Curious About Technology
Welcome to Coding Explorations, your go-to blog for all things software engineering, DevOps, CI/CD, and technology! Whether you're an experienced developer, a curious beginner, or simply someone with a passion for the ever-evolving world of technology, this blog is your gateway to valuable insights, practical tips, and thought-provoking discussions.
Recent Posts
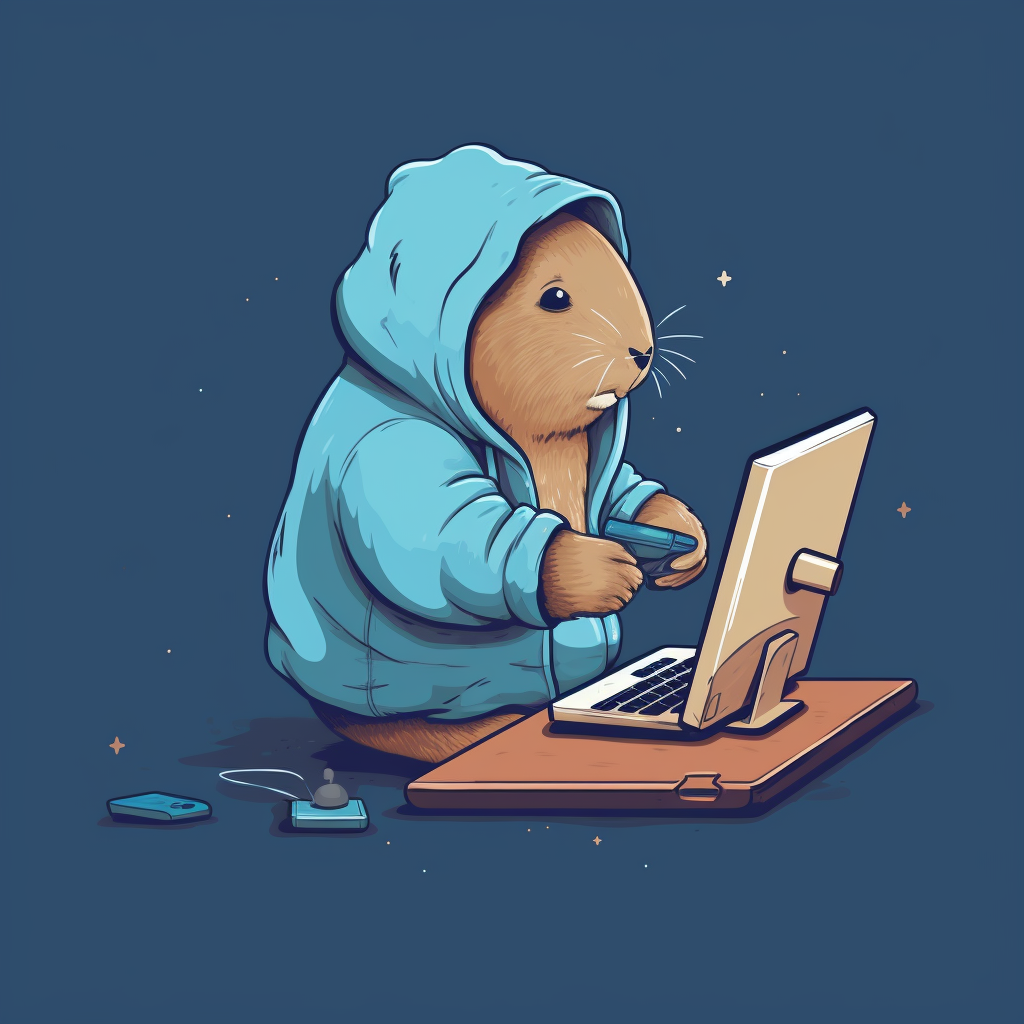
Design Pattern Series: Enhancing Functionality Elegantly with the Decorator Pattern
In the world of software design, patterns play a crucial role in solving common problems in a structured and efficient manner. Among these, the Decorator pattern stands out for its ability to add new functionality to objects dynamically. In Go, a language known for its simplicity and efficiency, implementing the Decorator pattern can significantly enhance the functionality of your code without cluttering the core logic.
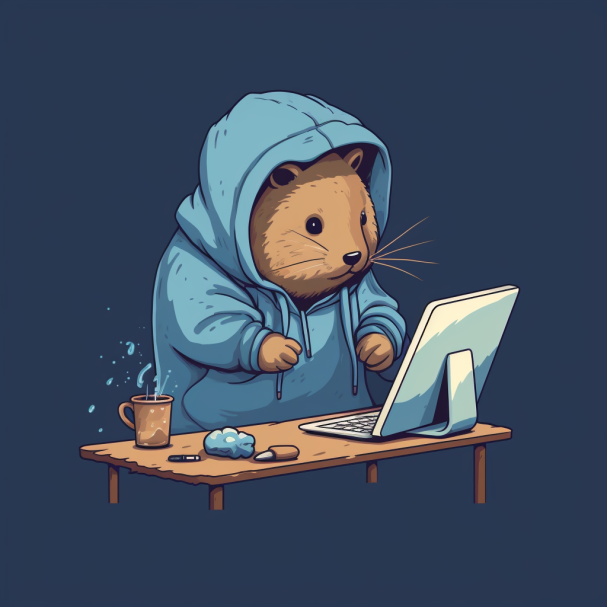
Design Pattern Series: Simplifying Complex Interfaces with the Adapter Pattern
In the world of software design, the Adapter pattern is a crucial tool for ensuring that different parts of a system can work together smoothly. This is particularly relevant in Go, a language known for its simplicity and efficiency.
