Curious About Technology
Welcome to Coding Explorations, your go-to blog for all things software engineering, DevOps, CI/CD, and technology! Whether you're an experienced developer, a curious beginner, or simply someone with a passion for the ever-evolving world of technology, this blog is your gateway to valuable insights, practical tips, and thought-provoking discussions.
Recent Posts
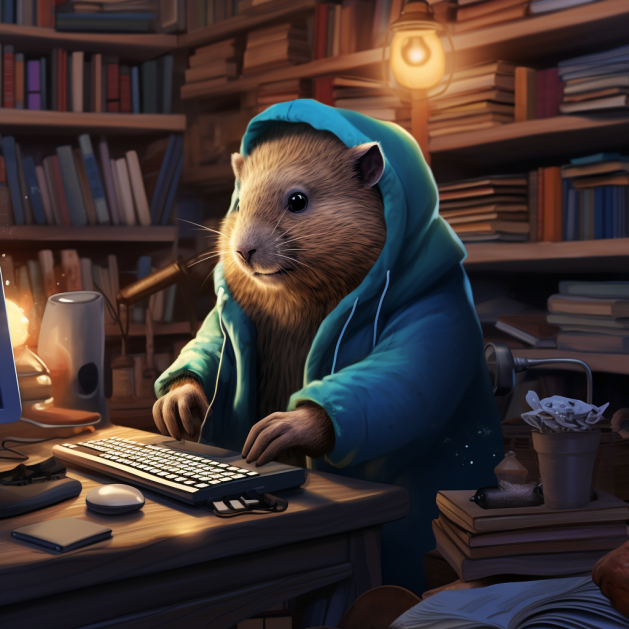
Understanding Iteration: Range vs. Index in GoLang
In GoLang, iteration over data structures is a fundamental concept that allows developers to traverse through arrays, slices, maps, and more. Two primary methods for iteration are using the range form and the traditional index-based iteration. Both approaches have their use cases, advantages, and limitations. Let's delve into these methods, understand their differences, and see when to use one over the other, with code examples to illustrate these concepts.
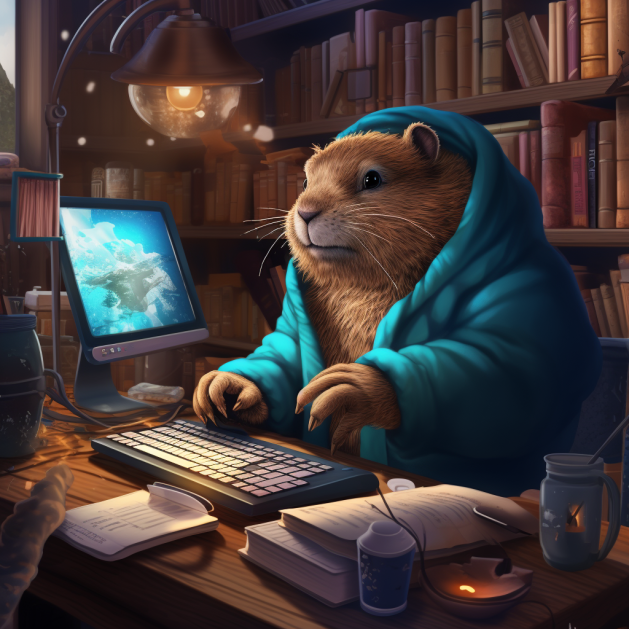
Exploring Array Techniques in Go: A Comprehensive Guide
Arrays are fundamental to any programming language, and Go is no exception. They offer a way to store and manipulate collections of data efficiently. In Go, arrays are value types, which means they are copied when assigned to a new variable or passed to functions. However, Go also provides slices, a more flexible and powerful alternative to arrays.

Mastering Nil Channels in Go: Enhance Your Concurrency Control
Using nil channels in Go can be a powerful tool for controlling the flow of concurrent operations, but it may initially seem counterintuitive. Here, we’ll explore what nil channels are, how Go treats them, and practical ways to utilize them effectively in your concurrent programming patterns.
