Curious About Technology
Welcome to Coding Explorations, your go-to blog for all things software engineering, DevOps, CI/CD, and technology! Whether you're an experienced developer, a curious beginner, or simply someone with a passion for the ever-evolving world of technology, this blog is your gateway to valuable insights, practical tips, and thought-provoking discussions.
Recent Posts
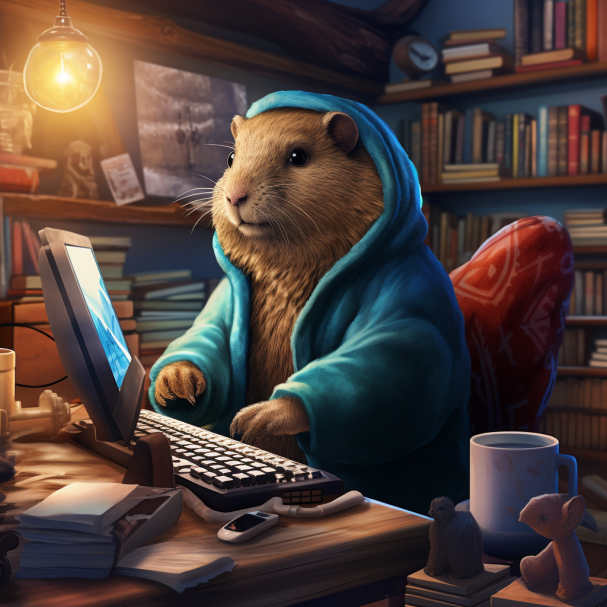
Advanced Techniques for Using Runes in Go Programming
Delve into the world of Go programming as we explore advanced techniques for handling runes. This blog provides practical code examples to master the use of runes in your Go projects.
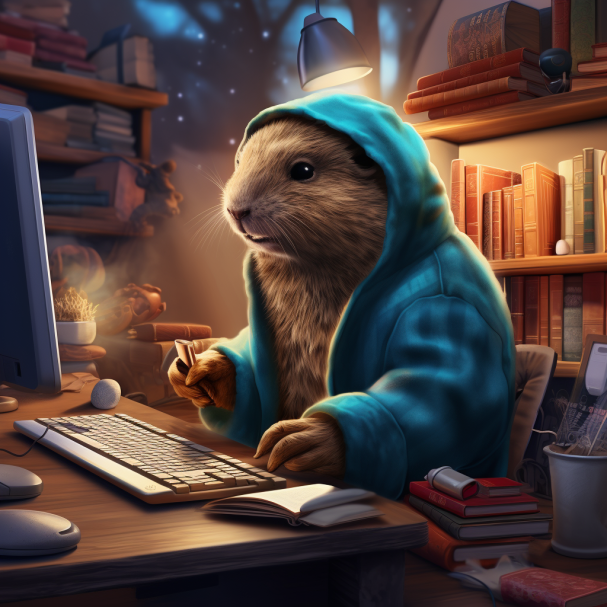
Mastering String Manipulation in Go: Techniques and Best Practices
Dive into the world of Go with our comprehensive guide on string manipulation, exploring methods, performance tips, and practical examples to improve your programming skills.
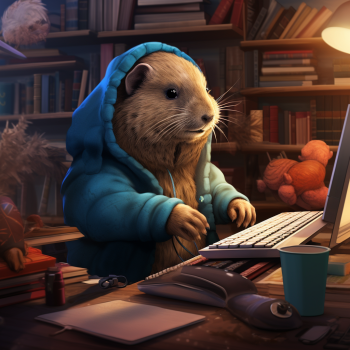
Goroutine Pools Explained: Maximize Efficiency in Go Applications
Goroutine pools are a critical component for efficient resource management in Go programming. This blog explains their importance, how to implement them, and best practices with actionable code examples.
