Curious About Technology
Welcome to Coding Explorations, your go-to blog for all things software engineering, DevOps, CI/CD, and technology! Whether you're an experienced developer, a curious beginner, or simply someone with a passion for the ever-evolving world of technology, this blog is your gateway to valuable insights, practical tips, and thought-provoking discussions.
Recent Posts
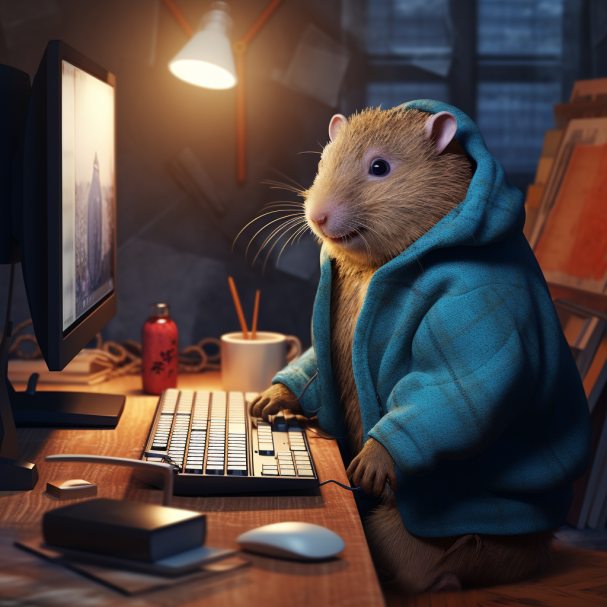
Understanding Singleflight in Go: A Solution for Eliminating Redundant Work
As developers, we often encounter situations where multiple requests are made for the same resource simultaneously. This can lead to redundant work, increased load on services, and overall inefficiency. In the Go programming language, the singleflight package provides a powerful solution to this problem. In this post, we'll explore what singleflight is, how it works, and how you can use it to optimize your Go applications.
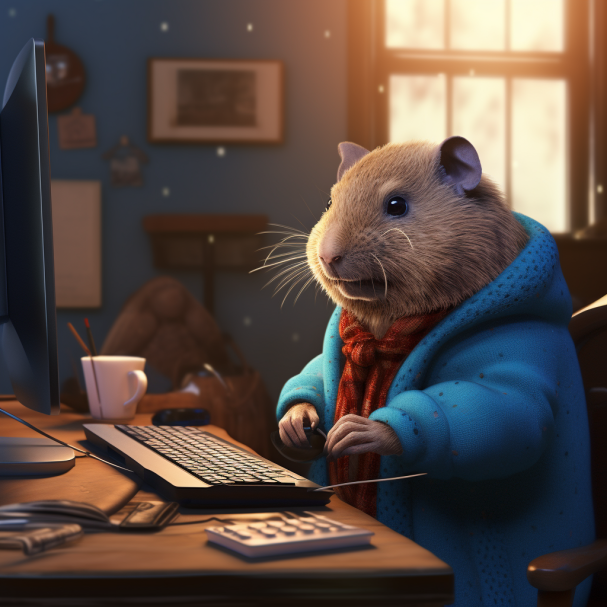
Mastering Go Templates: A Guide with Practical Examples
Go is a statically typed, compiled programming language designed by Google. It's known for its simplicity, efficiency, and robustness. Among its many features, Go's template package (text/template and html/template) is a powerful tool for generating textual output (like HTML) with dynamic data.