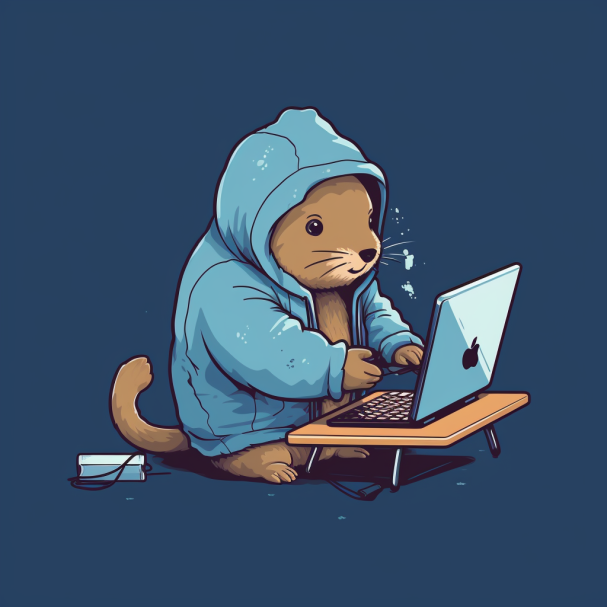
Design Pattern Series: Understanding the Abstract Factory Pattern
In the world of software design, design patterns serve as blueprints for solving common design problems. Among these, the Abstract Factory Pattern is a creational pattern used to create families of related or dependent objects without specifying their concrete classes. In Go, a language known for its simplicity and efficiency, implementing the Abstract Factory Pattern can streamline your development process, particularly when dealing with complex object creation.
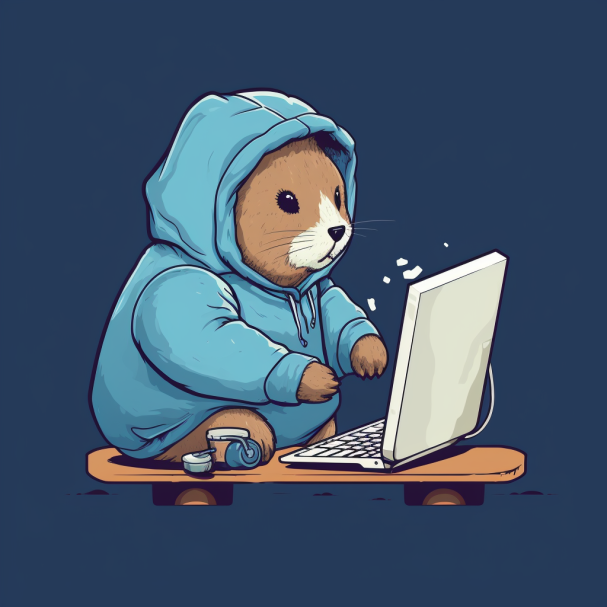
Design Pattern Series: Simplifying Object Creation with the Prototype Pattern
In the world of software design, patterns play a crucial role in solving common design problems. One such pattern, the Prototype pattern, is particularly useful in scenarios where object creation is a costly affair. This blog post delves into the Prototype pattern, its significance, and its implementation in Go, a popular statically typed, compiled programming language known for its simplicity and efficiency.
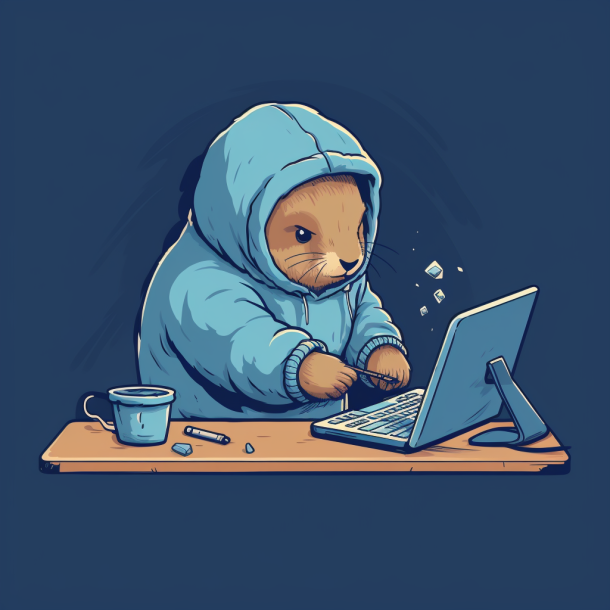
Design Pattern Series: Mastering the Builder Pattern in Go
In the realm of software development, the art of selecting the right design pattern is akin to setting the foundations of a building. It's a decision that reverberates through the lifecycle of your code, impacting its clarity, efficiency, and ease of maintenance. When working with Go, a language celebrated for its lean and effective style, this choice becomes even more pivotal.
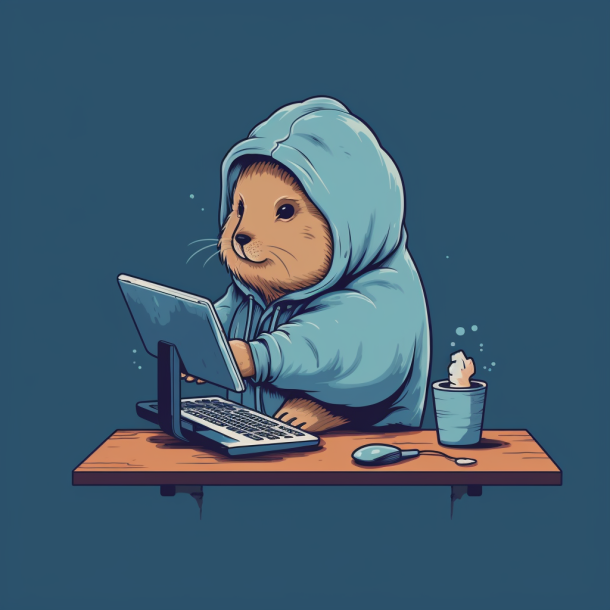
Design Pattern Series: Embracing the Factory Pattern for Flexibility and Scalability
We will embark on an insightful journey to uncover the rationale and process behind our team's decision to integrate the Factory pattern in a Go project. Our exploration will shed light on the transformative effect this pattern has had on the architecture of our application, offering valuable insights for fellow developers navigating similar paths.