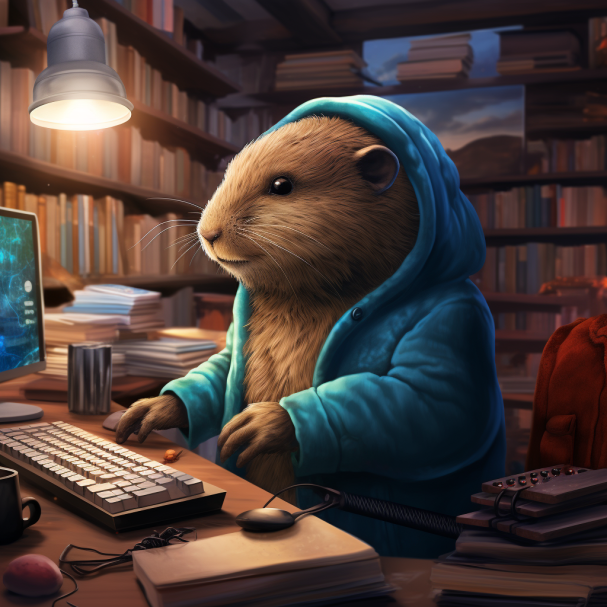
From Basics to Practical: Using Interface-Based Configuration in Go Programming
Discover how to implement the interface-based configuration pattern in Go. This guide includes a detailed code example to enhance your programming practices and streamline your configurations.
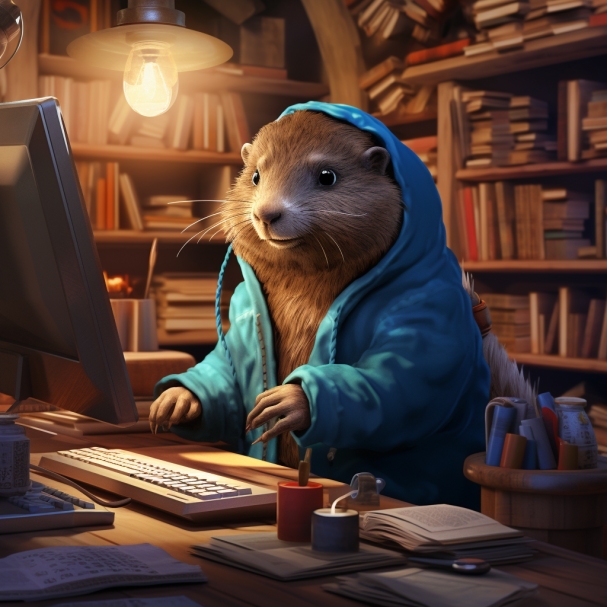
Unpacking the Functional Options Pattern in Go: Simplifying Configuration Complexity
The functional options pattern in Go provides a scalable and clean way to configure objects without overwhelming function signatures. Learn how to implement it with a practical example.

Understanding io.Pipe in Go: Streamline Your Data Flow
Explore the concept of io.Pipe in Go programming, an essential tool for managing data streams effectively between concurrent processes.
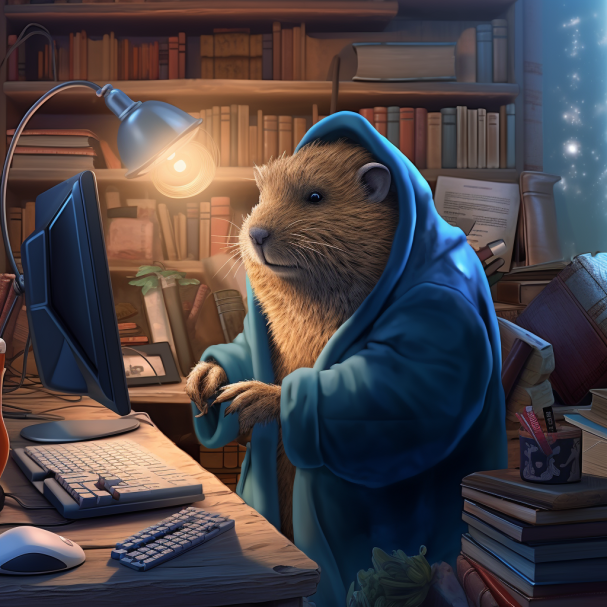
Mastering Request Tracing in Microservices with OpenTelemetry and Jaeger
Dive into the world of microservices to explore how OpenTelemetry and Jaeger can revolutionize the way you trace requests, ensuring your architecture is efficient, scalable, and easy to troubleshoot.