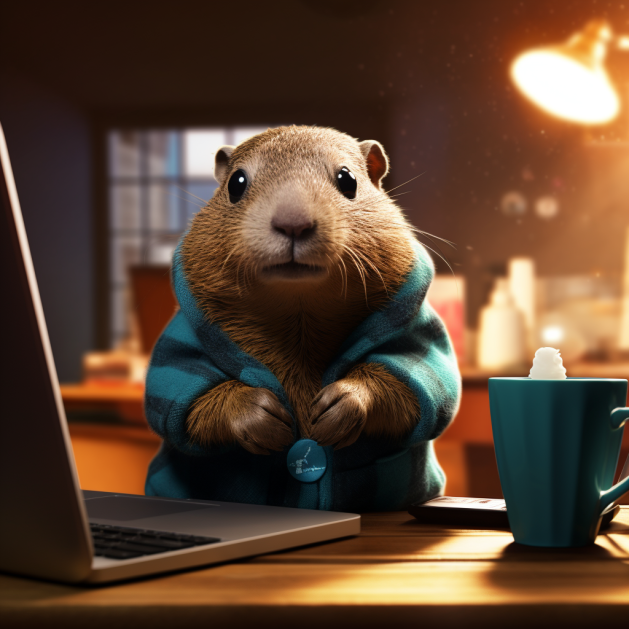
How to Effectively Unit Test Goroutines in Go: Tips & Tricks
Unit testing in Go, especially when it involves goroutines, presents unique challenges due to their concurrent nature. Goroutines are lightweight threads managed by the Go runtime, and they are used to perform tasks concurrently.

Understanding the Difference Between make and new in Go
In the Go programming language, memory allocation and initialization are crucial concepts that allow developers to manage data structures efficiently. Two built-in functions that play a significant role in this context are make and new. Although they might seem similar at first glance, they serve different purposes and are used in different scenarios.
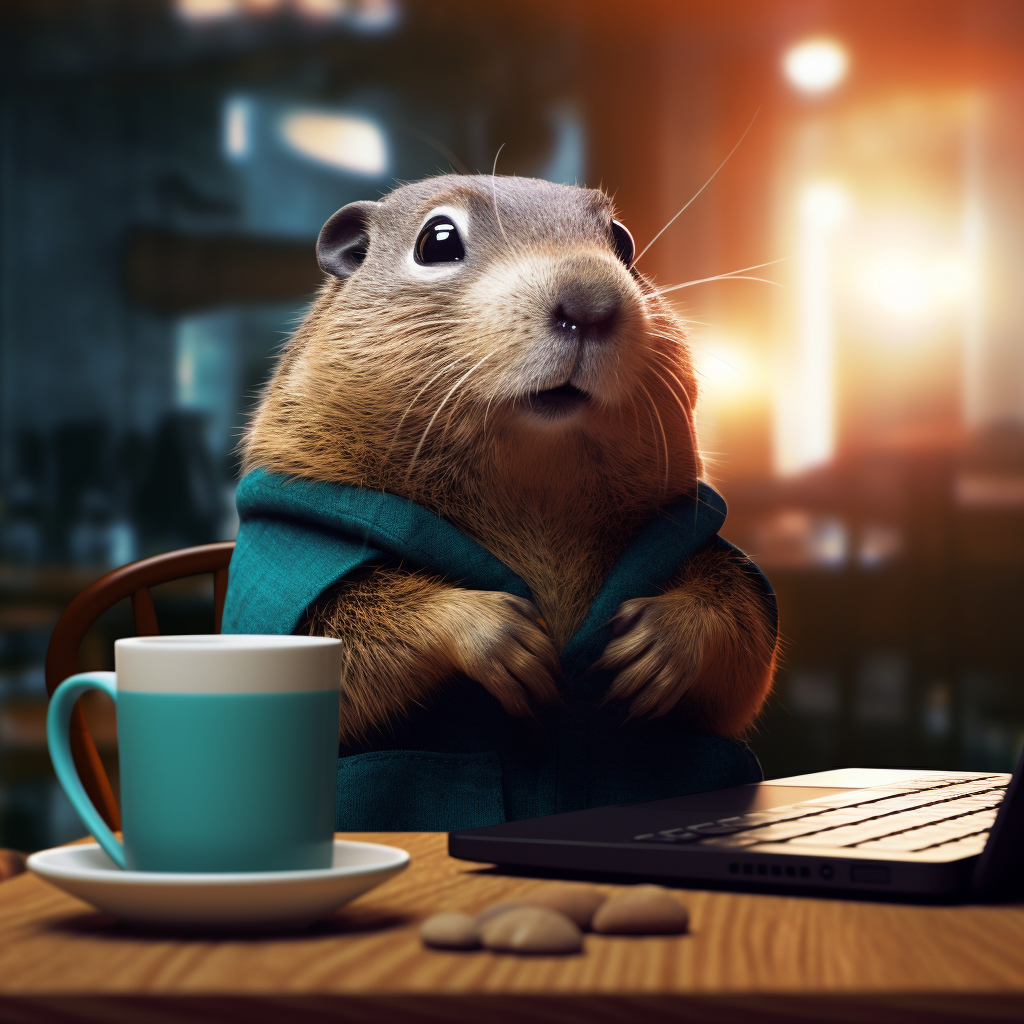
Understanding Type Aliases in Go: A Comprehensive Guide
Go is a statically typed programming language that has gained popularity for its simplicity, efficiency, and strong support for concurrent programming. One of the features that contribute to its simplicity and flexibility is the concept of type aliases.