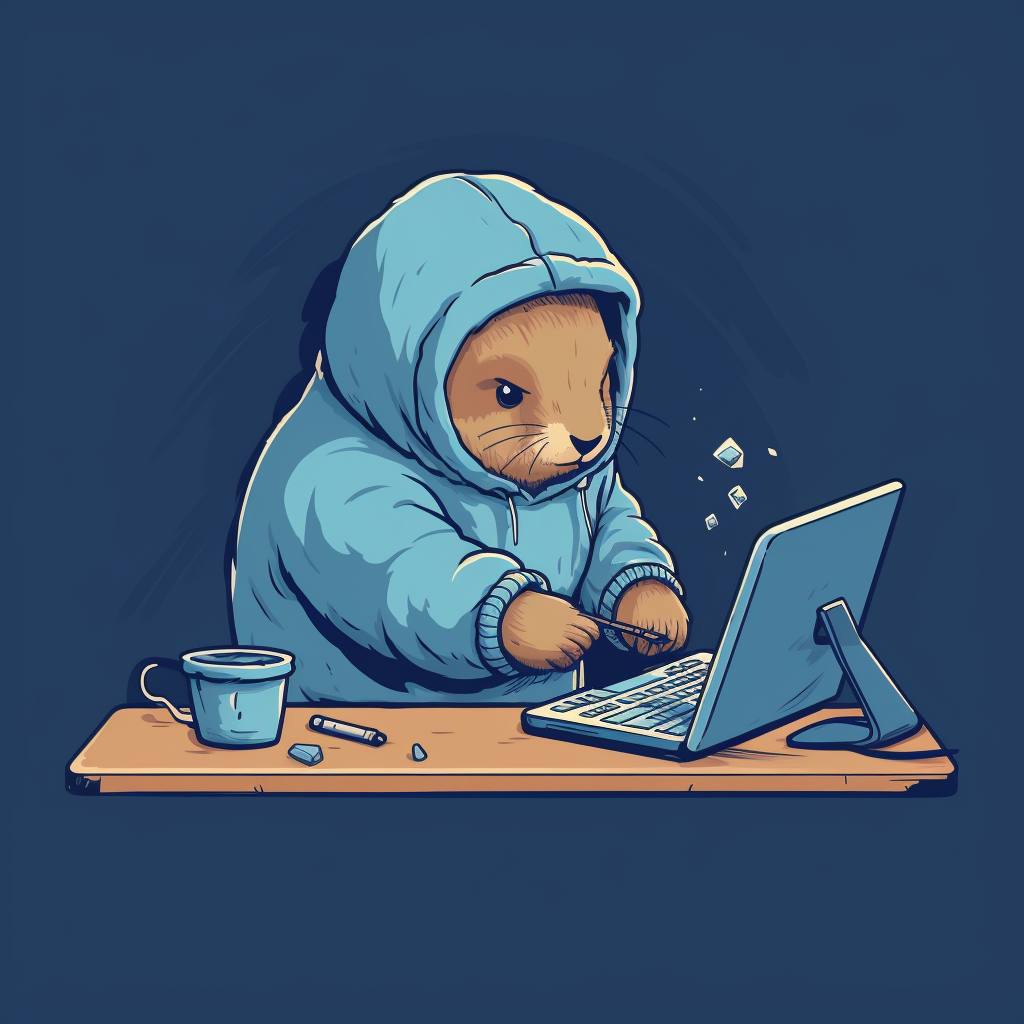
Design Pattern Series: Applying the Singleton Pattern in Go
In the intricate landscape of software engineering, design patterns stand out as vital blueprints, each crafted to methodically solve prevalent challenges. Among these, the Singleton pattern shines with a unique allure. Far from being generic fixes, these patterns offer adaptable guidelines tailored to meet distinct project needs.