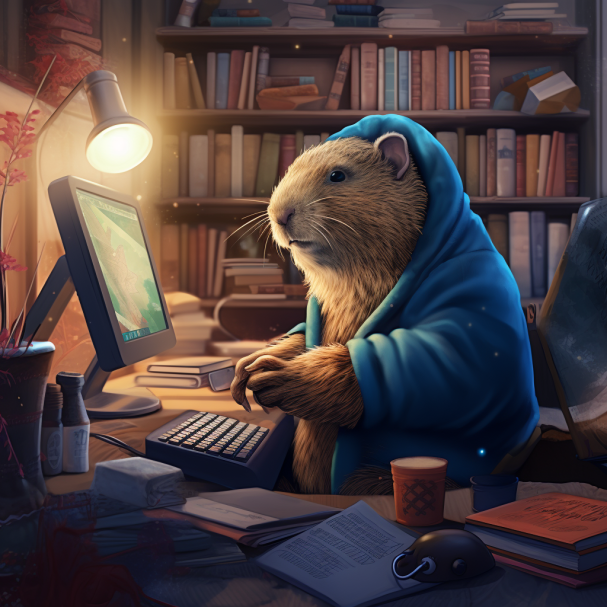
A Surge of Success: Preparing for High Traffic
Facing a flood of traffic can be daunting. Learn how to prepare your Go application to handle 100,000 requests without breaking a sweat, ensuring a seamless user experience.
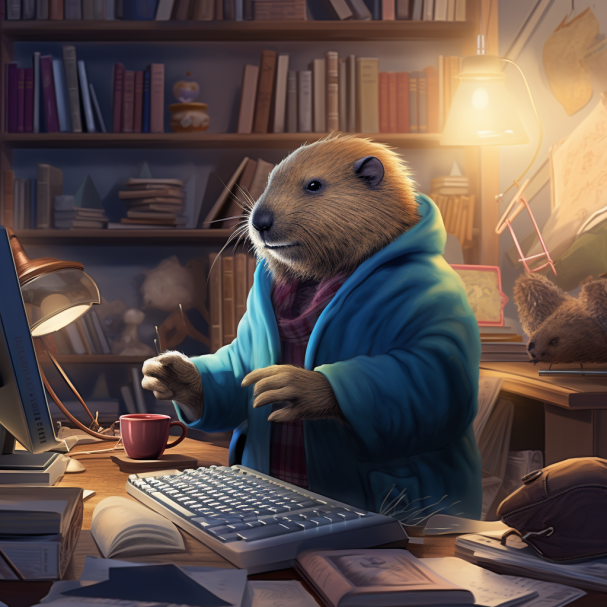
Unraveling the Mysteries of Go Struct Tags: A Comprehensive Guide
Ever wondered what struct tags in Go are all about? This post takes you through the what, why, and how of Go struct tags, including a handy list of tags and their specific uses. Perfect for both beginners and seasoned Go developers!
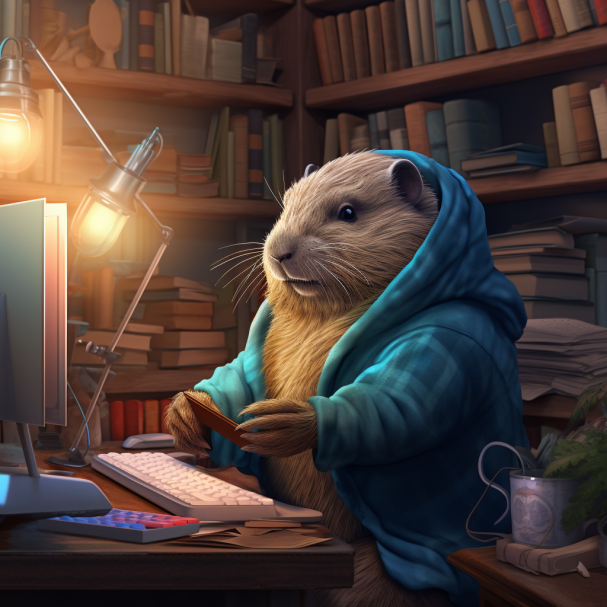
Speeding Up Your Go Development: Mastering Parallel Tests
Dive into the world of Go programming by mastering the art of running parallel tests. This comprehensive guide will walk you through everything you need to know to speed up your development process, making your testing phase as efficient as it gets. From best practices to overcoming common pitfalls, we've got you covered!