Understanding Variable Passing into Go Routines
Go is a statically typed, compiled programming language designed for simplicity and efficiency, with a particular emphasis on concurrent programming. One of the core features of Go's concurrency model is goroutines, which are functions capable of running concurrently with other functions. A common question among Go developers, especially those new to the language, is why and how to pass variables into a goroutine.
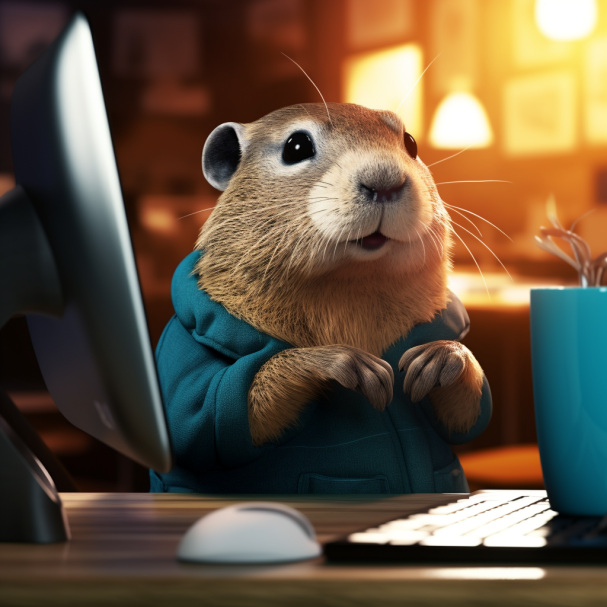
Using Go for Data Science: A Fresh Perspective
In the ever-evolving landscape of data science, Python has long been the reigning champion, largely due to its simplicity and the vast array of libraries available. However, the tide is slowly turning, and other languages are making their mark in the data science realm. One such contender is Go. Go is gaining popularity for its efficiency, performance, and ease of use.
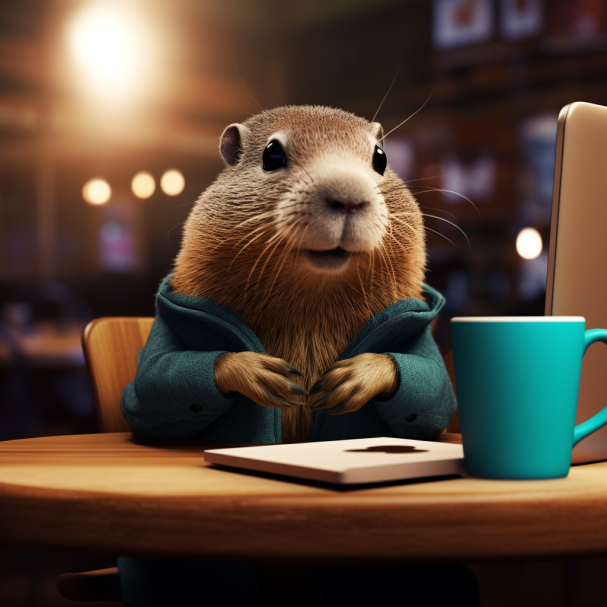
Understanding Go's Garbage Collection: A Deep Dive
Go is a statically typed, compiled programming language. Among its many features, Go's garbage collection mechanism stands out as a critical component for memory management.