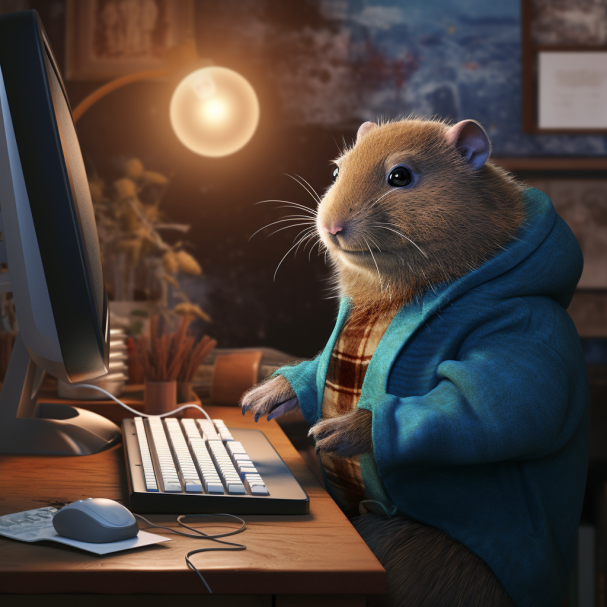
Understanding Rate Limiting in Go: A Comprehensive Guide
Rate limiting is a critical component in API design, ensuring that your services remain reliable and secure. In Go, there are several algorithms to implement rate limiting, each with its own set of advantages and challenges. In this post, we'll explore these algorithms, their pros and cons, and provide code examples to help you integrate them into your Go APIs.
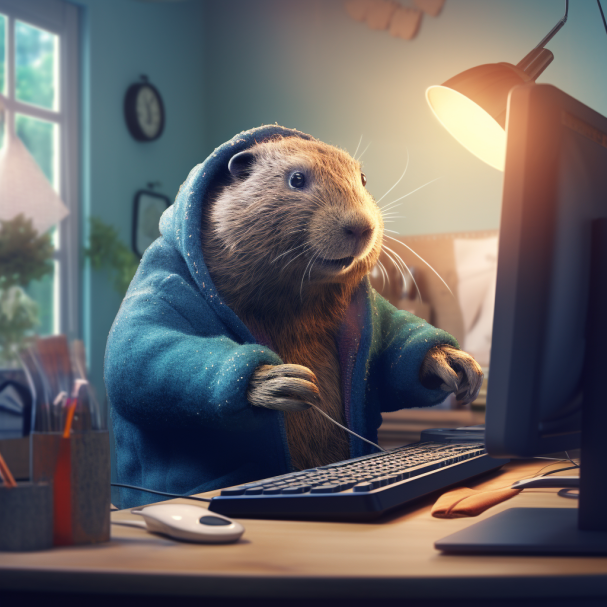
Mastering the select Statement in Go with Channels: A Comprehensive Guide
Go is a powerful programming language known for its simplicity and efficiency, particularly in the realms of concurrency and parallelism. One of the key features that makes Go stand out in handling concurrent operations is its use of channels and the select statement.
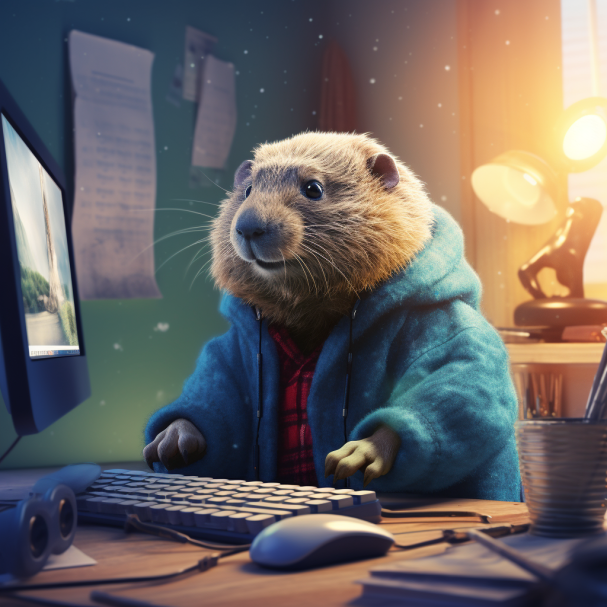
Writing Loops in Go: A Comprehensive Guide
Go is a statically typed, compiled programming language designed at Google. It is known for its simplicity, robustness, and efficient concurrency handling. One of the fundamental concepts in any programming language, including Go, is the loop - a control flow statement that allows code to be executed repeatedly based on a given Boolean condition. In this post, we'll dive into how to write loops in Go.