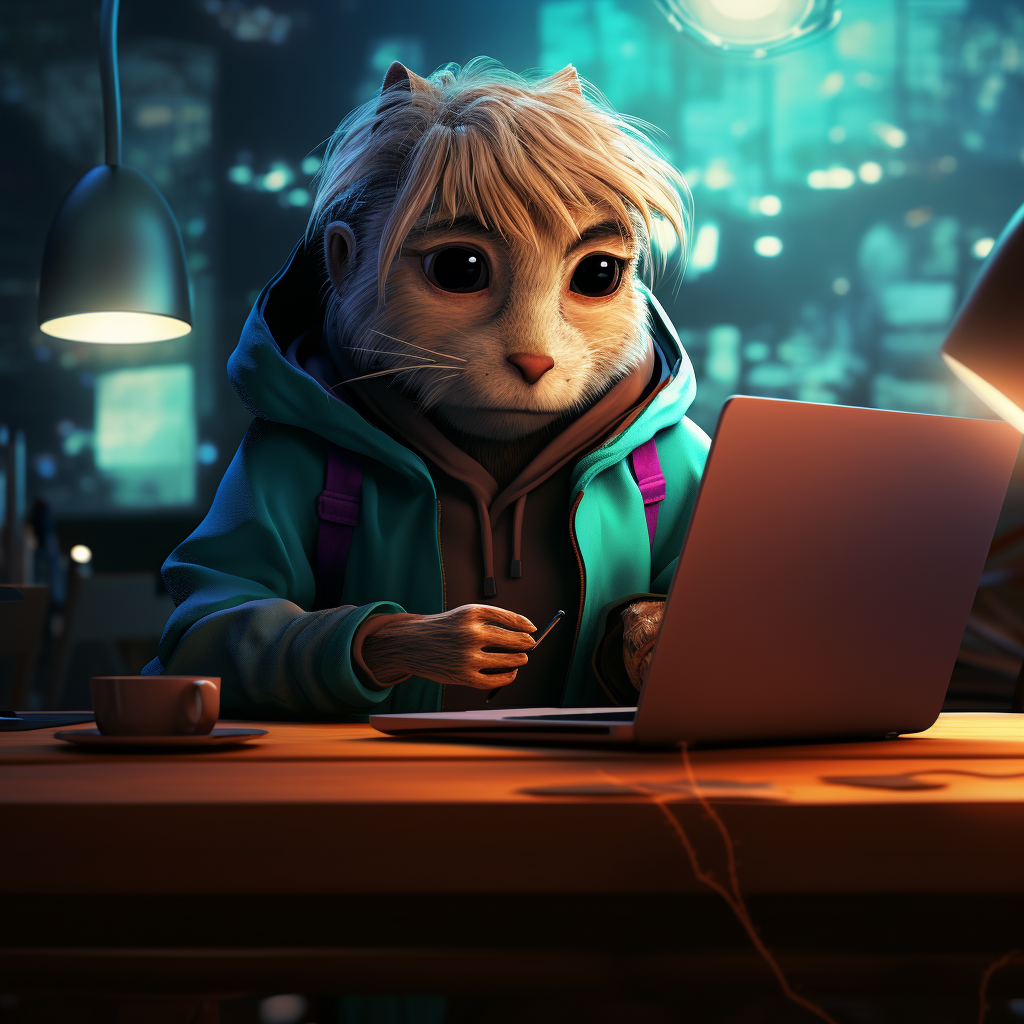
Interview Series: Writing Unit Tests in Go
Unit testing is a fundamental aspect of software development, ensuring that individual units of code function as expected. In the world of Go, writing unit tests is streamlined and integrated into the language's standard library, making it a seamless part of the development process.
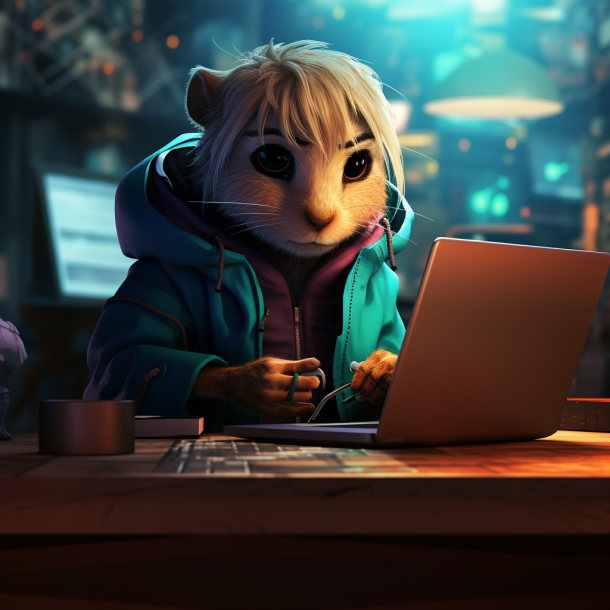
Interview Series: Profiling and Optimization Tools
Go has become a popular choice among developers for building fast and efficient software. One of the key reasons for its popularity is the rich set of tools it offers for profiling and optimizing the performance of programs.
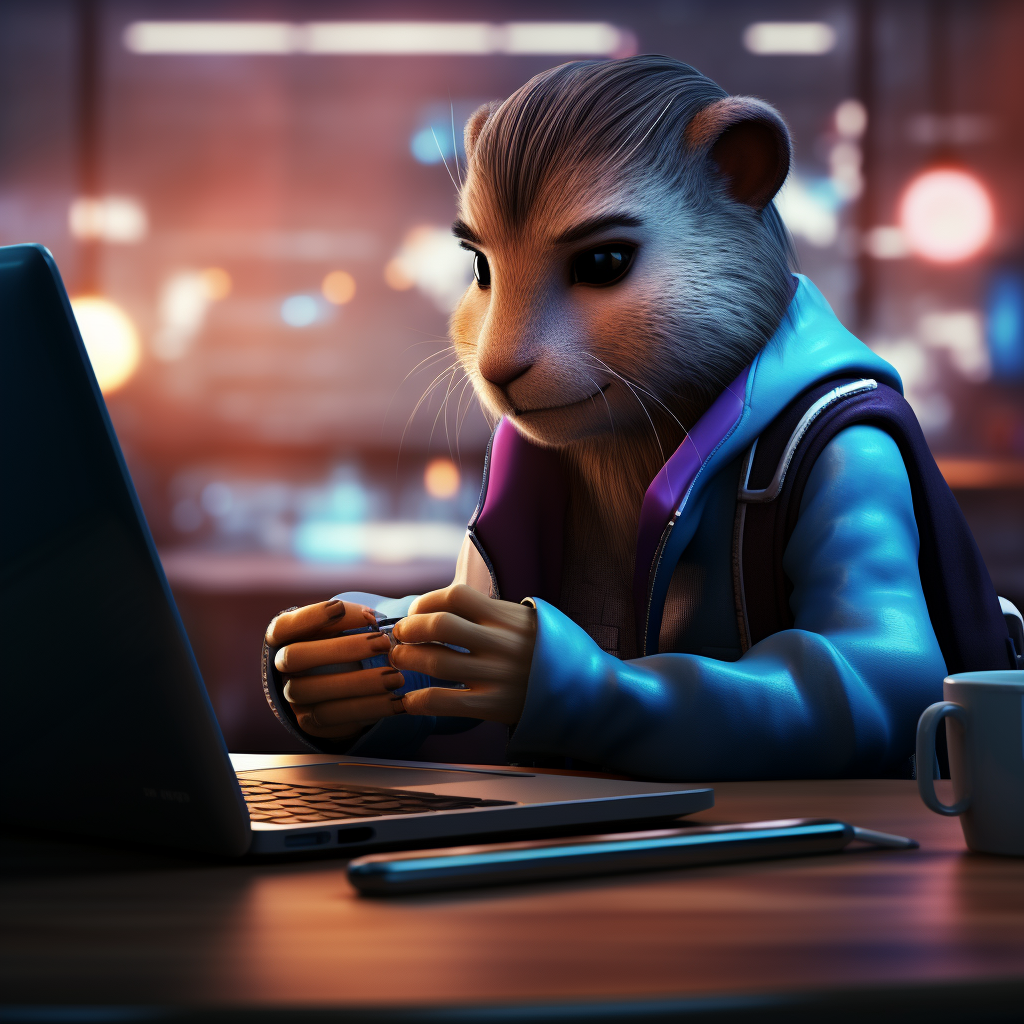
Interview Series: Most Frequently Used Standard Library Packages
Go is renowned for its simplicity, efficiency, and powerful standard library. The library provides a robust foundation that can serve almost any common programming need, from handling I/O to parsing JSON. In this post, we'll explore some of the most frequently used Go packages in the standard library and the purposes they serve in everyday coding.