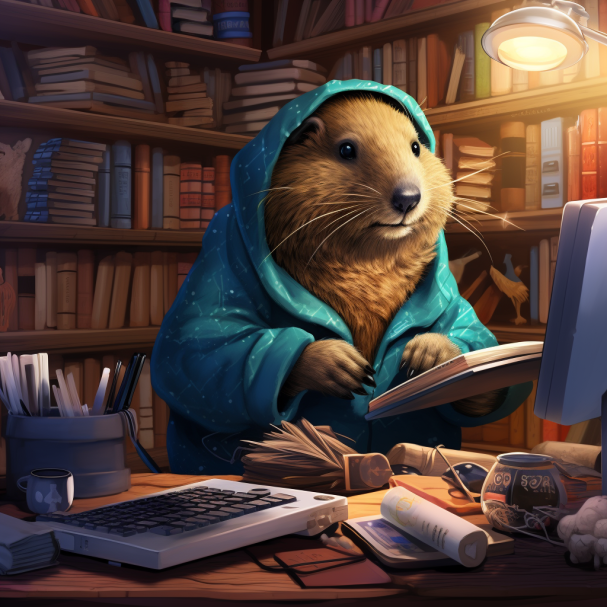
Efficient Data Management in Go: The Power of Struct Tags
In the world of programming, efficiency and organization are paramount. Go, a statically typed language developed by Google, provides a unique feature for structuring and managing data effectively: struct tags. These tags offer an elegant way to add metadata to the structure fields, making data serialization and validation more streamlined and robust.
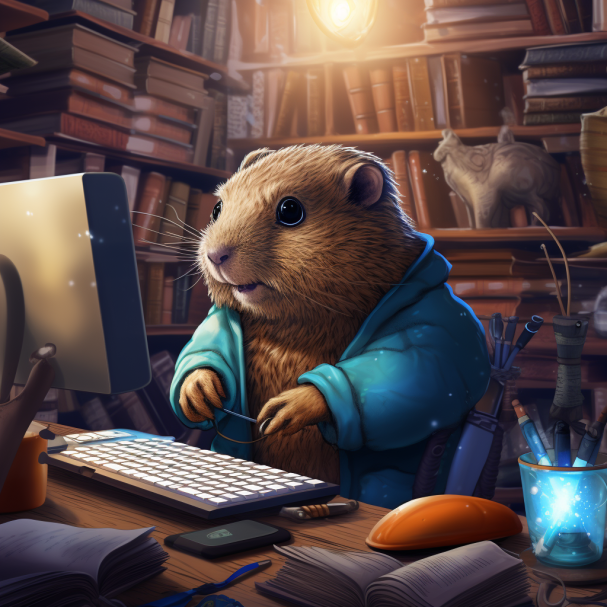
Mastering SQLMock for Effective Database Testing
Testing is a crucial aspect of software development, ensuring that applications perform as expected. For applications that interact with databases, mocking the database interactions is essential for isolated and efficient testing. SQLMock is a powerful tool in the Go ecosystem, designed to facilitate testing of database interactions without relying on a real database.
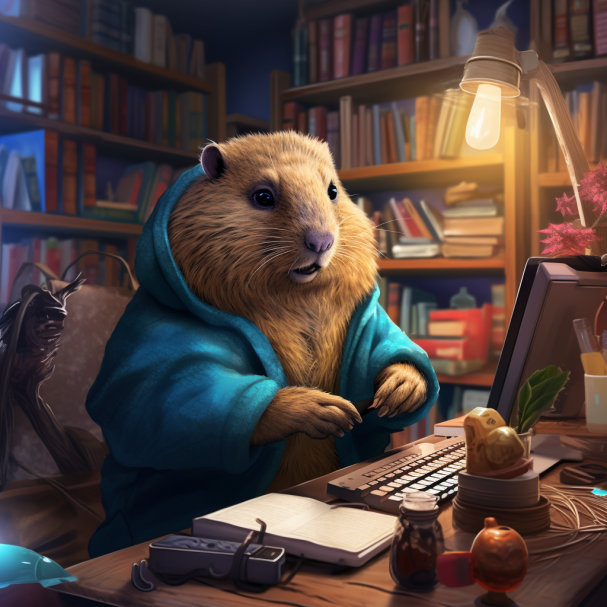
Mastering Performance Testing in Go with Vegeta
In the realm of software development, particularly in an era where web services and APIs reign supreme, ensuring that your application can handle high traffic and deliver consistent performance is paramount. For developers working in Go, a language renowned for its efficiency and concurrency capabilities, Vegeta emerges as a powerful tool for performance testing.