Mastering Concurrency in Go with errgroup: Simplifying Goroutine Management
Concurrency is a cornerstone of Go's design, granting it the power to handle multiple tasks simultaneously. However, managing concurrent operations can be complex, especially when dealing with errors and synchronization. Enter the errgroup package, a hidden gem in Go's ecosystem that simplifies handling goroutines, especially when they share a common error state.
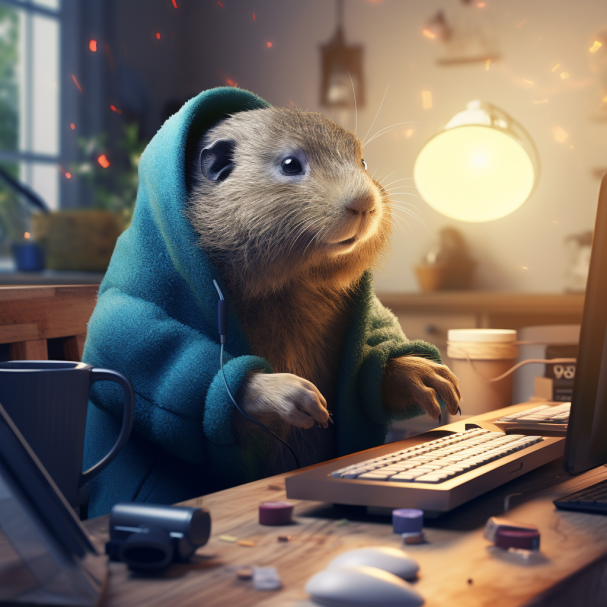
Data Sharding in Golang: Optimizing Performance and Scalability
In the world of software development, managing large volumes of data efficiently is a common challenge. This is particularly true for applications that require high performance and scalability. One effective strategy to address this challenge is data sharding.

Understanding UDP and TCP in Go: A Comprehensive Guide
In the world of networking, two primary protocols dominate communication: User Datagram Protocol (UDP) and Transmission Control Protocol (TCP). These protocols are fundamental for network communication, each with its unique characteristics and use cases.

Streaming Upload Solution in Golang: Building an Efficient API
Streaming uploads are crucial for handling large files or data streams efficiently in web applications. In this blog post, we'll explore how to implement a streaming upload solution using Go, a powerful language known for its efficiency and concurrency support.
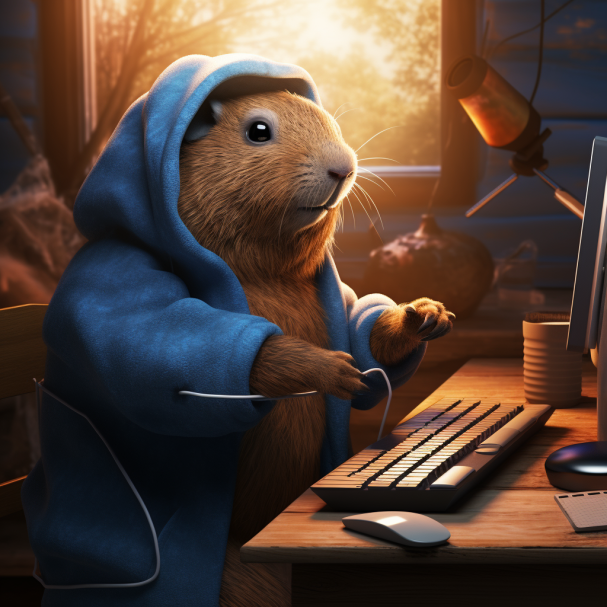
Understanding and Using the "go work" Command in Go Programming
In the evolving landscape of Go programming, the go work command has emerged as a valuable tool for developers. Introduced in recent versions of Go, this command streamlines the process of working with multiple modules in a more coherent and efficient manner.