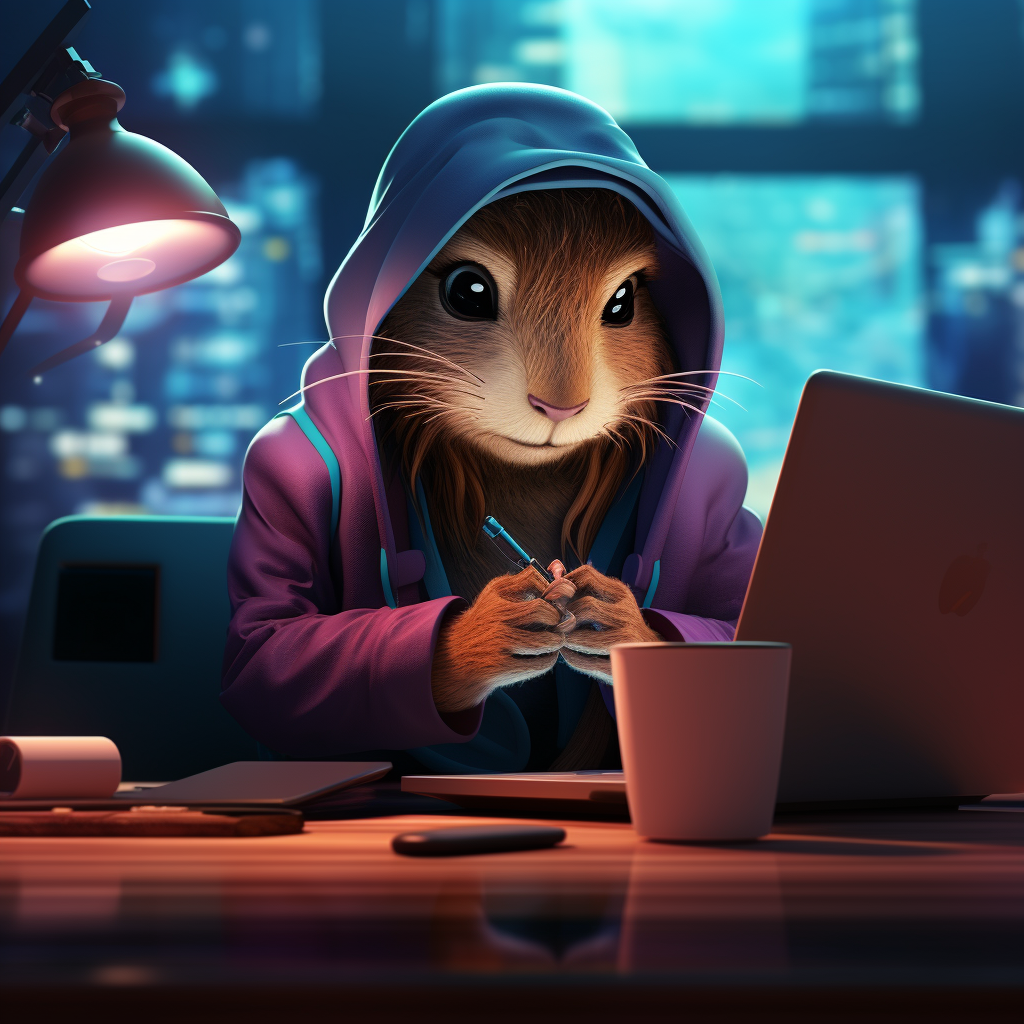
Interview Series: When to Use Buffered and Unbuffered Channels
Buffered and unbuffered channels are two types of communication pathways used in concurrent programming, particularly within the Go programming language ecosystem. They provide a means for goroutines (lightweight threads) to synchronize and communicate in a safe and efficient manner. Understanding when and why to use each can significantly affect the performance and correctness of concurrent applications.