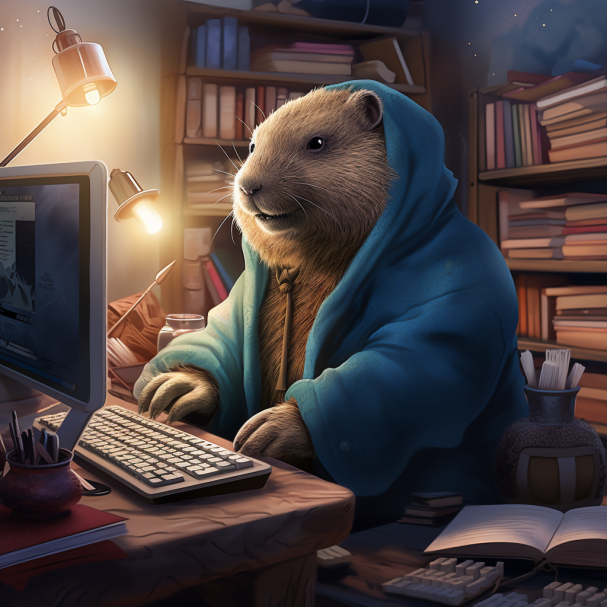
Mastering GOSSAFUNC: Unlocking Compiler Insights in Go Programming
Unveil the secrets of GOSSAFUNC in Go programming. Learn how it shapes compiler optimizations and transforms your coding experience, enhancing both efficiency and performance.
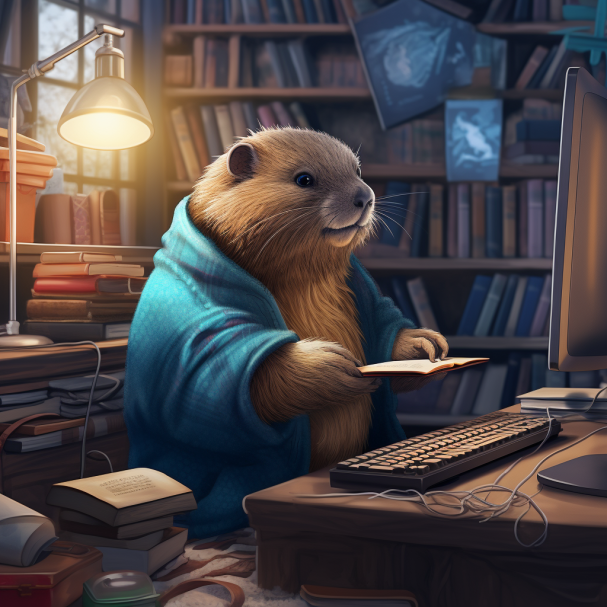
Understanding Pointers and uintptr in Go: Key Differences Explained
In the world of Go, pointers and uintptr are fundamental concepts that often cause confusion among newcomers. Both are used to deal with memory addresses, but they serve different purposes and obey different rules.
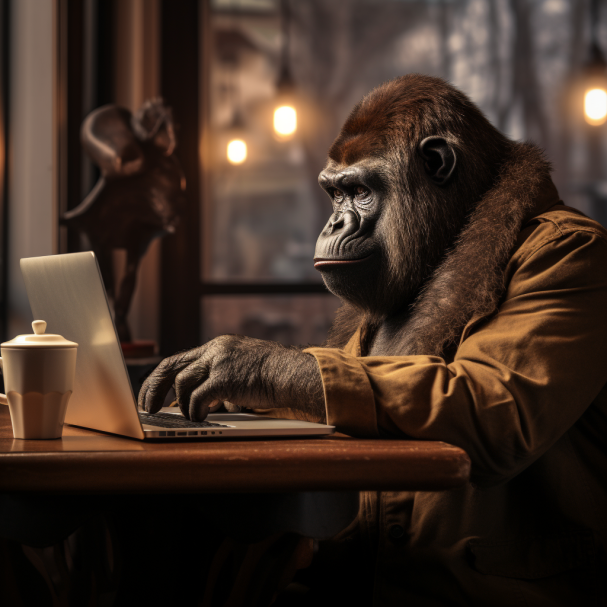
Leveraging LocalStack for S3 Client Integration Testing: A Comprehensive Guide
In the realm of cloud computing, Amazon S3 (Simple Storage Service) has established itself as a cornerstone service for storing and retrieving any amount of data at any time. However, integrating and testing applications that rely on S3 can introduce complexity, especially when ensuring that your application behaves as expected under various scenarios. This is where LocalStack comes into play. LocalStack provides a fully functional local cloud stack, allowing developers to test cloud applications offline, including services like S3, without incurring extra costs or relying on cloud connectivity.

Implementing the Saga Pattern in Go: A Practical Guide
In the world of microservices, ensuring data consistency across services without resorting to traditional, heavyweight transaction mechanisms is a common challenge. Enter the Saga Pattern: a strategy designed to manage transactions and compensations across loosely coupled services.