Writing WebAssembly in Go: A Guide for Expert Software Engineers
WebAssembly (often abbreviated as Wasm) has emerged as a groundbreaking technology, enabling developers to run code written in multiple languages at near-native speed on the web. Go, with its simplicity and efficiency, has become a popular choice for writing WebAssembly.

Creating an Effective Bloom Filter in Go: Enhancing Data Management Efficiency
In the realm of data structures, the Bloom filter stands out for its efficiency in space and time, especially when dealing with large data sets. This blog post delves into the concept of Bloom filters and illustrates their implementation in the Go programming language, a choice renowned for its simplicity and performance.

Enhancing Go Struct Efficiency: Essential Tips for Memory Optimization
Writing efficient Go structs is a vital skill for developers working with the Go programming language. In this blog post, we'll explore how to structure your Go structs efficiently, focusing particularly on the importance of field ordering and its impact on memory usage.
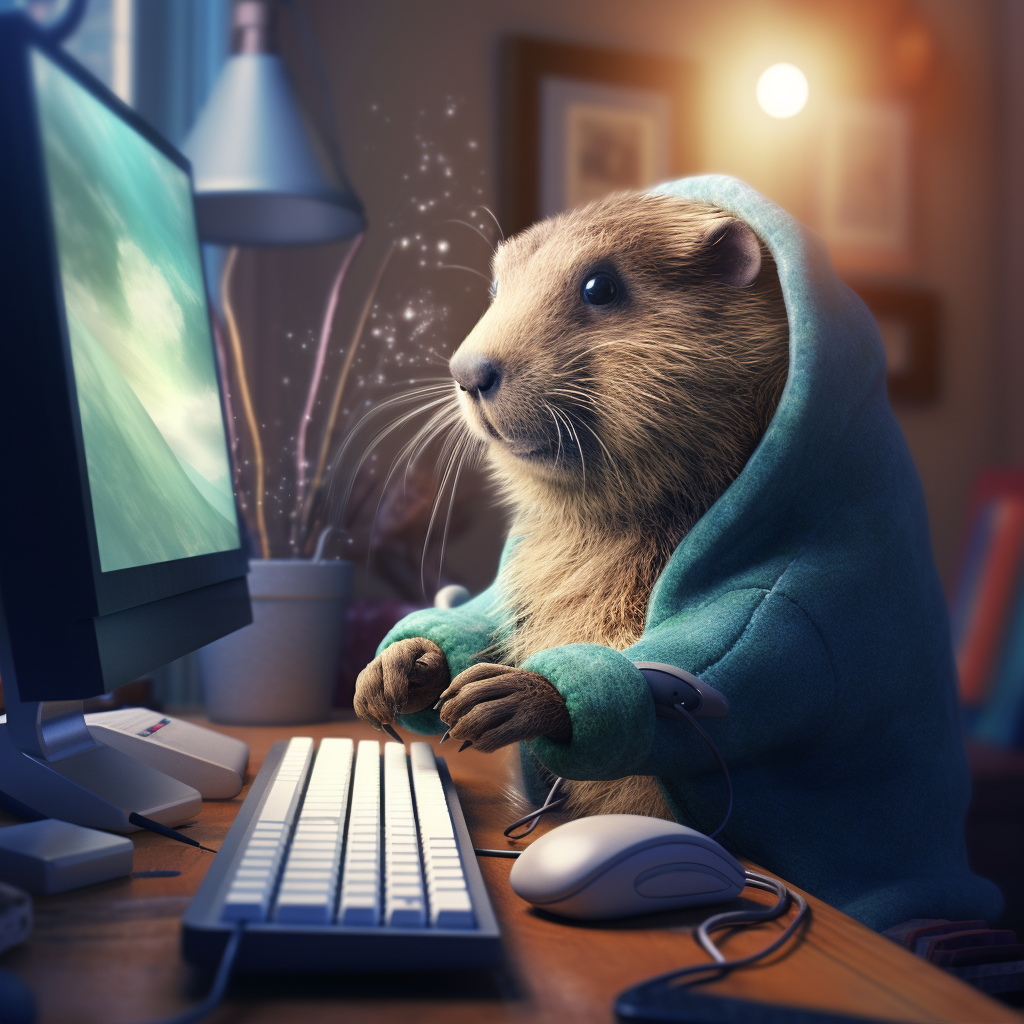
Designing an Effective Go Repository for Microservices
In the ever-evolving landscape of software engineering, microservices have become a pivotal architectural style, particularly in cloud-native applications. Go has emerged as a popular choice for developing these services due to its simplicity and high performance.