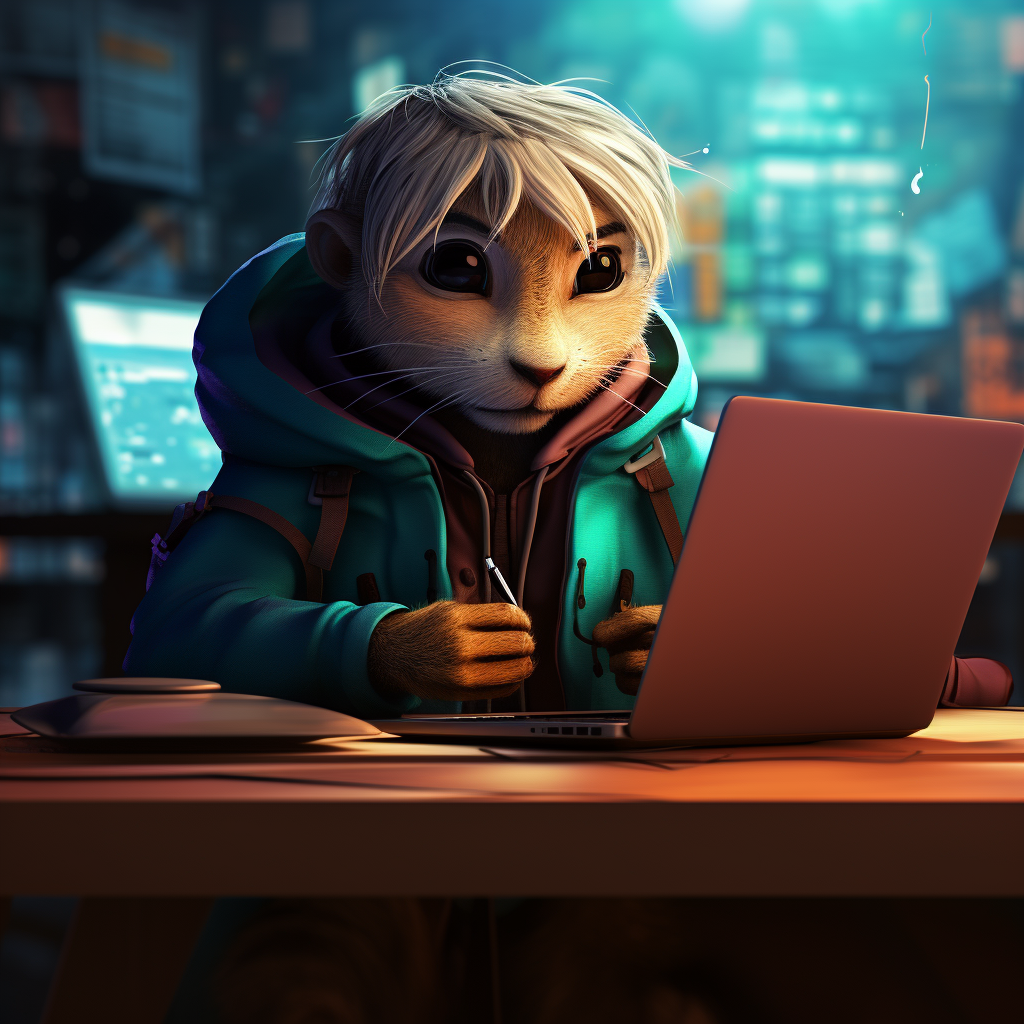
Interview Series: Understanding Memory Management in Go
Memory management is a critical aspect of any modern programming language. It ensures that a program uses the computer's memory efficiently, avoiding both wastage and shortages that could slow down or even halt execution. In the Go programming language, memory management is largely handled by a built-in garbage collector. But there are scenarios where manual memory management might be necessary.

Interview Series: Understanding Slices and Arrays in Go
When working with Go, one fundamental concept that often confuses newcomers is the difference between slices and arrays. Both are used to store collections of elements, but they serve different purposes and have different properties.

Understanding Error Handling in Go: A Different Approach from Traditional Exceptions
Error handling is an integral part of programming, as it helps in dealing with unexpected events and maintaining robust software. In Go, error handling is implemented in a way that is quite distinct from the exception mechanisms found in many other programming languages.
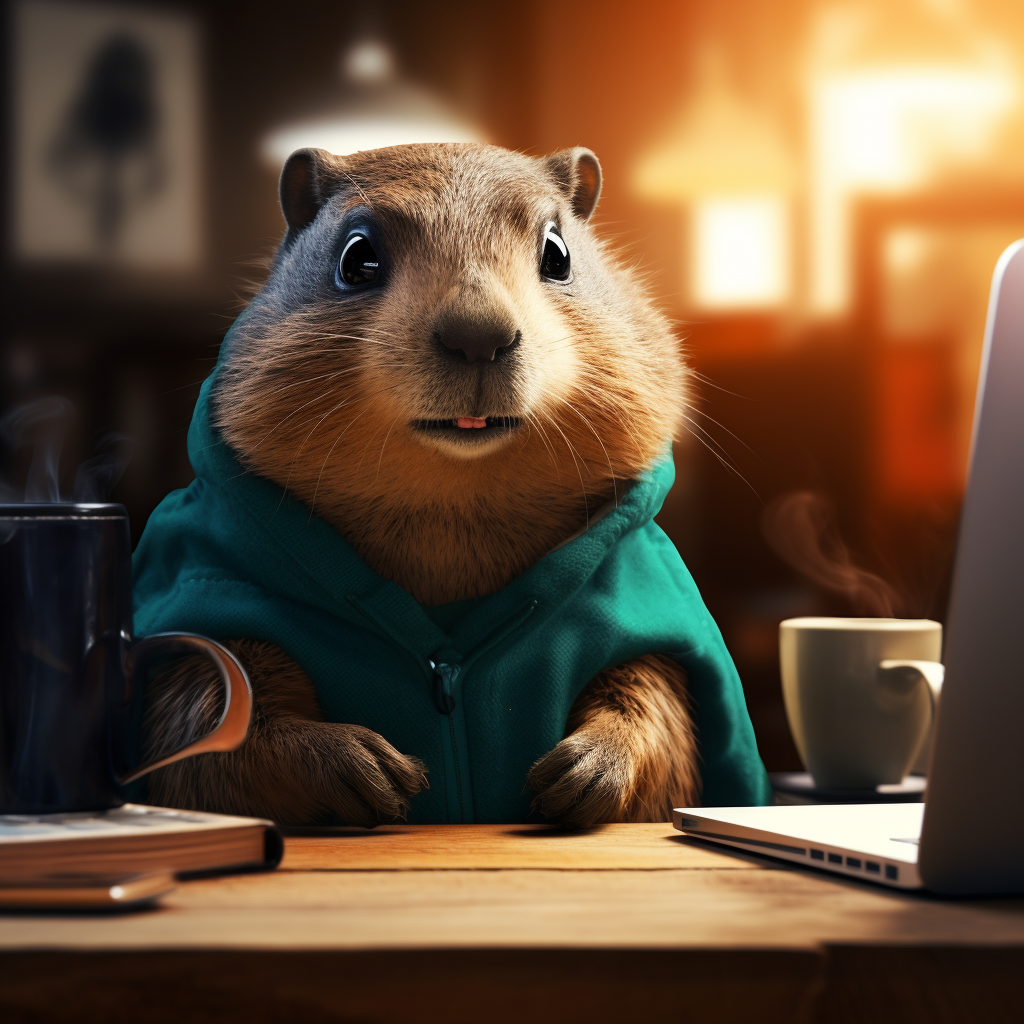
Interview Series: Understanding Deadlocks and Tools for Detection and Prevention