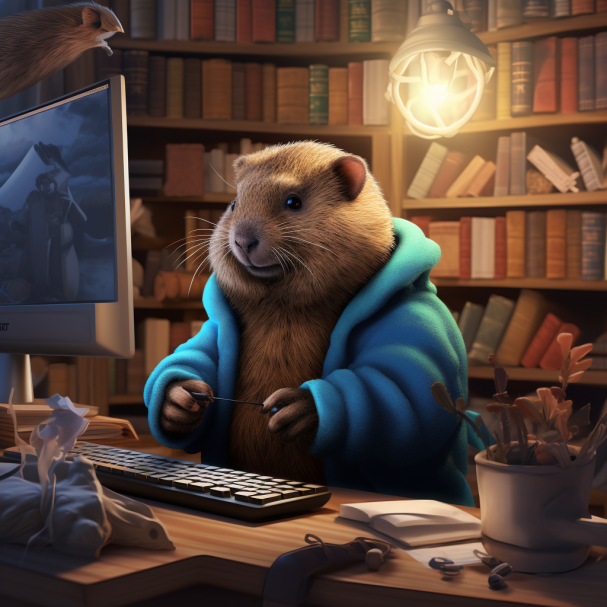
Go Fuzz Testing Explained: Enhance Your Code's Security & Reliability
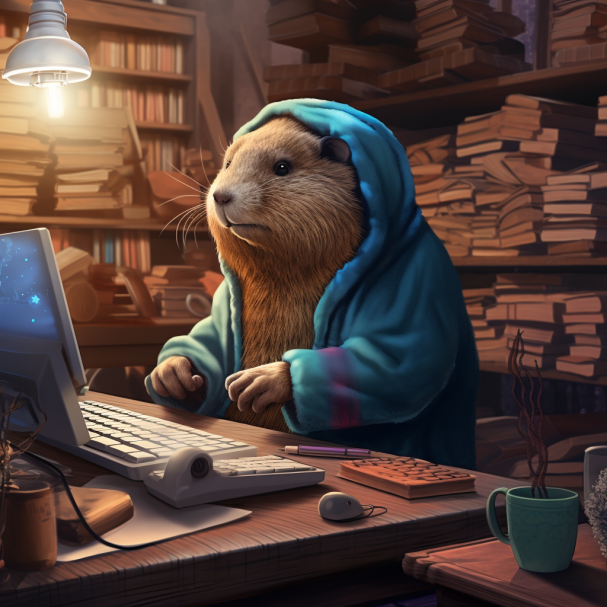
Understanding the Go Concurrency Scheduler: A Deep Dive
Concurrency is at the heart of many modern applications, especially those that require high throughput and efficiency. Go, a statically typed programming language developed by Google, has gained popularity for its simple and efficient approach to concurrency. Central to this approach is the Go concurrency scheduler, an intricate piece of the runtime that manages goroutines, Go's lightweight threads.
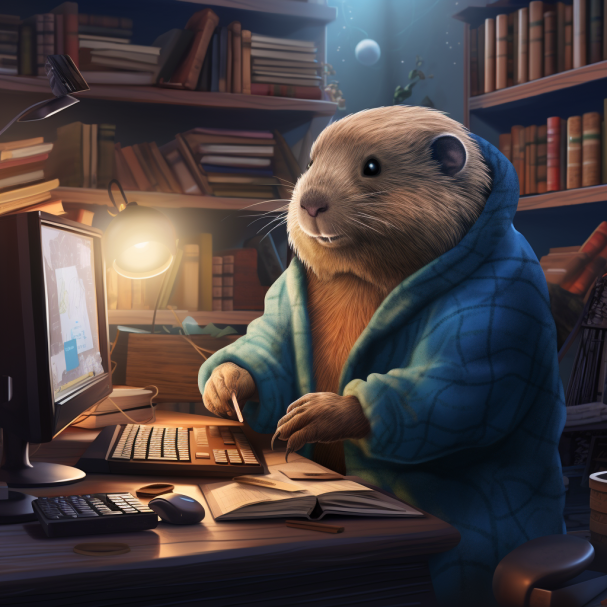
Leveraging Go's Concurrency for High-Performance Database Insertions
In the realm of software development, efficiently managing database operations is crucial for ensuring high performance and scalability. Go, with its built-in support for concurrency, offers an effective way to enhance the speed and efficiency of inserting records into a database.