The Circuit Breaker Pattern: Enhancing System Resilience
In the realm of software engineering, system resilience is paramount. As systems grow in complexity, the potential for failure multiplies, making robust error handling and failure management essential. This is where the Circuit Breaker pattern, a design pattern first popularized by Michael Nygard in his book "Release It!", comes into play.
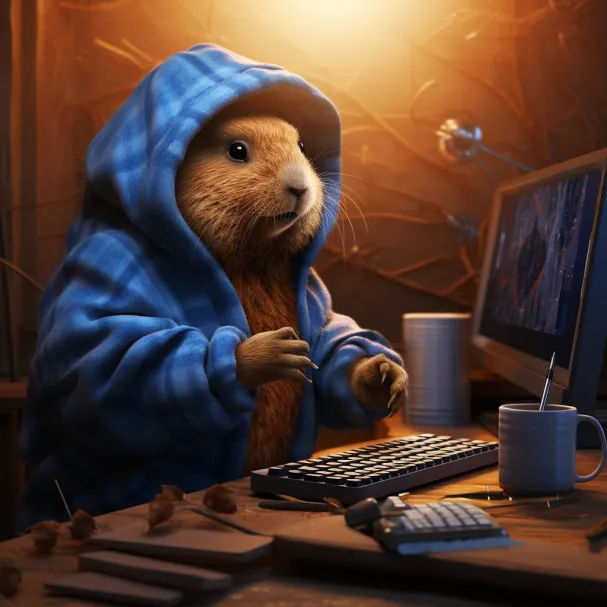
Mastering QLX in Go: A Comprehensive Guide
Welcome to our deep dive into using QLX with the Go programming language! Go, known for its efficiency and simplicity, has become a go-to choice for modern software development. In this guide, we'll explore how QLX, an advanced library/tool, enhances Go's capabilities, making database operations more seamless and efficient.
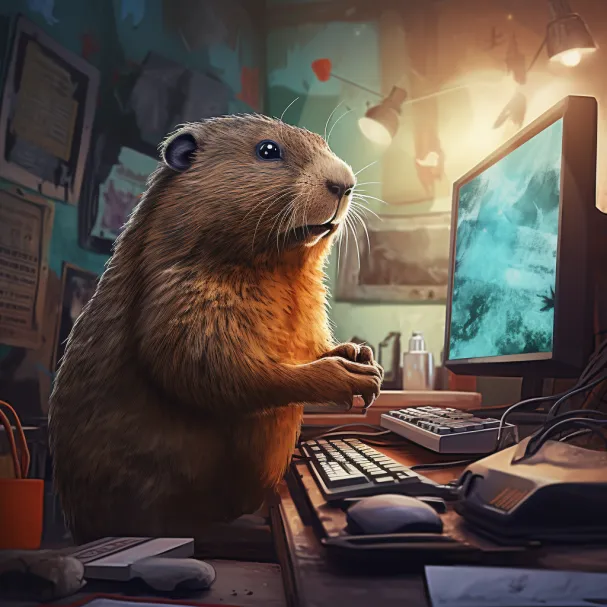
Mastering API Development with Go-Swagger: A Comprehensive Guide for Software Engineers
In the rapidly evolving world of technology, API development stands as a cornerstone of modern software engineering. Among the tools at a developer's disposal, Go-Swagger emerges as a powerful ally. This post will delve into the depths of Go-Swagger, guiding you through its efficient utilization in building robust APIs.