Concurrency is a core feature of Go, enabling programs to handle multiple tasks simultaneously. It's a powerful component of modern programming that can, when used correctly, lead to efficient and high-performance applications. However, concurrency also introduces the potential for certain types of bugs that are not present in sequential programming—one of the most notorious being deadlocks.
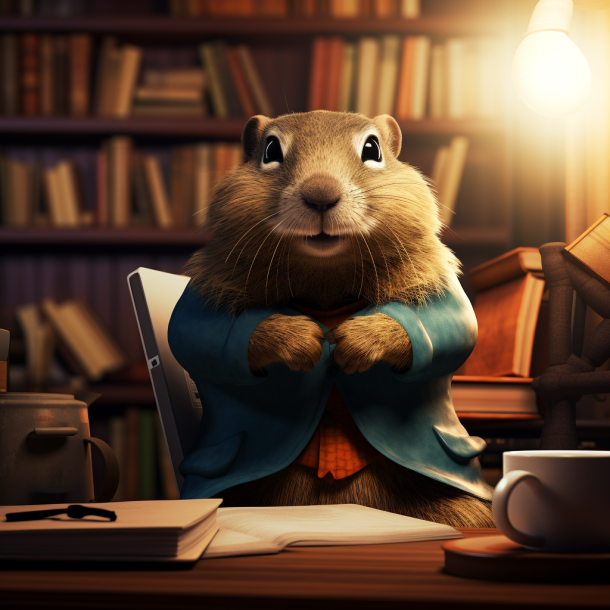
Interview Series: Understanding Goroutines in Go
When it comes to concurrent programming, the concept of 'threads' is often one of the first that comes to mind. However, if you've dipped your toes into the Go programming language, you may have come across a curious term: 'goroutine'. This unique approach to concurrency is one of the features that sets Go apart from other programming languages. But what exactly is a goroutine, and how does it differ from a thread in traditional threading models?
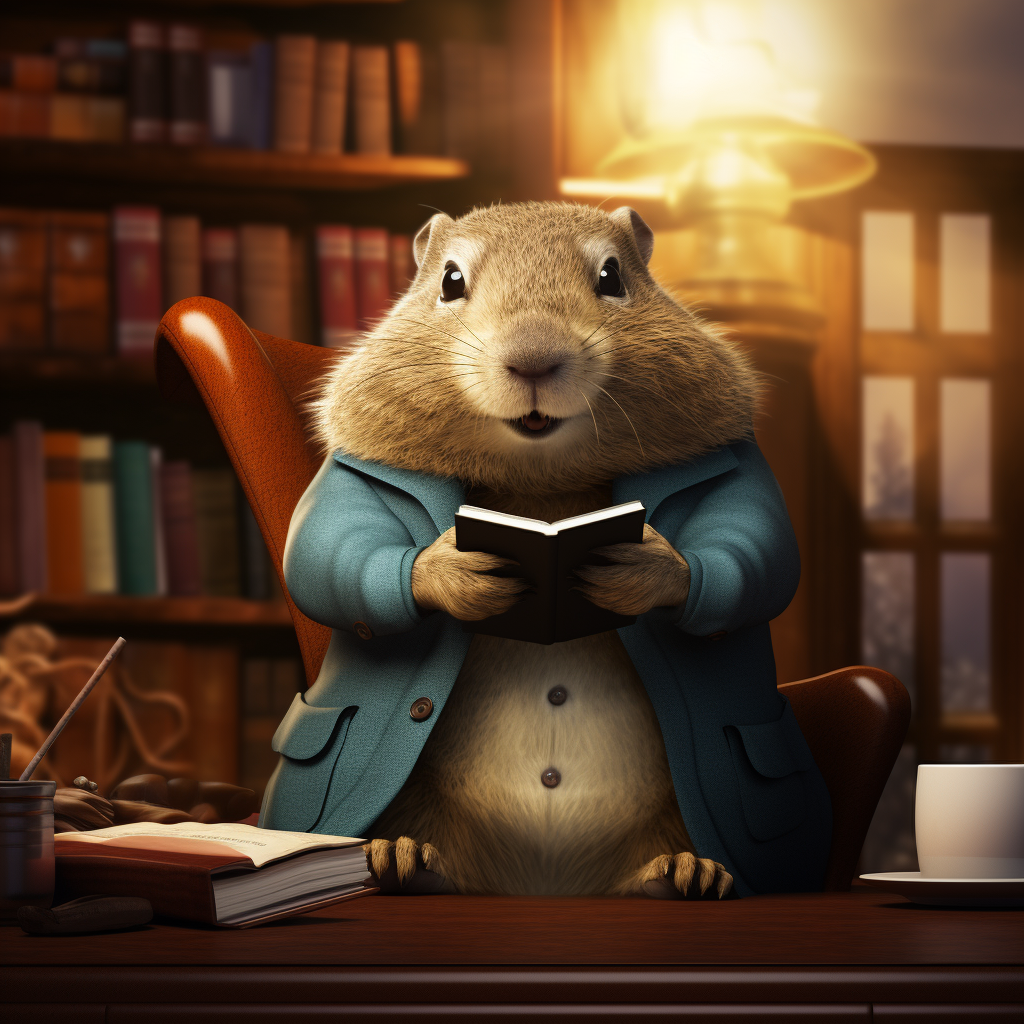
Mastering Regex Pattern Matching in Go: A Practical Guide
Regex, short for regular expressions, is a powerful tool for pattern matching and text manipulation that can make your life as a developer much easier—if you know how to use it, that is. In Go, regex is handled through the regexp package, which provides a rich set of functions to work with regular expressions.