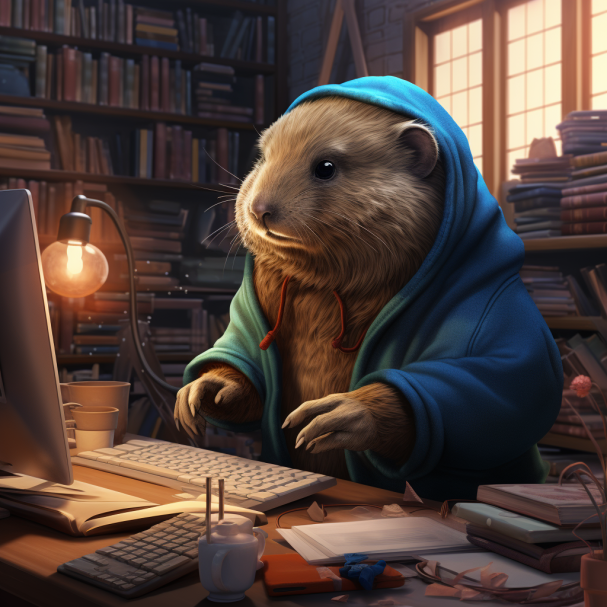
Understanding and Preventing Go Memory Leaks
In the world of software development, memory leaks can significantly impact the performance and reliability of applications. Go, also known as Golang, is celebrated for its efficiency and simplicity, especially in concurrent programming and system-level applications. However, like any other programming language, Go is not immune to memory leaks.
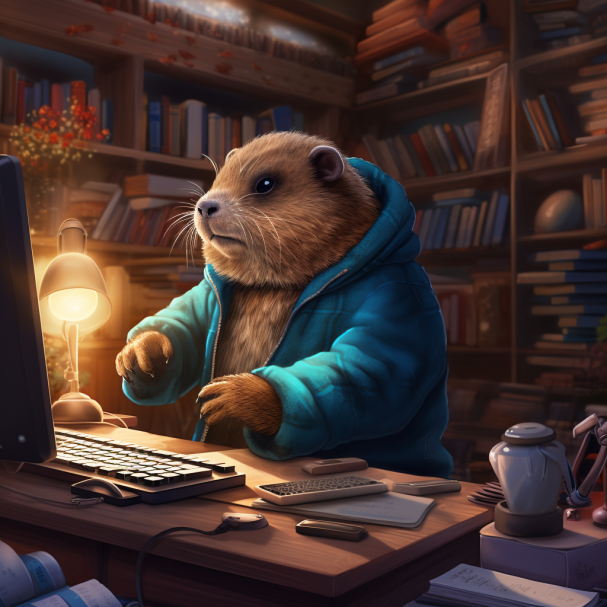
Understanding the Difference: make() vs new() in Go
In the world of Go programming, understanding the distinction between make() and new() functions is crucial for effective memory management and efficient coding practices. While both functions play pivotal roles in memory allocation, they serve different purposes and are used in different contexts.
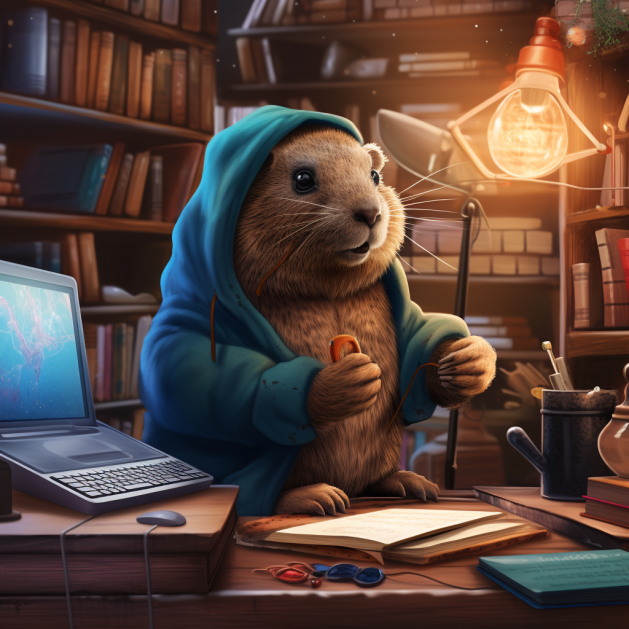
Integrating Flyway with Golang for Seamless Database Migrations
In the realm of application development, managing database schema changes elegantly across different environments can be quite challenging. This is where Flyway comes into play—a robust open-source tool that simplifies database migrations, ensuring consistency and version control of your database schema. While Flyway integrates smoothly with Java-based applications, its command-line client makes it a versatile tool for projects in any language, including Golang.