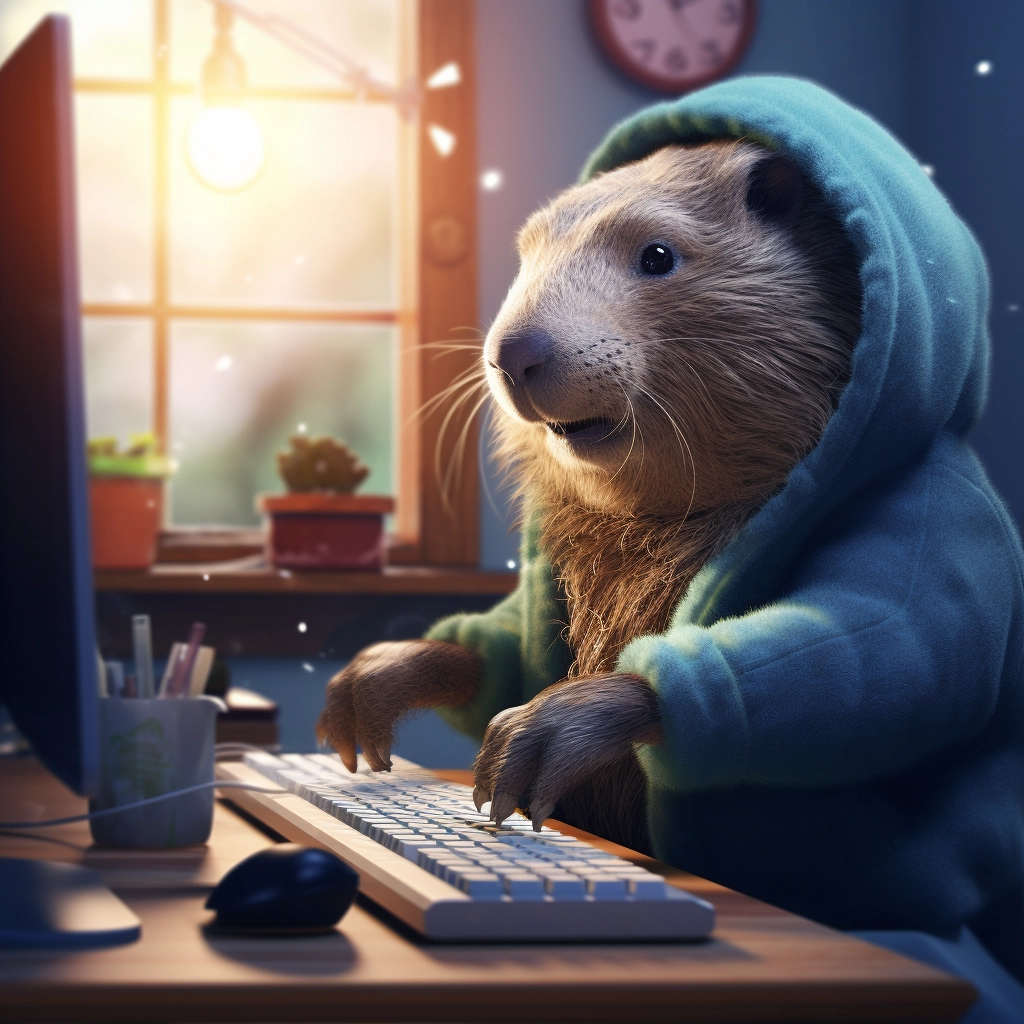
Mastering CLI Development with Cobra in Go: A Comprehensive Guide
In this post, we'll explore how to create powerful CLI tools using the Cobra library in the Go programming language. Whether you're new to Go or looking to expand your toolkit, this guide offers insights into building efficient CLI applications.