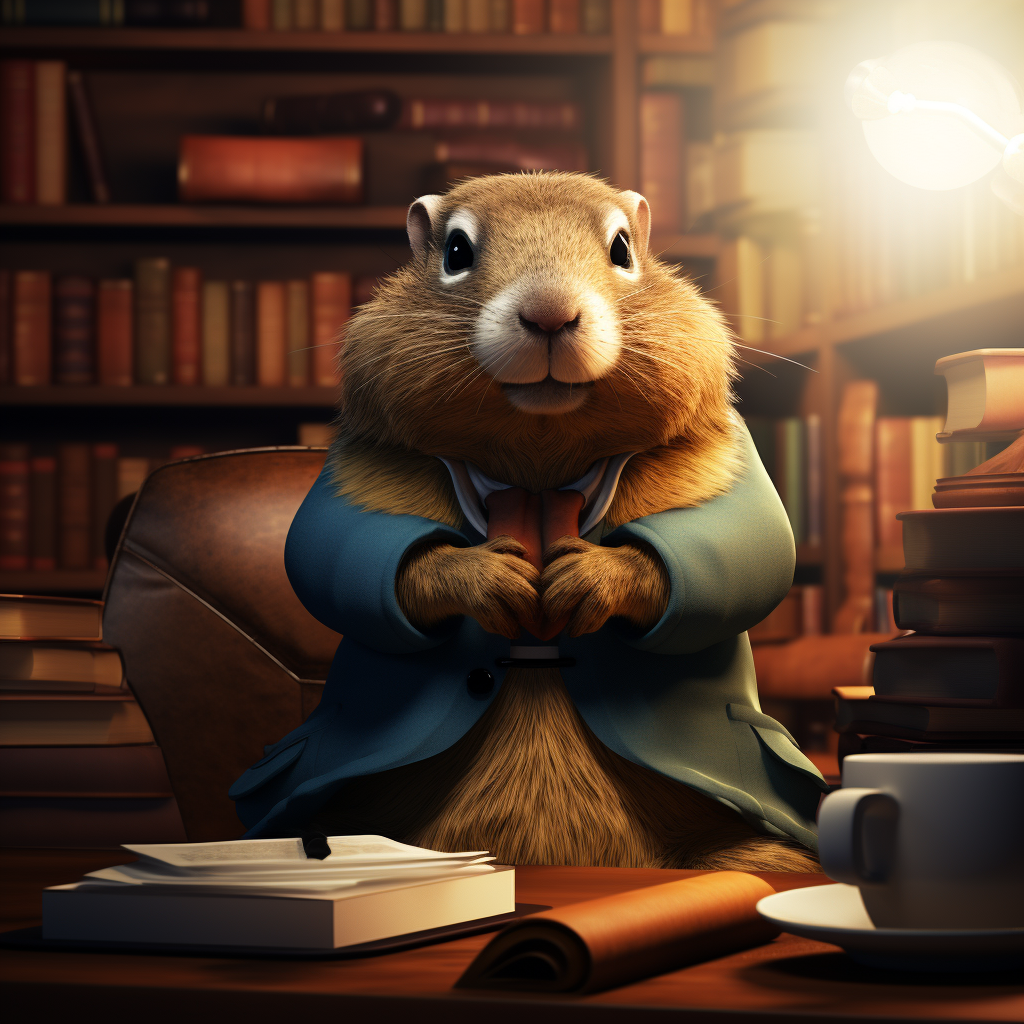
Understanding Encryption in Go: A Developer's Guide
Encryption is a critical aspect of securing data, ensuring that sensitive information remains inaccessible to unauthorized users. As a statically typed, compiled programming language, Go is renowned for its simplicity and efficiency, making it an excellent choice for implementing encryption.

Unlocking Performance Insights: CPU Profiling in Go
Performance is a critical factor for any application, and Go, with its reputation for efficiency, is no exception. As Go applications grow in complexity and scale, understanding where time is spent in your code becomes invaluable. CPU profiling is a powerful tool in a developer's arsenal to diagnose and resolve performance bottlenecks.

Understanding Streaming Data in Go
In the age of real-time analytics and big data, the ability to stream data efficiently is crucial for any application. Streaming data refers to a continuous flow of data that is processed sequentially and incrementally. Go with its lightweight goroutines and channels, is an excellent choice for building high-performance streaming data applications.