Mastering the Open/Closed Principle in Go Programming
The Open/Closed Principle (OCP) is a fundamental concept in object-oriented programming, and it holds a vital place among the five SOLID principles. Initially formulated by Bertrand Meyer, this principle plays a pivotal role in enhancing the flexibility and maintainability of software applications.
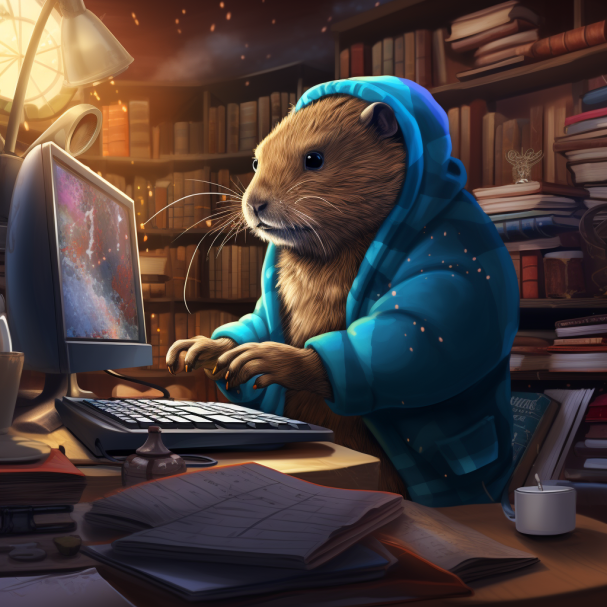
Advanced Implementation of Hexagonal Architecture in Go
In the realm of software design, Hexagonal Architecture, also known as Ports and Adapters Architecture, stands as a beacon for creating flexible, maintainable, and scalable applications. Its application in Go, a language celebrated for its straightforwardness and performance, can significantly enhance the adaptability and testability of software projects.
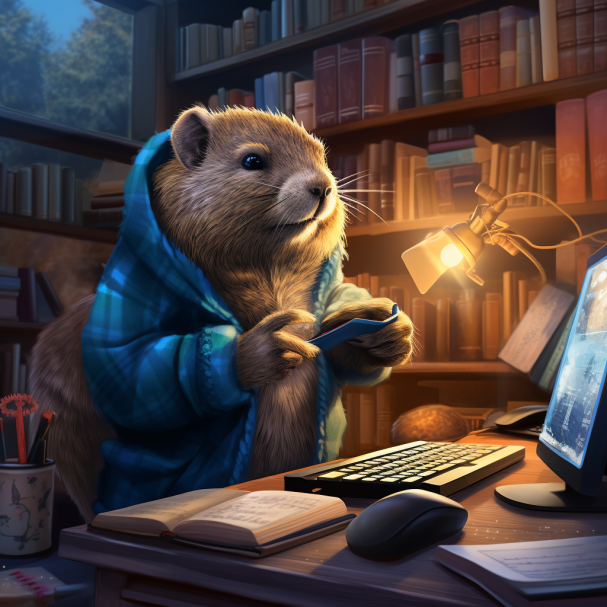
Managing Files in a Go API Folder Structure: Best Practices for Organizing Your Project
Creating a robust and efficient folder structure is crucial for any API project, and this is particularly true when working with Go, a language known for its simplicity and efficiency.