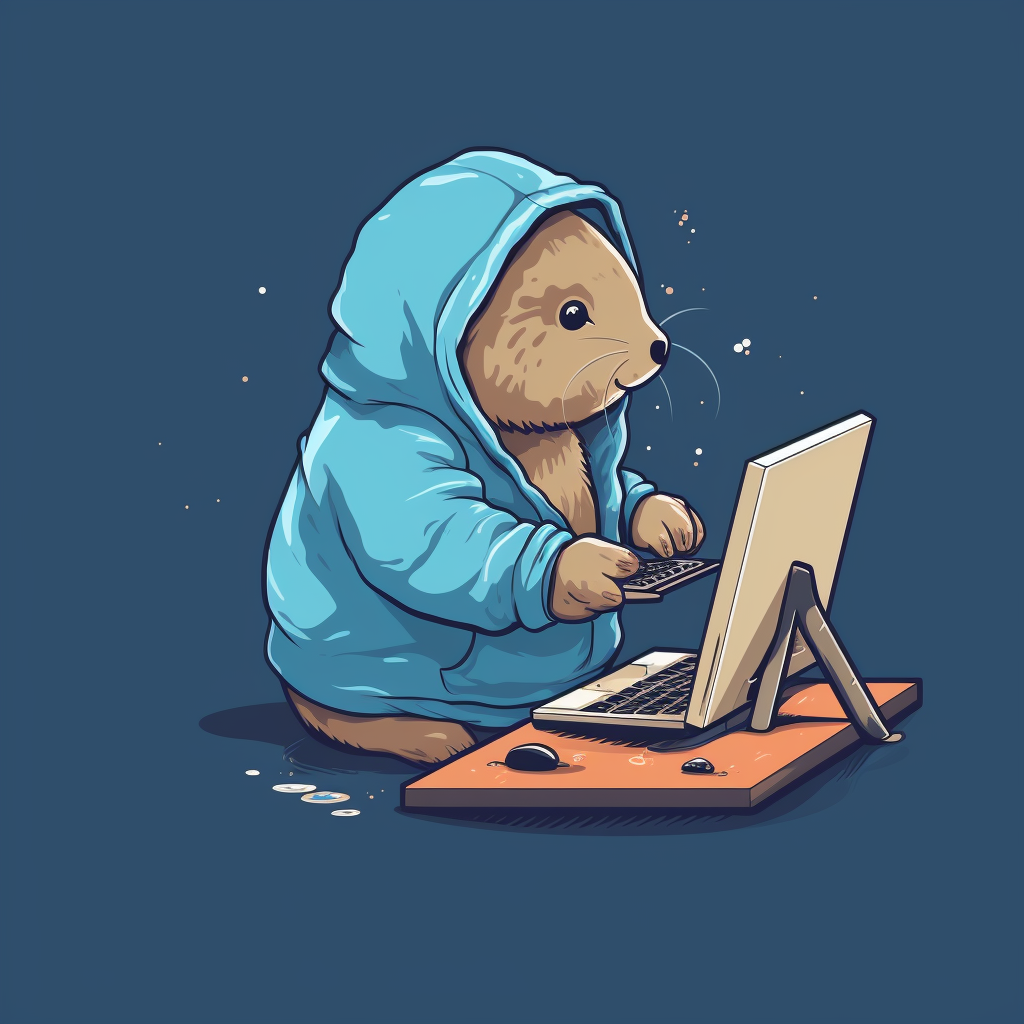
Design Pattern Series: Demystifying the Bridge Design Pattern in Go
Design patterns are essential tools in a programmer's toolbox. They provide solutions to common software design problems and promote code reusability, maintainability, and scalability. One such design pattern is the Bridge pattern, which separates an object's abstraction from its implementation.
