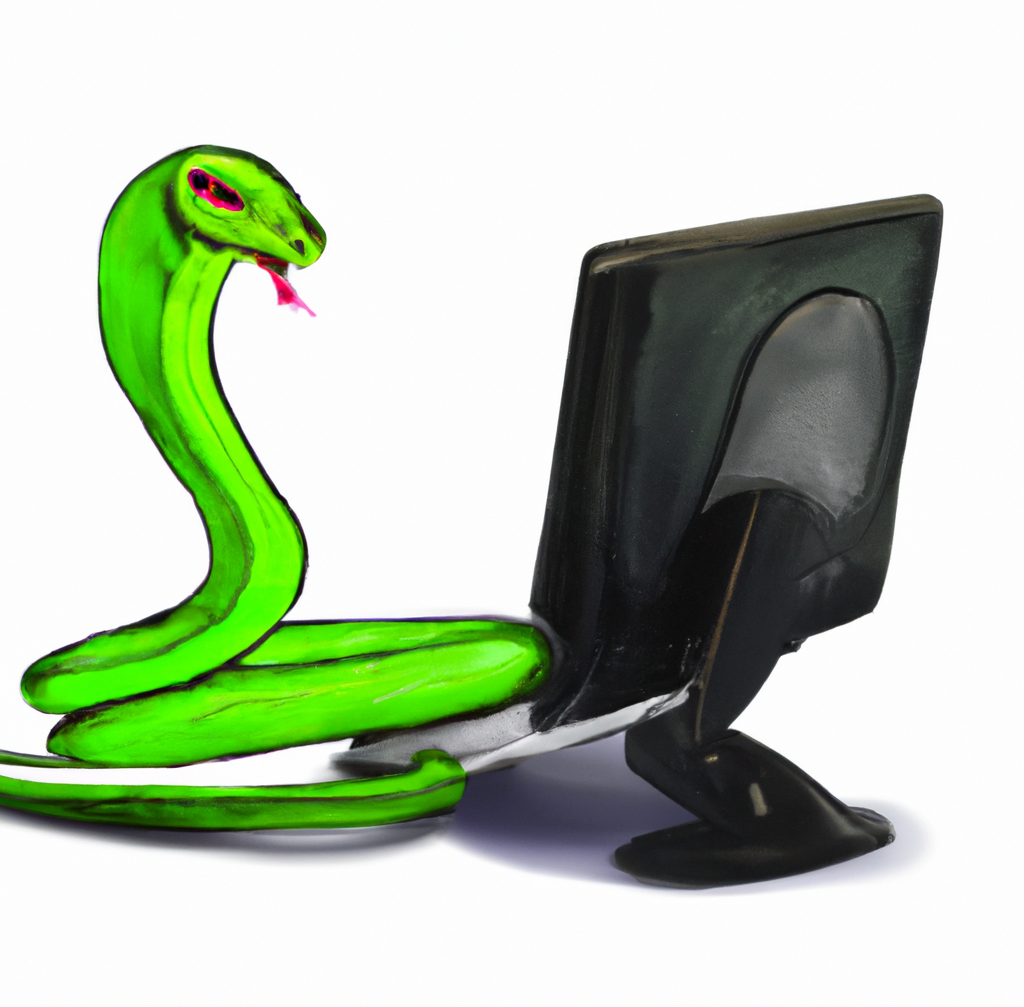
Mastering File Management and System Administration with Python Scripting
Python, a powerful and versatile programming language, provides a wide range of libraries and tools that can significantly simplify file management and system administration tasks. In this blog post, we will explore the potential of Python scripting and how it can be leveraged to streamline various administrative tasks, including file manipulation, directory handling, and system monitoring.

Empowering Go Development with Generics: A New Era of Reusability and Flexibility
One long-standing limitation was the absence of generics, which often led to code duplication and challenges in writing flexible, type-agnostic algorithms. In early 2022, Go 1.18 introduced generics, bringing a new era of reusability and flexibility to the language.

Writing Efficient Go Code: Best Practices for Performant and Idiomatic Programs
Writing efficient code is essential for creating high-performing applications. GoLang, with its simplicity and focus on performance, offers developers a unique opportunity to build efficient software.

Mastering Error Handling in GoLang: A Guide to "error," "panic," and "recover"
Error handling is a critical aspect of software development as it allows developers to gracefully handle unexpected situations and ensure the robustness and reliability of their applications. In GoLang, error handling is straightforward yet powerful, providing developers with multiple tools like the "error" interface, "panic," and "recover" mechanisms.