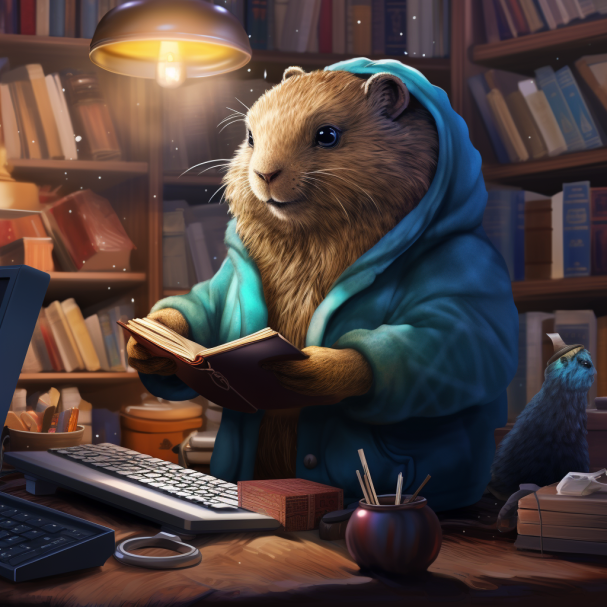
Building TLS Communications in a Go Application
Transport Layer Security (TLS) is an essential protocol for securing internet communications. As Go continues to gain popularity for building high-performance and scalable applications, implementing TLS in Go applications is becoming increasingly important.
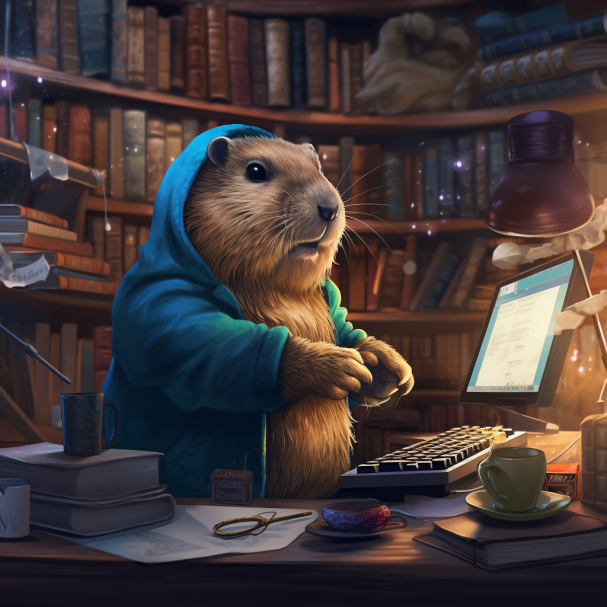
Go vs. Python for Parsing HTML: A Comparative Analysis
In the realm of web scraping and HTML parsing, choosing the right programming language and libraries is crucial for efficiency, ease of use, and reliability. Two popular choices in this domain are Go, using its standard libraries, and Python with its BeautifulSoup library.
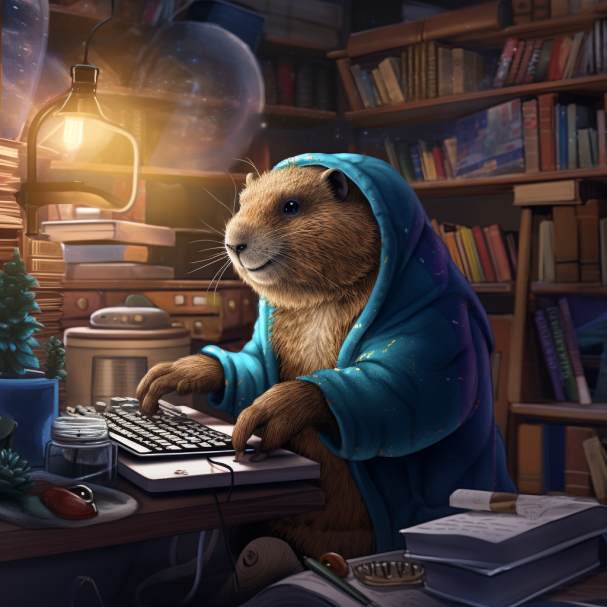
Understanding and Using the Empty Interface in Go
Go is a statically typed programming language known for its simplicity and efficiency. One of its unique features is the interface{} type, commonly known as the empty interface.

Enhancing Go Code Quality with Go-Critic: A Developer's Guide
As a Go developer, striving for high-quality, efficient, and maintainable code is always a priority. Go-Critic, an advanced linter for the Go programming language, serves as a crucial tool in achieving these goals.

Exploring Uber's FX: A Game Changer for Go Developers
In the dynamic world of software engineering, advancements and innovations are a constant. One such notable development is Uber's FX, an innovative framework created for the Go programming language.