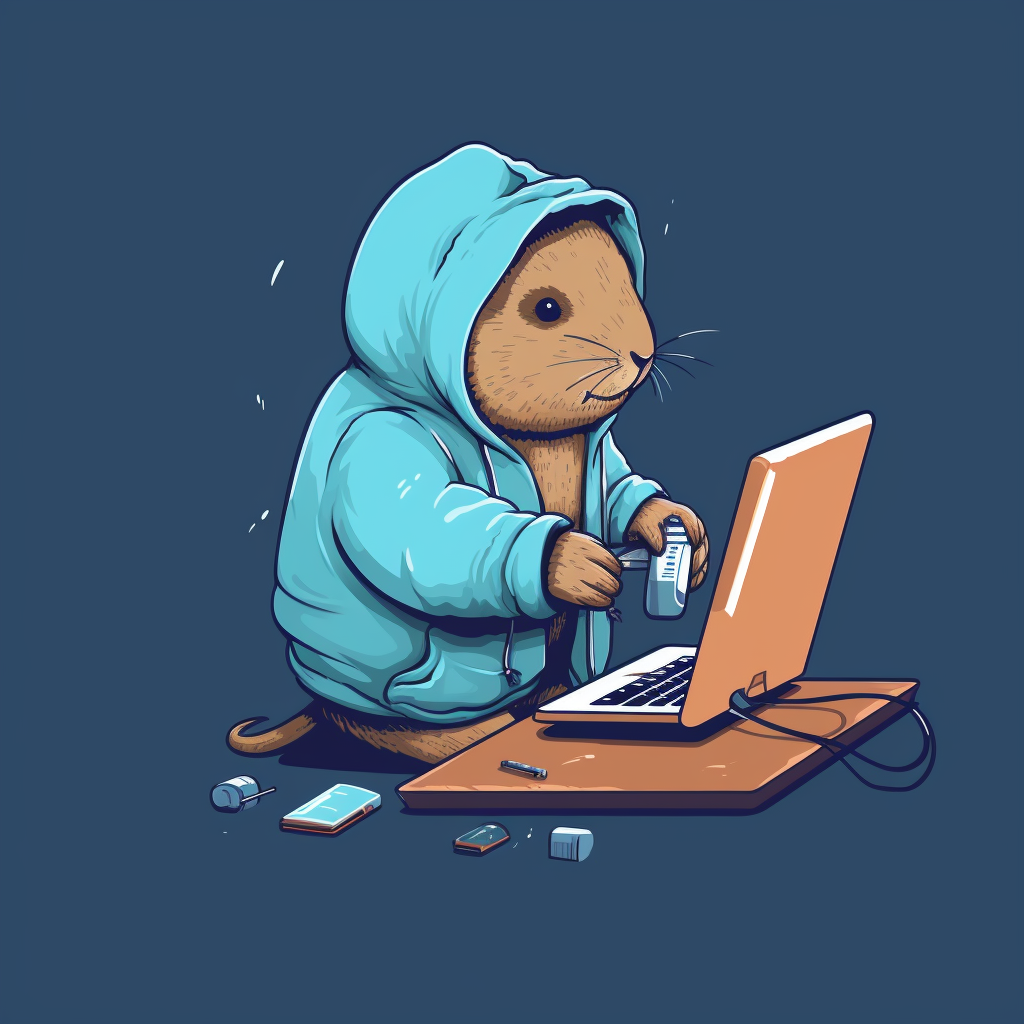
Design Pattern Series: Understanding the Facade Design Pattern in Go
Design patterns are essential tools in the software developer's toolkit, helping us solve common problems in elegant and maintainable ways. One such pattern is the Facade design pattern.